Interpolation Search in Python
Last Updated :
13 May, 2024
Interpolation Search is an efficient searching algorithm particularly when dealing with the uniformly distributed sorted arrays. It improves upon binary search by the estimating the location of the target element based on its value and the range of the elements in the array. For example, if the target value is closer to the first element, interpolation search is likely to start search from the staring side.
What is Interpolation Search:
Interpolation Search is a searching algorithm that finds the target value in the sorted array by narrowing down the search space. The Interpolation Search is an improvement over Binary Search when the values in a sorted array are uniformly distributed. Unlike Binary Search in which always divides the array into the two halves interpolation search calculates the probable position of the target element within the array.
How Interpolation Search Works:
Interpolation search assumes that the array is uniformly distributed and sorted in the ascending order. It takes advantage of this assumption to the estimate the position of the target element. Instead of always dividing the array into the two halves like in binary search interpolation search calculates the probable position of the target element using the interpolation formula:
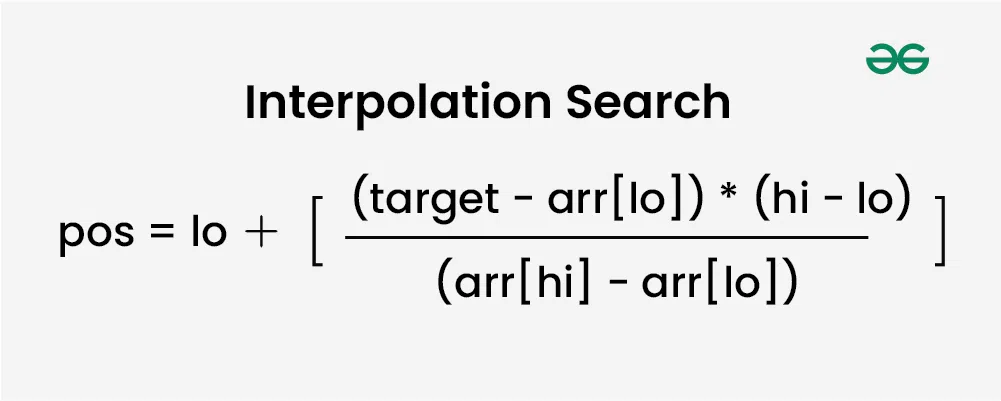
The idea of formula is to return higher value of pos when element to be searched is closer to arr[hi] and smaller value when closer to arr[lo]
pos = lo + ((target – arr[lo]) * (hi – lo)) / (arr[hi] – arr[lo])
arr[]: Array where elements need to be searched
target: Element to be searched
lo: Starting index in arr[]
hi: Ending index in arr[]
After estimating the position, interpolation search compares the target value with value at the calculated position. Based on this comparison it adjusts the search range accordingly.
The process repeats until the target element is found or until the search range becomes empty.
Implementation of Interpolation Search in Python:
Below is the implementation of Interpolation Search in Python
Python
# Python3 program to implement
# interpolation search
# with recursion
# If x is present in arr[0..n-1], then
# returns index of it, else returns -1.
def interpolationSearch(arr, lo, hi, x):
# Since array is sorted, an element present
# in array must be in range defined by corner
if (lo <= hi and x >= arr[lo] and x <= arr[hi]):
# Probing the position with keeping
# uniform distribution in mind.
pos = lo + (((hi - lo) * (x - arr[lo])) // (arr[hi] - arr[lo]))
# Condition of target found
if arr[pos] == x:
return pos
# If x is larger, x is in right subarray
if arr[pos] < x:
return interpolationSearch(arr, pos + 1,
hi, x)
# If x is smaller, x is in left subarray
if arr[pos] > x:
return interpolationSearch(arr, lo,
pos - 1, x)
return -1
# Driver code
# Array of items in which
# search will be conducted
arr = [10, 12, 13, 16, 18, 19, 20,
21, 22, 23, 24, 33, 35, 42, 47]
n = len(arr)
# Element to be searched
x = 19
index = interpolationSearch(arr, 0, n - 1, x)
if index != -1:
print("Element found at index", index)
else:
print("Element not found")
# This code is contributed by Hardik Jain
OutputElement found at index 5
Time Complexity: O(log2(log2 n)) for the average case, and O(n) for the worst case
Auxiliary Space: O(1)
The Interpolation search is a practical searching algorithm particularly for the uniformly distributed sorted arrays. It provides improvement over binary search by the efficiently estimating the probable position of the target element. However, it requires the array to be uniformly distributed and sorted and its performance can degrade in the certain cases.
Share your thoughts in the comments
Please Login to comment...