How to Use Fetch in TypeScript ?
Last Updated :
16 Jan, 2024
In TypeScript, fetching the data from any server or API using fetch() API is not the same as we used to do it in Plain or Vanilla JavaScript. Let us see how we can use the fetch API to fetch data from any server or API in TypeScript.
NOTE: Before using this code, please make sure that you have jQuery installed in your project folder or work directory.
Syntax:
async function func_name(url: string): Promise<any>{
const response = await fetch(api_url, method/request type, [other_params]);
const data = await response.json();
return data;
}
Approach
- Create an HTML file with the name index.html to structure our web page.
- Use the different HTML elements like div, heading, paragraph, etc to structure our web page.
- Now, create an index.ts file to write the TypeScript code for using the fetch API.
- Use a random API that provides the random data with the fetch API to fetch the data using the GET request.
- Create the dynamic HTML to show the fetched data to the user on the screen.
Example 1: The below code example uses the fetch API with async await and the try-catch blocks to fetch data and display it to the user by generating dynamic HTML.
Javascript
import $ from 'jquery' ;
declare var global: any;
global.jQuery = $;
import 'jquery-ui' ;
async function fetchGET(url: string): Promise<any> {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(
`Unable to Fetch Data, Please check URL
or Network connectivity!!`
);
}
const data = await response.json();
return data.results[0];
} catch (error) {
console.error( 'Some Error Occured:' , error);
}
}
async function clickHandler() {
try {
const returnedData =
$( '#currentUser' ).html(
`
Current User: <br/><br/>
<img style= "border-radius: 50%" src=
${returnedData.picture.medium} alt= "User Image" />
<p>
Name:
<b>
${returnedData.name.title}
${returnedData.name.first}
${returnedData.name.last}
</b>
</p>
<p>
Email:
<b>
${returnedData.email}
</b>
</p>
<p>
Country:
<b>
${returnedData.location.country}
</b>
</p>
<p>
Phone:
<b>
${returnedData.phone}
</b>
</p>
<p>
Gender:
<b>
${returnedData.gender}
</b>
</p>
`);
} catch (error) {
console.error( 'Some Error Occurred:' , error);
}
}
clickHandler();
$( '#btn' ).on( 'click' , clickHandler);
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" />
< title >
Using fetch in TypeScript
</ title >
< style >
.app {
text-align: center;
}
.h1 {
font-size: 30px;
color: green;
}
#result {
color: #FF671F;
}
</ style >
</ head >
< body >
< div class = "app" >
< h1 class = "h1" >
GeeksforGeeks
</ h1 >
< h3 id = "result" >
Click the below button to fetch
the data of new current user.</ h3 >
< button id = "btn" >
Change Current User
</ button >< br />< br />
< div id = "currentUser" ></ div >
</ div >
</ body >
</ html >
|
Output:
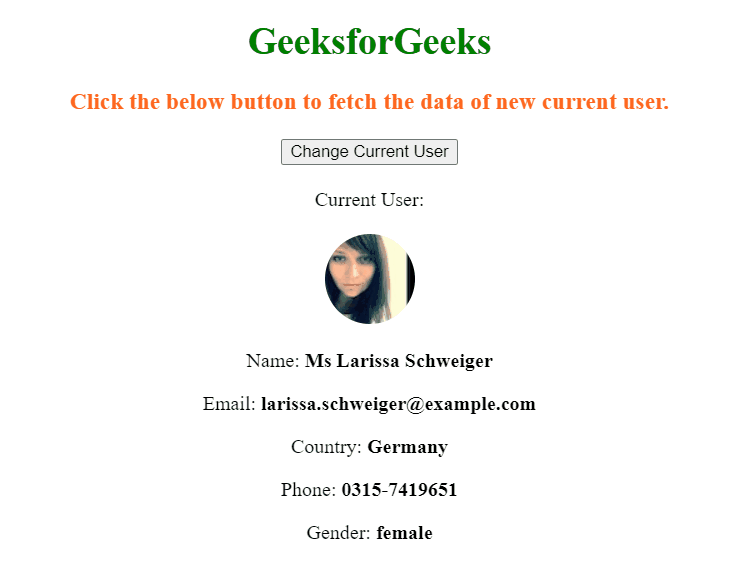
Example 2: The below code example uses the fetch() API without async await and the try-catch blocks.
Javascript
import $ from 'jquery' ;
declare var global: any;
global.jQuery = $;
import 'jquery-ui' ;
function fetchGET(url: string): Promise<any> {
return fetch(url).then((response) => {
if (!response.ok) {
throw new Error(
`Unable to Fetch Data, Please check
URL or Network connectivity!!`
);
}
return response.json();
})
.then((data) => data.results[0])
. catch ((error) => console.log(error));
}
async function clickHandler() {
try {
const returnedData =
$( '#currentUser' ).html(`
Current User: <br/><br/>
<img style= "border-radius: 50%" src=
${returnedData.picture.medium} alt= "User Image" />
<p>
Name:
<b>
${returnedData.name.title}
${returnedData.name.first}
${returnedData.name.last}
</b>
</p>
<p>
Email:
<b>
${returnedData.email}
</b>
</p>
<p>
Country:
<b>
${returnedData.location.country}
</b>
</p>
<p>
Phone:
<b>
${returnedData.phone}
</b>
</p>
<p>
Gender:
<b>
${returnedData.gender}
</b>
</p>
`);
} catch (error) {
console.error
( 'Some Error Occurred:' , error);
}
}
clickHandler();
$( '#btn' ).on( 'click' , clickHandler);
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" />
< title >
Using fetch in TypeScript
</ title >
< style >
.app {
text-align: center;
}
.h1 {
font-size: 30px;
color: green;
}
#result {
color: #FF671F;
}
</ style >
</ head >
< body >
< div class = "app" >
< h1 class = "h1" >
GeeksforGeeks
</ h1 >
< h3 id = "result" >
Click the below button to fetch
the data of new current user.</ h3 >
< button id = "btn" >
Change Current User
</ button >< br />< br />
< div id = "currentUser" ></ div >
</ div >
</ body >
</ html >
|
Output:
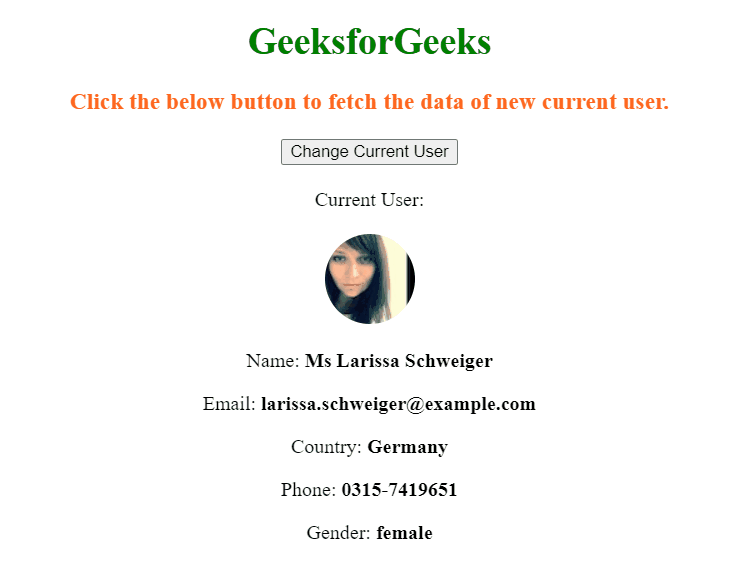
Share your thoughts in the comments
Please Login to comment...