The Java Collection framework is a fundamental part of the Java programming language, It covers a major part of Java and acts as a prerequisite for many Advanced Java Topics. However, programmers often find it difficult to find a platform to Practice Java Online.
Take a look at our Java Collection Exercise that contains Java Collection Practice Problems to practice and to develop your Java Programming skills. Our Java Collection Practice Exercise will cover all the topics from Basic to Advanced, from ArrayList to Priority Queue. So, let’s dive in and sharpen our Java Collection skills together!
What is Collection in Java?
Collection Framework in Java contains a Set of Interfaces and Classes to represent groups of objects as a Single Unit. In Java, the Collection interface (java.util.Collection) and Map interface (java.util.Map) are the two main “root” interfaces of Java collection classes.
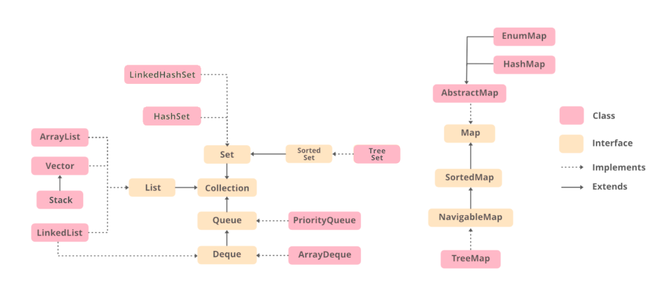
Basic Program
Want to solidify your Java collection skills? Here’s your chance! Get hands-on with these basic practice programs in Java Collection. Work with common collections like ArrayLists and HashSets, all while strengthening your Java coding abilities.
1. How to Print a Collection in Java?
Java// Java program to demonstrate how to
// Print a Collection
import java.util.*;
// Driver Class
class GFG {
// Main Function
public static void main(String[] args)
{
String[] geeks
= { "Rahul", "Utkarsh", "Shubham", "Neelam" };
List<String> al = new ArrayList<String>();
// adding elements of array to arrayList.
Collections.addAll(al, geeks);
System.out.println(al);
}
}
Output[Rahul, Utkarsh, Shubham, Neelam]
2. How to Make a Collection Read-Only in Java?
Java// Java Program to make
// Set Interface Object Read-Only
import java.util.Set;
import java.util.HashSet;
import java.util.Collections;
// Driver Class
class GFG {
// Main Function
public static void main(String[] args)
{
// Set of Integer
Set<Integer> numbers = new HashSet<Integer>();
// Set have 1 to 10 numbers
for (int i = 1; i <= 5; i++) {
numbers.add(i);
}
// print the integers
numbers.stream().forEach(System.out::print);
// Removing element from the list
numbers.remove(5);
System.out.println("\nAfter Performing Operation");
numbers.stream().forEach(System.out::print);
System.out.println(
"\nSet is also By Default Readable and Writable");
// Now making Read-Only Set
// Using unmodifiableSet() method.
try {
numbers = Collections.unmodifiableSet(numbers);
// This line will generate an Exception
numbers.remove(4);
}
catch (UnsupportedOperationException
unsupportedOperationException) {
System.out.println(
"Exceptions is "
+ unsupportedOperationException);
}
finally {
System.out.println(numbers.contains(3));
System.out.println("Now Set is Read-Only");
}
}
}
Output12345
After Performing Operation
1234
Set is also By Default Readable and Writable
Exceptions is java.lang.UnsupportedOperationException
true
Now Set is Read-Only
3. Java Program to Compare Elements in a Collection.
Input : List = [3, 5, 18, 4, 6]
Output: Min value of our list : 3
Max value of our list : 18
Explanation: Comparison lead to result that the min of the list is 3 and max of list is 18. Similarly we can test for other Collections too.
4. Java Program to Remove a Specific Element From a Collection
Input: ArrayList : [10, 20, 30, 1, 2]
Index: 1
Output: ArrayList : [10, 30, 1, 2]
Explanation: Removed element from index 1.
