Interactive Data Visualizations in JavaScript
Last Updated :
04 Oct, 2023
In this article we will learn about Interactive Data visualization in JavaScript, Data visualizations serve as a powerful tool for visually representing information and allowing viewers to easily perceive trends, patterns, and relationships in large datasets. Static visuals often fall short when providing an immersive experience that encourages exploration and understanding.
We will explore all the above methods along with their basic implementation with the help of examples.
Approach 1: Vanilla JavaScript and SVG
Vanilla JavaScript combined with Scalable Vector Graphics can be used to create interactive data visualizations and SVG provides a flexible and scalable way to render graphics on the web.
Example: In this example, we are using SVG to create a bar chart with two bars of different heights. When hovering over a bar, its color changes from pink to green.
HTML
<!DOCTYPE html>
< html >
< head >
< title >SVG Chart</ title >
< style >
.bar {
fill: Pink;
transition: fill 0.2s;
}
.bar:hover {
fill: green;
}
</ style >
</ head >
< body >
< svg id = "chart" width = "250" height = "500" >
</ svg >
< script >
const data = [200, 80];
const svg = document.getElementById('chart');
const Width = svg.getAttribute('width') / data.length;
const GFG = 10;
for (let i = 0; i < data.length ; i++) {
const rect =
document .createElementNS(
"...", 'rect');
rect.setAttribute('class', 'bar');
rect.setAttribute('x', i * (Width + GFG));
rect.setAttribute('y', svg.getAttribute('height') - data[i]);
rect.setAttribute('width', Width);
rect.setAttribute('height', data[i]);
svg.appendChild(rect);
}
</script>
</ body >
</ html >
|
Output:
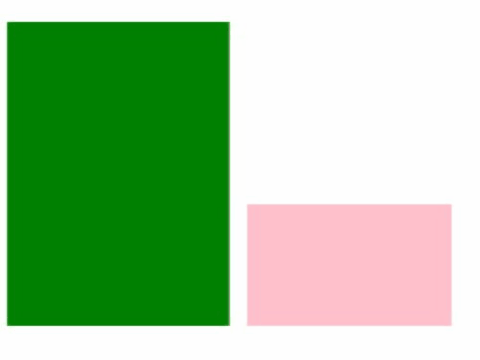
Approach 2: Using D3.js Library
The D3.js is a powerful JavaScript library for the creating interactive data visualizations and provides a wide range of tools for the binding data to the DOM and manipulating the document based on data.
Example: In this example, This interactive D3.js chart displays bars with heights based on provided data. When hovering over a bar, its color changes from green to red.
HTML
<!DOCTYPE html>
< html >
< head >
< title >The Interactive D3.js Chart</ title >
< script src =
</ script >
< style >
.bar {
fill: green;
transition: fill 0.2s;
}
.bar:hover {
fill: red;
}
</ style >
</ head >
< body >
< div id = "chart" ></ div >
< script >
const data = [18, 34, 22, 28];
const Width = 250;
const Height = 150;
const svg = d3.select("#chart")
.append("svg")
.attr("width", Width)
.attr("height", Height);
const barWidth1 = Width / data.length;
const barSpacing1 = 10;
svg.selectAll("rect")
.data(data)
.enter()
.append("rect")
.attr("class", "bar")
.attr("x", (d, i) => i * (barWidth1 + barSpacing1))
.attr("y", d => Height - d * 5)
.attr("width", barWidth1)
.attr("height", d => d * 5);
</ script >
</ body >
</ html >
|
Output:
Approach 3: Using jQuery
In this approach, Using jQuery, create interactive data visualizations by attaching event listeners to DOM elements, enabling smooth animations and transitions for enhanced user experience.
Example: In this example, we create an interactive bar that expands and changes color on hover. The page also includes a green title.
HTML
<!DOCTYPE html>
< html >
< head >
< title >jQuery Interactive Bar Example</ title >
< script src =
</ script >
< style >
.bar {
width: 50px;
height: 150px;
background-color: green;
transition: width 0.2s, background-color 0.2s;
}
h2 {
color: green;
}
</ style >
</ head >
< body >
< h2 >GeeksforGeeks</ h2 >
< div class = "bar" ></ div >
< script >
$(document).ready(function () {
$('.bar').hover(function () {
$(this).stop().animate(
{
width: '100px',
backgroundColor: 'red'
}, 'fast');
}, function () {
$(this).stop().animate(
{
width: '50px',
backgroundColor: 'blue'
},
'fast');
});
});
</ script >
</ body >
</ html >
|
Output:
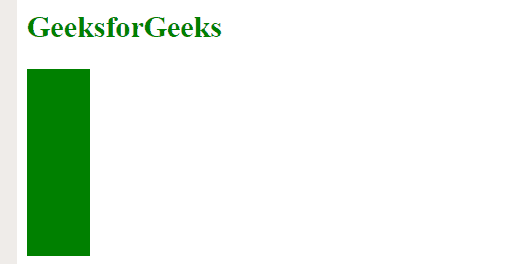
Share your thoughts in the comments
Please Login to comment...