How to test query strings in Postman requests ?
Last Updated :
05 Jan, 2024
To test query strings in Postman requests, we only need to simply enter the key and value of each parameter and Postman will append them to the URL automatically. To the end of the request URL, the parameters are appended using ‘?’ and key-value pairs are separated by ‘&’.
e.g. :- ?id=22&type=vivek
Prerequisites
There are 2 approaches or methods to test query strings in postman are :
1. Using URL:
In this approach, we try to append the query strings to the end of the API URL. It is the most common method to test query string in postman requests. We can directly paste the API URL into Postman String bar and select HTTP Method.
example:-
- http://localhost:3000/name
- http://localhost:3000/students
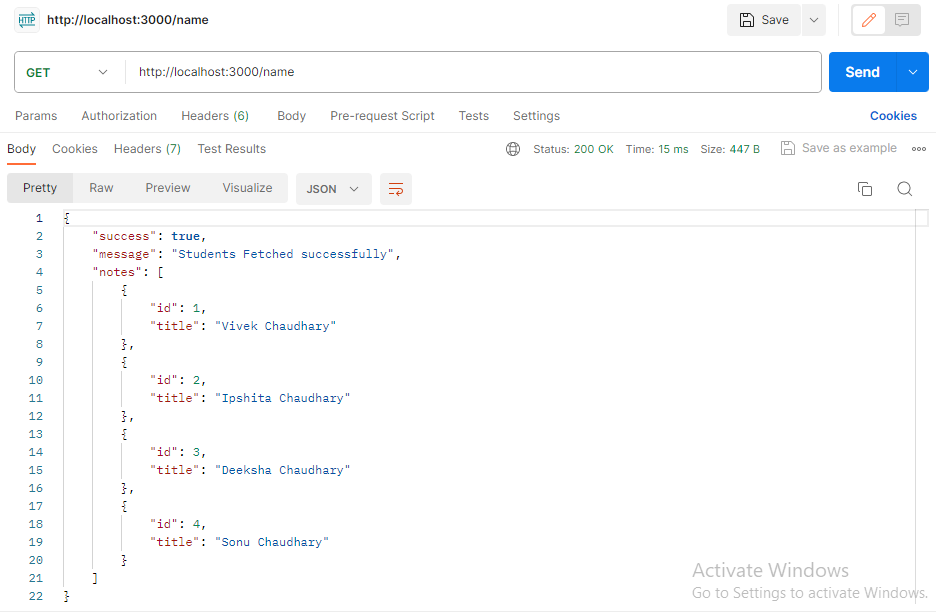
URL Output – http://localhost:3000/name
2. Using Parameters:
Using Parameters in a query string allows you to enter the parameters to your API and fetch for the specific data as defined by those parameters. We can pass parameters directly to the API or we can simply enter them in the key-value pairs field. After enter the parameters in the API or if you choose to enter in the params fields then these parameters will automatically added to the API.
