How to Fetch XML with Fetch API in JavaScript ?
Last Updated :
18 Apr, 2024
The JavaScript fetch() method retrieves resources from a server and produces a Promise. We will see how to fetch XML data with JavaScript’s Fetch API, parse responses into XML documents, and utilize DOM manipulation for streamlined data extraction with different methods.
These are the following methods:
Using response.text() Method
To fetch the XML data, use the fetch() function. However, as the Fetch API lacks native XML parsing support, handling the response involves alternative methods. Convert it to text and then parse it using an XML parser like DOMParser. First, use the fetch() function to request the XML file. If the response is successful, convert it to text using the response.text(). Use DOMParser to parse the XML text into an XML document. Handle any errors that may occur during the fetch operation or XML parsing.
Example: This example shows the implementation of the above-explained approach.
JavaScript
fetch('https://codetogo.io/api/users.xml')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.text();
})
.then(xmlText => {
const parser = new DOMParser();
const xmlDoc = parser.parseFromString(xmlText,
'text/xml');
// Now you can work with the parsed XML document
console.log(xmlDoc);
})
.catch(error => {
console.error(`There was a problem with
the fetch operation:`, error);
});
Output: The browser console will show you the output.
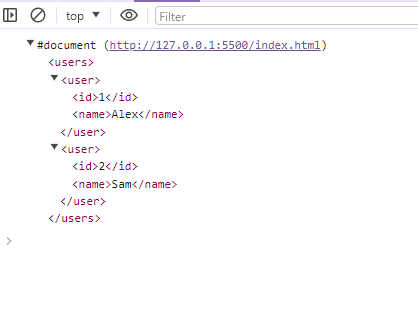
Using FileReader Object
To fetch XML data using both the Fetch API and the FileReader API in JavaScript, first use the Fetch API to retrieve the XML file. Then, utilize the response.blob() method to obtain the response body as a Blob object. Next, employ the FileReader API to read the Blob as text using FileReader.readAsText(). Finally, parse the XML text into a document object using DOMParser, enabling further processing of the XML data within the application.
Example: This example shows the implementation of the above-explained approach.
JavaScript
fetch('https://codetogo.io/api/users.xml')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok');
}
return response.blob();
})
.then(blob => {
const reader = new FileReader();
reader.onload = function (event) {
const xmlText = event.target.result;
const parser = new DOMParser();
const xmlDoc = parser.parseFromString(xmlText,
'text/xml');
// Now you can work with the xmlDoc
console.log(xmlDoc);
};
reader.readAsText(blob);
})
.catch(error => {
console.error(`There was a problem with
the fetch operation:`, error);
});
Output: The browser console will show you the output.
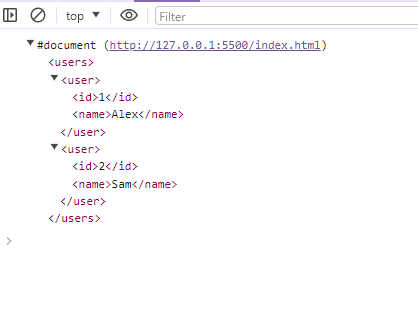
Note: The DOMParser method will give error if you run that in NodeJs environment. You must use browser console to see your output by linking it to the HTML file. Also, you must use CORS chrome extension that will allow you to use fetch function and DOMParser.
Share your thoughts in the comments
Please Login to comment...