Kotlin if-else expression
Last Updated :
09 Jun, 2022
Decision Making in programming is similar to decision-making in real life. In programming too, a certain block of code needs to be executed when some condition is fulfilled. A programming language uses control statements to control the flow of execution of a program based on certain conditions. If the condition is true then it enters into the conditional block and executes the instructions.
There are different types of if-else expressions in Kotlin:
- if expression
- if-else expression
- if-else-if ladder expression
- nested if expression
if statement:
It is used to specify whether a block of statements will be executed or not, i.e. if a condition is true, then only the statement or block of statements will be executed otherwise it fails to execute.
Syntax:
if(condition) {
// code to run if condition is true
}
Flowchart:
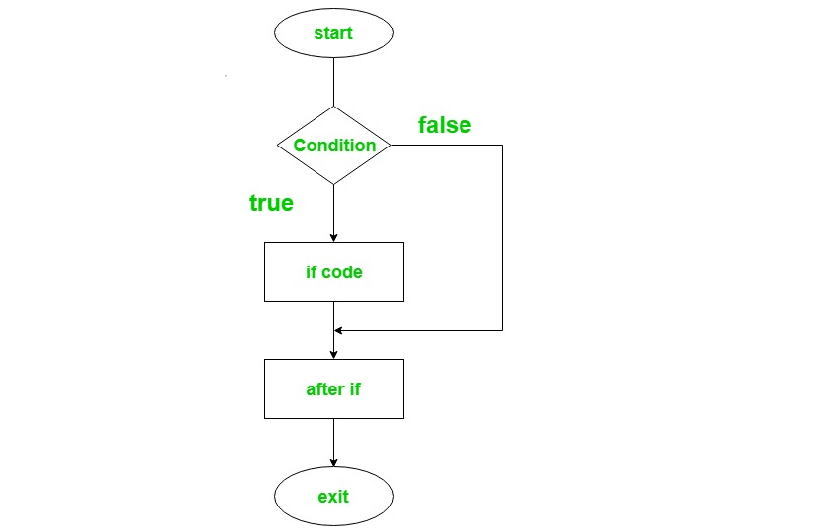
Example:
Java
fun main(args: Array<String>) {
var a = 3
if (a > 0 ){
print( "Yes,number is positive" )
}
}
|
Output:
Yes, number is positive
if-else statement:
if-else statement contains two blocks of statements. ‘if’ statement is used to execute the block of code when the condition becomes true and ‘else’ statement is used to execute a block of code when the condition becomes false.
Syntax:
if(condition) {
// code to run if condition is true
}
else {
// code to run if condition is false
}
Flowchart:
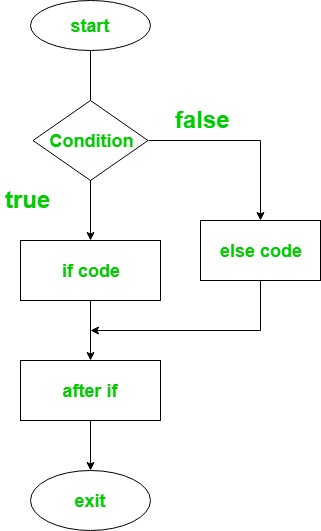
Here is the Kotlin program to find the larger value of two numbers.
Example:
Java
fun main(args: Array<String>) {
var a = 5
var b = 10
if (a > b){
print( "Number 5 is larger than 10" )
}
else {
println( "Number 10 is larger than 5" )
}
}
|
Output:
Number 10 is larger than 5
Kotlin if-else expression as ternary operator:
In Kotlin, if-else can be used as an expression because it returns a value. Unlike java, there is no ternary operator in Kotlin because if-else returns the value according to the condition and works exactly similar to ternary.
Below is the Kotlin program to find the greater value between two numbers using if-else expression.
Java
fun main(args: Array<String>) {
var a = 50
var b = 40
var max = if (a > b){
print( "Greater number is: " )
a
}
else {
print( "Greater number is:" )
b
}
print(max)
}
|
Output:
Greater number is: 50
if-else-if ladder expression:
Here, a user can put multiple conditions. All the ‘if’ statements are executed from the top to bottom. One by one all the conditions are checked and if any of the conditions is found to be true then the code associated with the if statement will be executed and all other statements bypassed to the end of the block. If none of the conditions is true, then by default the final else statement will be executed.
