Importing Multiple Files in Python
Last Updated :
26 Mar, 2024
Here, we have a task to import multiple files from a folder in Python and print the result. In this article, we will see how to import multiple files from a folder in Python using different methods.
Import Multiple Files From a Folder in Python
Below, are the methods of importing multiple files from a folder in Python.
- Using os and open
- Using glob Method
- Using pandas Method
File structure :
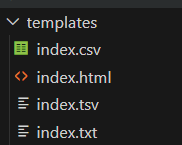
index.txt : GeeksforGeeks
index.csv : GeeksforGeeks
index.tsv : GeeksforGeeks
index.html : <h1>GeeksforGeeks</h1>
Import Multiple Files From A Folder using OS and Open
In this code example, below code utilizes the `os` module to list files in the “templates” folder. It then iterates through the files, reads those with a “.txt” extension, and prints their content. The script uses the `open` function to access each file, reads its content.
Python3
import os
folder_path = "templates"
files = os.listdir(folder_path)
for file in files:
if file.endswith(".txt"):
file_path = os.path.join(folder_path, file)
with open(file_path, 'r') as f:
data = f.read()
# Process the data as needed
print(f"File: {file}\nData:\n{data}\n{'=' * 30}")
Output
File: index.txt
Data:
GeeksforGeeks
==============================
Import Multiple Files From A Folder using glob Method
In this example, below uses the glob module to create a list of CSV files in the “templates” folder. It then iterates through the list, opens each file, reads its content, and prints file information along with the data. The script employs the os.path.join function to construct the file path and displays a separator line after each file’s data.
Python3
import glob
import os
folder_path = "templates"
csv_files = glob.glob(os.path.join(folder_path, '*.csv'))
for file in csv_files:
with open(file, 'r') as f:
data = f.read()
# Process the CSV data as needed
print(f"File: {file}\nData:\n{data}\n{'=' * 30}")
Output
File: templates\index.csv
Data:
GeeksforGeeks
==============================
Import Multiple Files From A Folder using pandas Method
In this example, below Python code the `Pandas` library to read multiple HTML files from the “templates” folder. It uses `glob` to create a list of HTML files, reads each file into a Pandas DataFrame, and concatenates them into a single DataFrame named `combined_data`. The final DataFrame can be further processed as needed, and its contents are printed.
Python3
import pandas as pd
import glob
import os
folder_path = "templates"
csv_files = glob.glob(os.path.join(folder_path, '*.html'))
dfs = [pd.read_csv(file) for file in csv_files]
combined_data = pd.concat(dfs, ignore_index=True)
# Process the combined_data dataframe as needed
print(combined_data)
Output
Empty DataFrame
Columns: [<h1>GeeksforGeeks</h1>]
Index: []
Share your thoughts in the comments
Please Login to comment...