How to use ReactJS with HTML Template ?
Last Updated :
29 Nov, 2023
We all know ReactJS is a front-end development framework. For the front end, we need to create HTML templates. So, this article teaches you how to use HTML templates in ReactJS. The process is very simple.
Prerequisites:
Approach:
We will create a simple one-page HTML template in React js. They have different sections like home blog contact us and courses etc. It looks like same as a normal HTML and CSS template.
Steps to Create the React Application:
Step 1: You will start a new project using create-react-app so open your terminal and type:
npx create-react-app myreact
Step 2: Now go to your react-footer folder by typing the given command in the terminal:
cd myreact
Step 3: Now we will create an assets folder in the public directory and create a style.css file. The path will be:
public/assets/style.css
Project Structure:
.png)
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Step 4: Create an HTML template or download:
First, we need to create an HTML template to use in React JS. If you don’t have an HTML template then simply download it.
Step 5: Below is the code example
- Add your CSS and JavaScript link to it, then index.html looks like as follows:
- Add a blank Fragment tag and paste the body part of the HTML template in it. Follow the below code.
- We can add CSS for styling. For that, we need to edit style.css located in public/assets folder. Then style.css looks like as follows:
Javascript
import React from 'react' ;
function App() {
return (
<>
<div id= "container" >
<div id= "header" >
<nav>
<h1>Geeksforgeeks</h1>
<li><a href= '#header' >Home</a></li>
<li ><a href= "#extra" >Bolgs</a></li>
<li ><a href=
Contact US</a></li>
<li ><a href=
Courses</a></li>
</nav>
</div>
<div id= "wrapper" >
<div id= "content" >
<h1>Welcome to Geeksforgeeks</h1>
<h2>Build your career with Geeksforgeeks </h2>
<p>
Geeksforgeeks provides you to best
tutorials, articles, coding, courses etc.
<p>Geeksforgeeks DSA self placed code is
best course ever and it is made by experts.</p>
</p>
<button>Learn More</button>
</div>
</div>
<div id= "navigation" >
<img src=
alt= "logo" />
</div>
<div id= "extra" >
<div id= "card" >
<h3>Create Math captcha using PHP</h3>
<p>In this article we are implement Math captcha.</p>
<button>Read More</button>
</div>
<div id= "card" >
<h3>Style Google Custom Search Manually</h3>
<p>We are styling Google Custom Search
manually with CSS</p>
<button>Read More</button>
</div>
<div id= "card" >
<h3>What is Interaction Manager.</h3>
<p>In this article we will see what is
Interaction Manager and how use it.</p>
<button>Read More</button>
</div>
</div>
<div id= "footer" ><p>Copyright@2007</p>
</div>
</div>
</>
)
}
export default App;
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" />
< meta name = "viewport"
content = "width=device-width, initial-scale=1" />
< meta name = "theme-color"
content = "#000000" />
< meta name = "description"
content = "Web site created using create-react-app" />
< link rel = "stylesheet" href = "./assets/style.css" >
< title >Geeksforgeeks</ title >
</ head >
< body >
< noscript >You need to enable JavaScript
to run this app.</ noscript >
< div id = "root" ></ div >
< script type = "text/javascript" >
AddFillerLink("content", "navigation", "extra")
</ script >
</ body >
</ html >
|
CSS
html,
body {
margin : 0 ;
padding : 0 ;
}
body {
color : #292929 ;
font : 90% Roboto, Arial , sans-serif ;
font-weight : 300 ;
}
div#header {
position : relative ;
}
div#header h 1 {
height : 80px ;
line-height : 80px ;
margin : 0 ;
padding-left : 10px ;
background : green ;
color : white ;
margin-left : 5% ;
}
div#header nav {
position : relative ;
display : flex;
list-style : none ;
margin : 0 ;
padding-left : 10px ;
background : green ;
color : white ;
}
div#header li {
align-items: center ;
justify- content : center ;
margin-top : 28px ;
padding-left : 10% ;
color : white ;
}
div#header li a {
color : #fff ;
text-decoration : none ;
}
div#navigation img {
width : 30 rem;
height : 22 rem;
margin : 6px ;
}
div#extra {
display : flex;
padding : 0px ;
margin : auto ;
}
div#extra #card {
width : 20 rem;
height : 13 rem;
margin : 5% ;
padding : 5px ;
border : 2px solid #292929 ;
}
div#extra #card button {
height : 50px ;
width : 10 rem;
background-color : rgb ( 8 , 62 , 8 );
color : #fff ;
border : none ;
position : absolute ;
bottom : 4px ;
border-radius: 10px ;
margin : 20px ;
}
div#footer {
background : #42444e ;
color : #fff ;
}
div#footer p {
padding : 20px 10px ;
text-align : center ;
}
div#wrapper {
float : left ;
width : 60 ;
margin-left : 5% ;
}
div#wrapper#content {
text-align : center ;
}
div#content h 1 {
font-size : 50px ;
font-weight : 800 ;
color : rgb ( 11 , 49 , 11 );
}
div#content button {
height : 50px ;
width : 10 rem;
background-color : rgb ( 8 , 62 , 8 );
color : #fff ;
border : none ;
border-radius: 10px ;
margin : 20px ;
}
div#navigation {
float : right ;
width : 40% ;
}
div#extra {
clear : both ;
width : 100% ;
}
|
Step to Run Application: Run the application using the following command from the root directory of the project.
npm start
Output:
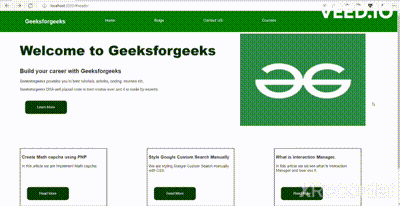
HTML template with react js
Share your thoughts in the comments
Please Login to comment...