How to use Regex Validator in Django?
Last Updated :
29 Sep, 2023
Django, a popular web framework, provides a built-in regex validator that allows you to enforce specific patterns on input data. Whether you need to validate user input, form fields, or any other data in your Django application, regex validators can be incredibly useful. In this article, we’ll explore how to use regex validators in Django.
Setting up the Project
Installation: To install Django.
Creating the Project
Create the project by using the command:
django-admin startproject bookstore
cd bookstore
Create an application named ‘mini’ by using the command:
python manage.py startapp mini
Now add this app to the ‘INSTALLED_APPS’ in the settings file.
Structuring Your Project
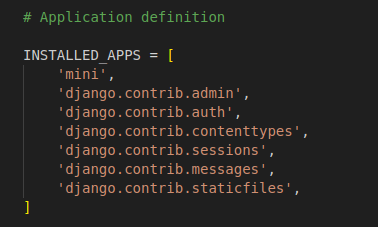
mini/models.py
This code defines a Django model called Vehicle with a registration_number field. The registration_number field is limited to a maximum length of 10 characters and is validated using a RegexValidator to ensure it follows the specified format. The ‘__str__’ method is provided to display the registration number when you print a Vehicle object.
Python3
from django.core.validators import RegexValidator
from django.db import models
class Vehicle(models.Model):
registration_number = models.CharField(
max_length = 10 ,
validators = [
RegexValidator(
regex = r '^[A-Z]{3}\d{3}$' ,
message = "Enter a valid registration number in the format ABC123." ,
code = "invalid_registration" ,
),
],
)
def __str__( self ):
return self .registration_number
|
mini/view.py
These views work together to allow users to add new vehicles with registration numbers following the specified format and to view a list of all vehicles in the system. The ‘add_vehicle’ view handles form submissions, including regex validation, while the vehicle_list view displays the list of vehicles stored in the database.
Python3
from django.shortcuts import render, redirect
from .models import Vehicle
from .forms import VehicleForm
def add_vehicle(request):
if request.method = = 'POST' :
form = VehicleForm(request.POST)
if form.is_valid():
form.save()
return redirect( 'vehicle_list' )
else :
form = VehicleForm()
return render(request, 'add_vehicle.html' , { 'form' : form})
def vehicle_list(request):
vehicles = Vehicle.objects. all ()
return render(request, 'vehicle_list.html' , { 'vehicles' : vehicles})
|
mini/form.py
By defining VehicleForm in this manner, you are essentially creating a form that is tightly integrated with the Vehicle model.
Python3
from django import forms
from .models import Vehicle
class VehicleForm(forms.ModelForm):
class Meta:
model = Vehicle
fields = [ 'registration_number' ]
|
templates/add_vehicle.html
This file used to add vehicle details.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Add Vehicle</ title >
</ head >
< body >
< h1 >Add Vehicle</ h1 >
< form method = "post" >
{% csrf_token %}
{{ form.as_p }}
< button type = "submit" >Save</ button >
</ form >
</ body >
</ html >
|
templates/vehicle_list.html
This HTML file is used for listing the vehicles.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Vehicle List</ title >
</ head >
< body >
< h1 >Vehicle List</ h1 >
< ul >
{% for vehicle in vehicles %}
< li >{{ vehicle }}</ li >
{% empty %}
< li >No vehicles in the list.</ li >
{% endfor %}
</ ul >
< a href = "{% url 'add_vehicle' %}" >Add Vehicle</ a >
</ body >
</ html >
|
mini/urls.py
Configure your app’s URLs to map to the views you’ve created:
Python3
from django.urls import path
from . import views
urlpatterns = [
path( 'add-vehicle/' , views.add_vehicle, name = 'add_vehicle' ),
path( 'vehicle-list/' , views.vehicle_list, name = 'vehicle_list' ),
]
|
urls.py
Include your app’s URLs in the project’s main urls.py:
Python3
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path( 'admin/' , admin.site.urls),
path( 'vehicles/' , include( 'your_app.urls' )),
]
|
Output
Share your thoughts in the comments
Please Login to comment...