5. How to Get a Size of Collection in Java?
Input: Linked_List: [geeks, for, geeks]
Output: 3
Explanation: As there are only 3 elements in the Collection So the value returned is 3.
ArrayList Problems
ArrayList is a Collection which is the implementation of Dynamic Array Data Structure.

ArrayList
6. How to Add an Element in Java ArrayList?
Input: Number of Elements to be Added: 3
Elements to be Added : "A" , "B" , "C"
Output: list=[ A, B, C ]
7. How to remove an element from ArrayList in Java?
Input: ArrayList = [10, 20, 30, 1, 2] index=1
Output: [10, 30, 1, 2]
8. How to Replace an Element in Java ArrayList?
Input: ArrayList = [A, B, C, D]
index = 2
element =' E '
Output: [ A, B, E, D ]
9. Java Program to Convert Array to ArrayList.
Input: arr = [ 2, 3, 4, 5, 8]
Output: ArrayList = [ 2, 3, 4, 5, 8]
10. Comparing two ArrayList In Java
Input : ArrayList1 = [1, 2, 3, 4],
ArrayList2 = [4, 2, 3, 1]
Output: Array List are not equal
LinkedList Problems
LinkedList Class is an implementation of the LinkedList data structure which is a linear data structure where the elements are not stored in contiguous locations and every element is a separate object with a data part and address part.

Linked List in Java
11. How to Iterate LinkedList in Java?
Java// Java program for iterating the LinkedList
// using Iterator
import java.util.Iterator;
// Importing LinkedList class from
// java.util package
import java.util.LinkedList;
public class GFG {
public static void main(String[] args) {
// Creating a LinkedList of Integer Type
LinkedList<Integer> linkedList = new LinkedList<>();
// Inserting some Integer values to our LinkedList
linkedList.add(15);
linkedList.add(10);
linkedList.add(1);
linkedList.add(40);
linkedList.add(71);
// Calling the function to iterate our LinkedList
iterateUsingIterator(linkedList);
}
// Function to iterate the Linked List using Iterator
public static void iterateUsingIterator(LinkedList<Integer> linkedList){
System.out.print("Iterating the LinkedList using Iterator : ");
// Creating an Iterator to our current LinkedList
Iterator it = linkedList.iterator();
// Inside the while loop we check if the next element
// exists or not if the next element exists then we print
// the next element and move to it otherwise we come out
// of the loop
// hasNext() method return boolean value
// It returns true when the next element
// exists otherwise returns false
while(it.hasNext()){
// next() return the next element in the iteration
System.out.print(it.next() + " ");
}
}
}
OutputIterating the LinkedList using Iterator : 15 10 1 40 71
12. How to Perform List to Set Conversion in Java?
Input: List : {1,2,3,4}
Output: Set : {1,2,3,4}
13. Java Program to Convert ArrayList to LinkedList.
Input: ArrayList : {1,2,3,4}
Output: LinkedList : {1,2,3,4}
14. Java Program to Convert Array to LinkedList in Java.
Input: Array : {1,2,3,4}
Output: LinkedList : {1,2,3,4}
HashMap Problems
HashMap is the Class in Java Collection which is implemented based on Map Data Structure. It works on Key value pairs where we can store data in pairs , and can access based on Key values in Java.
