Importing Python Functions from Other Scripts
Last Updated :
06 Feb, 2024
Modular programming in Python enables reuse and structure of code. To accomplish this, importing functions from other scripts or modules is a potent method. This procedure facilitates collaborative development, promotes concern separation, and improves code readability. The ideas and procedures involved in importing Python functions from one script to another will be covered in detail in this tutorial.
Importing Python Functions from Other File in Python
Below are some of the examples by which we can understand how we can import Python functions from another Python file in Python:
Example 1: Addition and Subtraction by Importing Python Function
In this example, two scripts are involved. The first script (`operations.py`) contains function for addition and subtraction. The second script (`main.py`) imports the entire module (`operations`) and utilizes the imported functions. The `import operations` statement makes all the functions from `operations.py` available in `main.py`.
operations.py
Python3
def add_numbers(a, b):
return a + b
def subtract_numbers(a, b):
return a - b
|
main.py
Python3
import operations
add = operations.add_numbers( 2 , 3 )
subtract = operations.subtract_numbers( 5 , 4 )
print ( "Addition Result:" , add)
print ( "Subtraction Result:" , subtract)
|
Output:
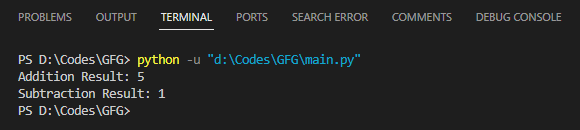
Video Demonstration
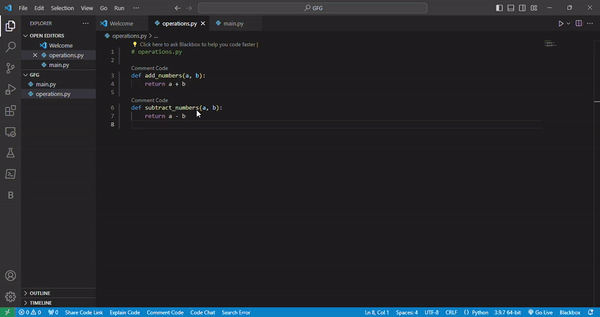
Example 2: Finding Area by Importing Python Functions from Other Scripts
In this example, we have two scripts. The first script (`geometry.py`) contains functions related to geometry. The second script (`main.py`) imports only the `area_of_square` function from the geometry module using the `from geometry import area_of_square` statement. This approach allows you to selectively import only the functions you need, reducing namespace clutter.
geometry.py
Python3
def area_of_rectangle(length, width):
return length * width
def area_of_square(side):
return side * side
|
main.py
Python3
from geometry import area_of_square
side = 4
result = area_of_square(side)
print ( "Area of Square:" , result)
|
Output:
Video Demonstration
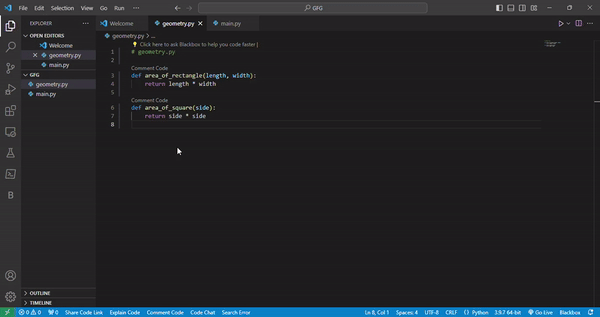
Example 3: Importing Class and Python Functions from Other Scripts
In this example, we’ll explore organizing complex modules and importing classes. Consider a scenario where we have a script (`shapes.py`) containing multiple classes representing different geometric shapes. The second script (`main.py`) imports these classes to create instances of shapes and calculate their areas.
shapes.py
Python3
class Circle:
def __init__( self , radius):
self .radius = radius
def area( self ):
return 3.14 * ( self .radius * * 2 )
class Rectangle:
def __init__( self , length, width):
self .length = length
self .width = width
def area( self ):
return self .length * self .width
|
main.py
Python3
from shapes import Circle, Rectangle
circle_instance = Circle(radius = 4 )
rectangle_instance = Rectangle(length = 4 , width = 6 )
print ( "Area of Circle:" , circle_instance.area())
print ( "Area of Rectangle:" , rectangle_instance.area())
|
Output:
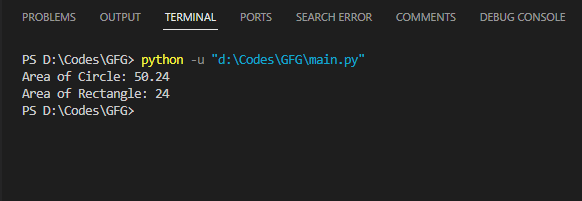
Video Demonstration
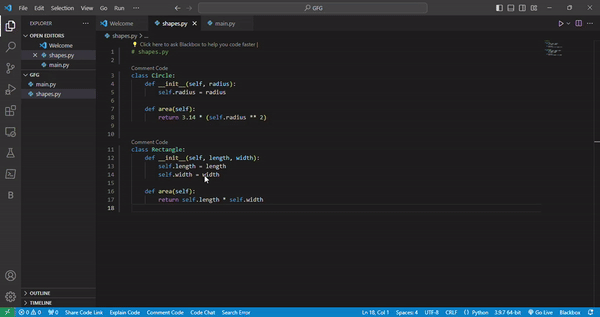
Share your thoughts in the comments
Please Login to comment...