Syntax:
if(Firstcondition) {
// code to run if condition is true
}
else if(Secondcondition) {
// code to run if condition is true
}
else{
}
Flowchart:
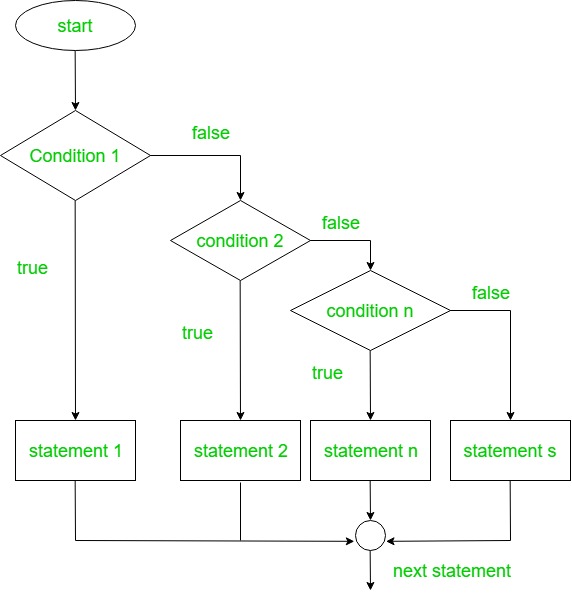
Below is the Kotlin program to determine whether the number is positive, negative, or equal to zero.
Java
import java.util.Scanner
fun main(args: Array<String>) {
val reader = Scanner(System.`in`)
print( "Enter any number: " )
var num = reader.nextInt()
var result = if ( num > 0 ){
"$num is positive number"
}
else if ( num < 0 ){
"$num is negative number"
}
else {
"$num is equal to zero"
}
println(result)
}
|
Output:
Enter any number: 12
12 is positive number
Enter any number: -11
-11 is negative number
Enter any number: 0
0 is zero
nested if expression:
Nested if statements mean an if statement inside another if statement. If the first condition is true then code the associated block to be executed, and again check for the if condition nested in the first block and if it is also true then execute the code associated with it. It will go on until the last condition is true.
Syntax:
if(condition1){
// code 1
if(condition2){
// code2
}
}
Flowchart:
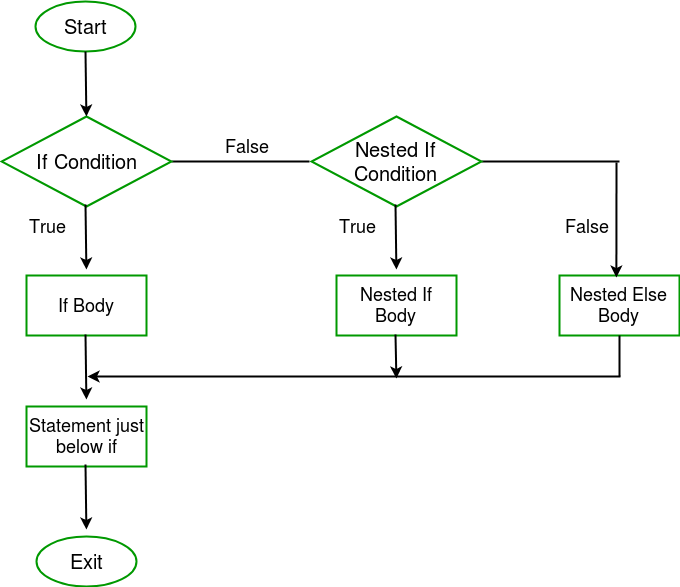
Below is the Kotlin program to determine the largest value among the three Integers.
Java
import java.util.Scanner
fun main(args: Array<String>) {
val reader = Scanner(System.`in`)
print( "Enter three numbers: " )
var num1 = reader.nextInt()
var num2 = reader.nextInt()
var num3 = reader.nextInt()
var max = if ( num1 > num2) {
if (num1 > num3) {
"$num1 is the largest number"
}
else {
"$num3 is the largest number"
}
}
else if ( num2 > num3){
"$num2 is the largest number"
}
else {
"$num3 is the largest number"
}
println(max)
}
|
Output:
Enter three numbers: 123 231 321
321 is the largest number
Share your thoughts in the comments
Please Login to comment...