.png)
HashMap in Java
15. How to Iterate HashMap in Java?
Input: { "A" = "Angular" , "P": "Python" , "J" = "Java" , "H" = "Hibernate" }
Output: P = Python
A = Angular
H = Hibernate
J = Java
16. How to Check if a Key exists in a HashMap in Java?
Input: HashMap: { 1=Geeks, 2=ForGeeks, 3=GeeksForGeeks }, key = 2
Output: True
Input: HashMap: { 1=G, 2=e, 3=e, 4=k, 5=s }, key = 10
Output: False
17. Java Program to Find the Occurrence of Words in a String using HashMap.
Input: "Alice is girl and Bob is boy"
Output: { Bob=1 , Alice=1 , and=1 , is=2 , girl=1 , boy=1 }
18. How to Copy One HashMap to Another HashMap in Java?
Input: HashMap1 = { A=1 , B=2 , C=3 }
Output: HashMap2 = { A=1 , B=2 , C=3 }
19. Java Program to Print all Duplicate characters in a String.
Input: str = "geeksforgeeks"
Output: s : 2
e : 4
g : 2
k : 2
20. Java Program to Convert HashMap to TreeMap.
Input: HashMap: {1=Geeks, 2=forGeeks, 3=A computer Portal}
Output: TreeMap: {1=Geeks, 2=forGeeks, 3=A computer Portal}
HashSet Problems
Challenge your understanding of Java HashSets! This section dives into practical problems that test your ability to leverage the unique properties of HashSets. Solve these problems and solidify your grasp of this essential Java collection!
21. Initializing HashSet in Java
22. How to Sort HashSet in Java.
Input: HashSet: [Geeks, For, ForGeeks, GeeksforGeeks]
Output: [For, ForGeeks, Geeks, GeeksforGeeks]
23. Convert Array to HashSet in Java.
Input : Array: [1, 2, 3, 4, 5, 6]
Output: Set: [1, 2, 3, 4, 5, 6]
24. How to Print HashSet Elements in Java?
Input: Set: { 5 , 2 , 3 , 6 , null };
Output: null
2
3
5
6
Explanation: Elements of Set are not stored in the order they are inserted.
Stack Problems
Stack is part of Collection in Java, it is a Interface which is based on Stack Data Structure. It follows the principle of LIFO that is Last in First Out.
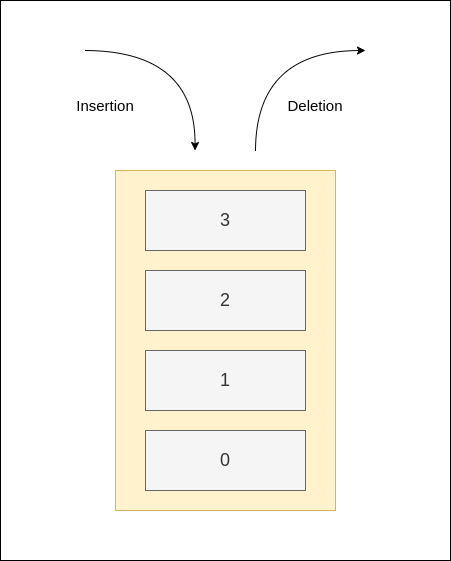
Stack in Java
25. How to Add Elements in Stack Collection in Java?
Input: stack = [10, 20, 30, 40, 50]
ele = 100
pos = 2
Output: [10 , 20 , 100 , 30 , 40 , 50]
Explanation: Add element (ele) in the stack at the position (pos).
26. Calculate weight of parenthesis based on the given conditions.
Input: S = “(()(()))”
Output: 6
Explanation:
() = 1 , then (()) =2
()(()) = 1+2 = 3
(()(())) = 2*3 = 6
To check the rules refer to the article mentioned below.
27. Check If it is Possible to Convert Binary String into Unary String
Choose two adjacent characters, such that both are 1’s or 0’s. Then invert them, Formally, if both are 0’s then into 1’s or vice-versa.
If we are able to convert every element either to all 1 or all 0 then the answer will be Yes else No.
Input: N = 6, S = “110110”
Output: YES
Explanation: The operations are performed as:
Invert 0,1 -> S = 000110
Invert 4,5 -> S = 000000
Queue Problems
Queue is the part of Java Collection, it is an Interface based on Queue Data Structure. It follows the Principle of FIFO that is First In First Out.
