How to use Bootstrap in React JS ?
Last Updated :
14 Dec, 2023
Explore the seamless integration of Bootstrap into React JS for enhanced styling and responsive web development. This comprehensive guide provides step-by-step instructions, covering the installation process, utilization of Bootstrap components, and best practices to harness the power of both technologies.
There are the following ways we can use Bootstrap in our React JS Project.
1. Using Bootstrap CDN:
The simplest way to use Bootstrap in your React application is to use BootstrapCDN. There are no downloads or installations needed. Simply provide a link in the head section of your app, as seen in the example below.
<link rel=”stylesheet” href=”https://stackpath.bootstrapcdn.com/bootstrap/ 4.1.0/css/bootstrap.min.css” integrity=”sha384-9gVQ4dYFwwWSjIDZnLEWnxCjeSWFphJiwGPXr1jddIhOegiu1FwO5qRGvFXOdJZ4″ crossorigin=”anonymous”>
2. Using the react-bootstrap library:
This is the recommended method for applying Bootstrap to your React program if you’re using a build tool or a package bundler like webpack. Bootstrap must be installed as a dependency for your app.
npm install bootstrap
3. Install a React Bootstrap package:Â
The third choice for integrating Bootstrap into a React app is to use a package that includes rebuilt Bootstrap components that can be used as React components. The following are the two most common packages:
- react-bootstrap
- bootstrap
In the following example, we have implemented the first way to use Bootstrap in the ReactJS project.
Steps to Create React Application:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: Installing the required dependencies:
npm install react-bootstrap bootstrap
Project Structure:
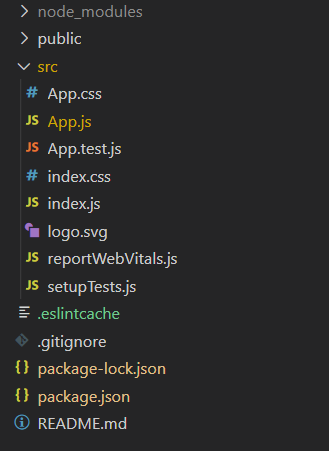
The updated dependencies in package.json file will look like:
"dependencies": {
"bootstrap": "^5.3.2",
"react": "^18.2.0",
"react-bootstrap": "^2.9.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example: Now write down the following code in the App.js file.
Javascript
import React, { useState } from 'react' ;
class App extends React.Component {
constructor(props) {
super (props)
this .state = {
counter: 0
}
this .handleClick = this .handleClick.bind( this );
}
handleClick = () => {
console.log( "hi" )
alert( "The value of counter is :" + this .state.counter)
this .setState({ counter: this .state.counter + 1 })
}
render() {
return (
<div >
<div className= "jumbotron" >
<h1 className= "display-4" >Hello From GFG!</h1>
<p className= "lead" >This is a simple Example of using
bootstrap in React.</p>
<hr className= "my-4" />
<p>the Component is called jumbotron in bootstrap.</p>
<p className= "lead" >
<a onClick={ this .handleClick}
className= "btn btn-primary btn-lg" href= "#"
role= "button" >Click me</a>
</p>
</div>
</div>
);
}
}
export default App;
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output: Now open your browser and go to http://localhost:3000
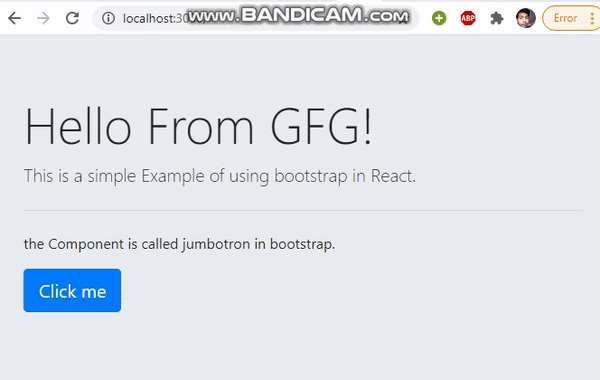
Share your thoughts in the comments
Please Login to comment...