jQuery | Get Content and Attributes
Last Updated :
22 Feb, 2019
Get Content: In order to get the content of DOM object, there are three simple methods.jQuery methods for DOM manipulation which are listed below:
- text(): It is used to set or return the text content of selected elements.
- html(): It is used to set or return the content of selected elements including HTML markup.
- val(): It is used to set or return the value of form fields.
Example: This example uses text content method to get the content.
<!DOCTYPE html>
< html >
< head >
< title >jQuery Get Content</ title >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 id = "GFG1" style = "color:green;" >
GeeksForGeeks
</ h1 >
< h2 id = "GFG2" >jQuery Get Content</ h2 >
< button id = "btn1" >Text</ button >
< button id = "btn2" >HTML</ button >
< script >
$(document).ready(function(){
$("#btn1").click(function(){
alert("Text: " + $("#GFG2").text());
});
$("#btn2").click(function(){
alert("HTML: " + $("#GFG1").html());
});
});
</ script >
</ body >
</ html >
|
Output:
- Before click on the button:
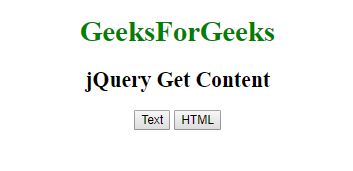
- After click on the Text button:
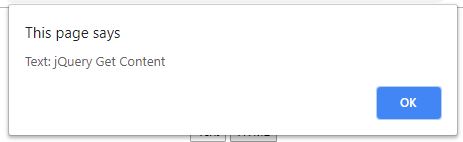
- After click on the Html button:
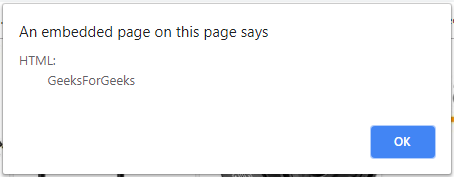
Get Attributes The jQuery attr() method is used to get attribute values of DOM objects.
Example: This example uses attr() method to get the attribute value.
<!DOCTYPE html>
< html >
< head >
< title >jQuery Get Attributes</ title >
< script src =
</ script >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
GeeksForGeeks
</ h1 >
< h2 >jQuery Get Attributes</ h2 >
< button id = "btn1" >Click</ button >
< br >< br >
< h3 >
geeksforgeeks.org
</ a >
</ h3 >
< script >
$(document).ready(function(){
$("button").click(function(){
alert($("#GFG").attr("href"));
});
});
</ script >
</ body >
</ html >
|
Output:
- Before click on the button:
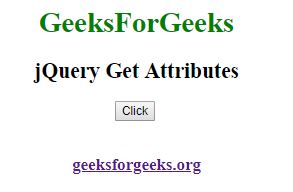
- After click on the button:
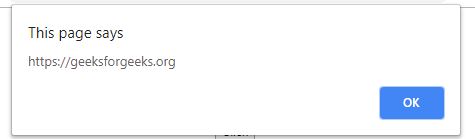
Share your thoughts in the comments
Please Login to comment...