Java Program to Print Mirror Upper Star Triangle Pattern
Last Updated :
17 Mar, 2023
The pattern has two parts – both mirror reflections of each other. The base of the triangle has to be at the bottom of the imaginary mirror and the tip at the top.
Illustration:
Input:
size = 7
Output:
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
* * * * * * *
* * * * * *
* * * * *
* * * *
* * *
* *
*
Approach:
Divide pattern into sub-patterns
- Divide this into two parts
- Both parts are a mirror reflection of each other. And each part comprises 2 triangles. Therefore, In total, we have to print 4 triangles to get the desired pattern.
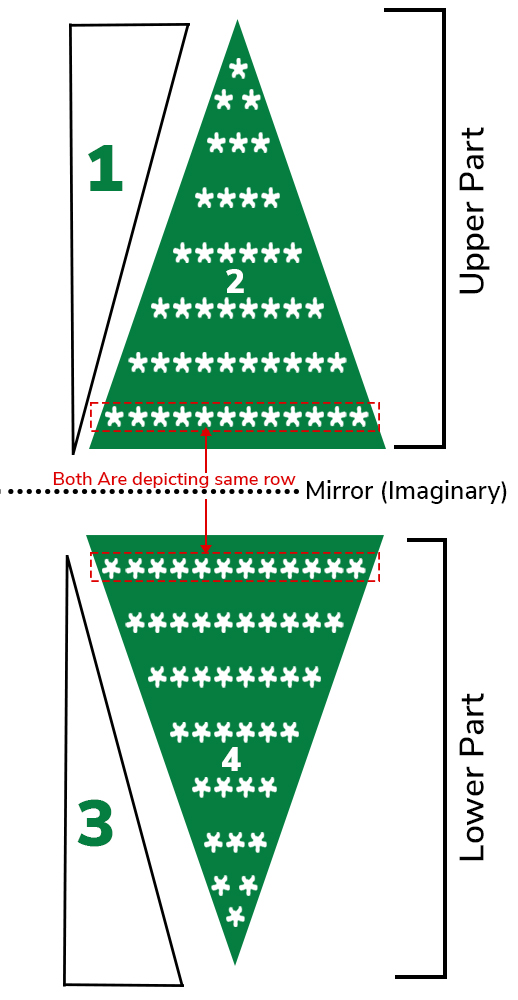
Example 1: Upper Part
Java
import java.io.*;
class GFG {
private static void displayUpperPart( int size)
{
int m, n;
for (m = size - 1 ; m >= 0 ; m--) {
for (n = 0 ; n < m; n++) {
System.out.print( " " );
}
for (n = m; n <= size - 1 ; n++) {
System.out.print( "*"
+ " " );
}
System.out.println();
}
}
public static void main(String[] args)
{
int size = 7 ;
displayUpperPart(size);
}
}
|
Output
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
Example 2: Lower Part of the triangle
Java
import java.io.*;
class GFG {
private static void displayLowerPart( int size)
{
int m, n;
for (m = 1 ; m <= size; m++) {
for (n = 1 ; n < m; n++) {
System.out.print( " " );
}
for (n = m; n <= size; n++) {
System.out.print( "*"
+ " " );
}
System.out.println();
}
}
public static void main(String[] args)
{
int size = 7 ;
displayLowerPart(size);
}
}
|
Output
* * * * * * *
* * * * * *
* * * * *
* * * *
* * *
* *
*
Example 3: Complete Mirror Upper Star Triangle Pattern
Java
import java.io.*;
public class GFG {
private static void displayUpperPart( int size)
{
int m, n;
for (m = size - 1 ; m >= 0 ; m--) {
for (n = 0 ; n < m; n++) {
System.out.print( " " );
}
for (n = m; n <= size - 1 ; n++) {
System.out.print( "*"
+ " " );
}
System.out.println();
}
}
private static void displayLowerPart( int size)
{
int m, n;
for (m = 1 ; m <= size; m++) {
for (n = 1 ; n < m; n++) {
System.out.print( " " );
}
for (n = m; n <= size; n++) {
System.out.print( "*"
+ " " );
}
System.out.println();
}
}
public static void main(String[] args)
{
int size = 7 ;
displayUpperPart(size);
displayLowerPart(size);
}
}
|
Output
*
* *
* * *
* * * *
* * * * *
* * * * * *
* * * * * * *
* * * * * * *
* * * * * *
* * * * *
* * * *
* * *
* *
*
Time complexity: O(n2) where n is given input size
Auxiliary Space : O(1)
Share your thoughts in the comments
Please Login to comment...