-660.png)
Queue in Java
28. How to Iterate over a Queue in Java?
Below is the Solution of the question:
Java// Java Program demonstrating
// Queue Iteration
import java.util.Iterator;
import java.util.LinkedList;
import java.util.Queue;
// Driver Class
public class Gfg {
// Main function
public static void main(String[] args) {
// Iterating Queue
Queue<Integer> studentQueue = new LinkedList<>();
// Inserting element in Queue
studentQueue.add(1);
studentQueue.add(3);
studentQueue.add(8);
studentQueue.add(14);
studentQueue.add(9);
studentQueue.add(3);
// Initialising Iterator
Iterator<Integer> studentQueueIterator = studentQueue.iterator();
// Iterating Queue
while (studentQueueIterator.hasNext()) {
Integer name = studentQueueIterator.next();
System.out.print(name+" ");
}
}
}
29. How to Add Elements in Queue Collection in Java?
30. How to Implement a Circular Queue in Java?
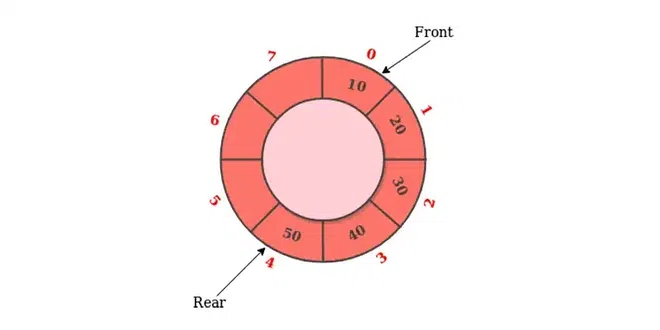
Circular Queue
31. How to Convert a List to a Queue in Java?
Input: List = { A, B, C}
Output: Queue = { A, B, C }
Priority Queue Problems
Got the hang of Java collections? Time to test your skills! This section throws some priority queue problems your way. Practice putting elements in order, taking them out strategically, and mastering this awesome data structure in a fun way.
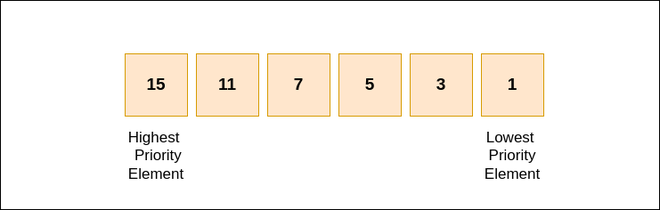
Priority Queue in Java
32. How to implement Min Heap in Java?
Input: 5 , 1 , 10 , 30 , 20
Output: 1 , 5, 10 , 20 , 30
Explanation: Min Heap is a type of Data Structure in which internal node is smaller than or equal to its children.
33. How to Configure Java Priority Queue to Handle Duplicate Elements?
PriorityQueue doesn't handle duplicate elements in a specific way. But we can configure PriorityQueue to add add duplicate elements in it.
34. How to Check if a PriorityQueue is Empty or Contains Elements in Java?
Input: { 6 , 4 , 1 , 55 }
Output: Not Empty
35. How to Implement PriorityQueue through Comparator in Java?
Implementation of a PriorityQueue Using a Custom Comparator to Define the Priority of Elements in the Queue.
36. Java Program to Sort Items By Weight
Input: items = [“Laptop”, “TV”, “Phone”, “Watch”]
weights = [500, 1000,250, 50]
Output: [“TV”, “Laptop”, “Phone”, “Watch”]
Explanation: Here the items are sorted based on their weight in decreasing order
Conclusion
You’ve completed these Java collection exercises. Now you can handle ArrayLists, HashSets, Priority Queues, and more with confidence. Ready to push your skills further? Revisit these exercises, experiment with your own ideas, and solidify your Java collection mastery!
Share your thoughts in the comments
Please Login to comment...