Example:
- http://localhost:3000/name/1
- http://localhost:3000/name/2
Output for: http://localhost:3000/name/1
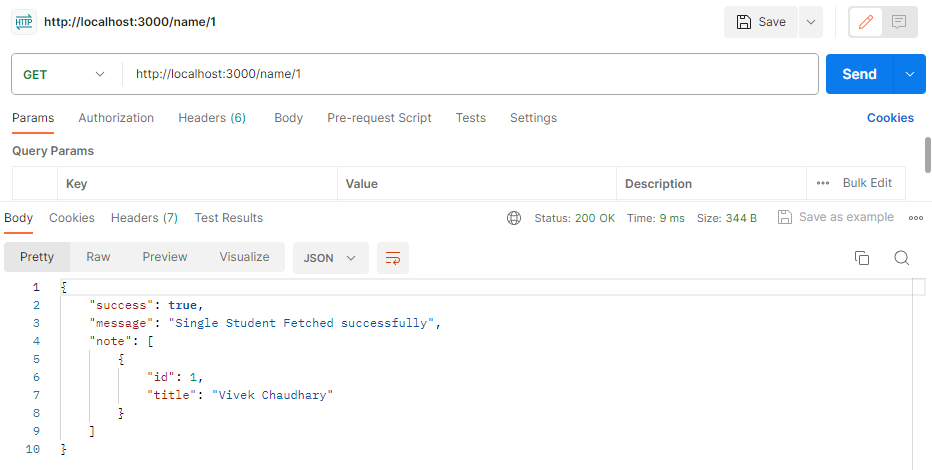
Output – http://localhost:3000/name/1
Output for – http://localhost:3000/name/2
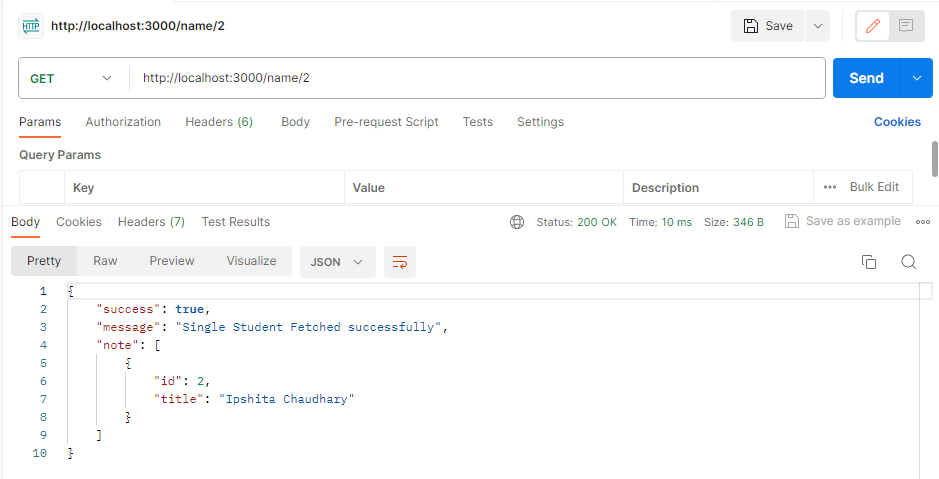
Output – http://localhost:3000/name/2
Steps to Create an Express Application:
Step 1: Create an node application
npm init -y
Step 2: Install the express and mysql2
npm install express
npm install mysql2
Project Structure:
.png)
Project Structure
Step 3: Create the files using as “schema.sql“, “database.js” and “index.js” in your application, Below is the code for each file ( You can checkout here to connect express application using mysql )
Javascript
import express, { json } from "express" ;
import { getNames } from "./database.js" ;
import { getName } from "./database.js" ;
const app = express();
const port = process.env.PORT || 3000;
app.get( '/name' , async (req, res) => {
const notes = await getNames();
return res.status(200).json({
success: true ,
message: "Students Fetched successfully" ,
notes: notes
})
});
app.get( '/name/:id' , async (req, res) => {
const id = req.params.id;
const note = await getName(id);
return res.status(200).json({
success: true ,
message: "Single Student Fetched successfully" ,
note: note
})
});
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
|
Javascript
import mysql from 'mysql2'
const pool = mysql.createPool({
host: 'localhost' ,
user: 'root' ,
password: 'YOUR_SQL_PASSWORD' ,
database: 'name'
}).promise()
export async function getNames() {
const [rows] = await pool.query( "SELECT * FROM name" )
return rows;
}
export async function getName(id) {
const [rows] = await pool.query(`
SELECT * FROM name WHERE id = ?`, [id])
return rows;
}
|
schema.sql:
--This is a comment in sql
--schema.sql
--create a new database
CREATE DATABASE Students;
--use Students database as current database
USE Students;
--create a table name in Students Database
CREATE TABLE name (
id integer AUTO_INCREMENT PRIMARY KEY,
title VARCHAR(255) NOT NULL
);
--Insert the values into the Students Database to test Query Strings
INSERT INTO name (title)
VALUES('Vivek Chaudhary'),('Ipshita Chaudhary'),('Deeksha Chaudhary'),('Sonu Chaudhary');
Output:
.png)
Output
Database Table Output:
.png)
Database Table
Share your thoughts in the comments
Please Login to comment...