How to Take Input in JavaScript in VS Code Terminal ?
Last Updated :
04 Mar, 2024
In JavaScript, capturing the user input through VScode Terminal can be done using various methods and approaches. These approaches are readline module, process.stdin, and process.argv.
Using readline module
In this approach, we are using the readline module to create an interface for reading input from the standard input (keyboard) in the VS Code Terminal. The ` rl.question` method prompts the user with ‘Enter Your Name:’, waits for their input, and executes a callback function to display the entered name.
Syntax:;l
const readline = require('readline');
Example: The below example uses a readline module to take input in JavaScript in VS Code Terminal.
Javascript
const readline = require( 'readline' );
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.question( 'Enter Your Name: ' , (input) => {
console.log(`Your Name is : ${input}`);
rl.close();
});
|
Output:
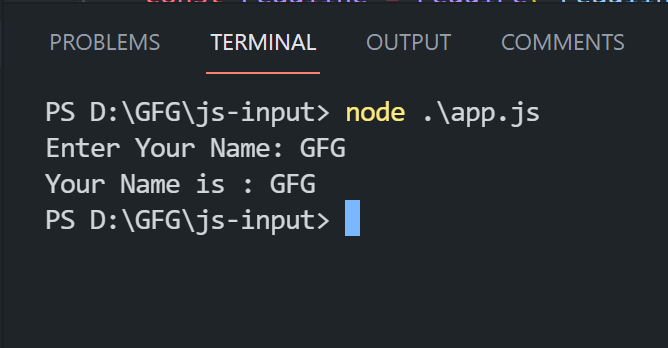
Using process.argv
In this approach, we are using process.argv, the script retrieves command-line arguments provided when executing the program. The code assigns the third element (process.argv[2]) to the variable input, and it checks whether a value is provided. If a value is present, it prints “Your Name is: [input]”, otherwise, it prints ‘No input provided.’
Syntax:
const arg1 = process.argv[2];
Example: The below example uses process.argv to take input in JavaScript in VS Code Terminal.
Javascript
const input = process.argv[2];
if (input) {
console.log(`Your Name is: ${input}`);
} else {
console.log( 'No input provided.' );
}
|
Output:
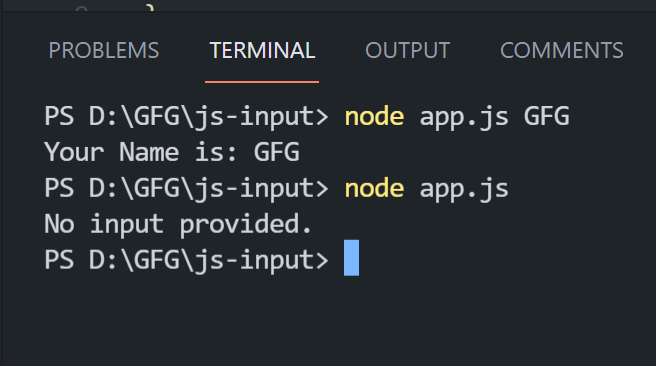
Using process.stdin
In this approach, we are using process.stdin, the script uses process.stdout.write to prompt the user with ‘Enter Your Name:’ and listens for data events on process.stdin to capture the user’s input. The entered data is then processed, trimmed of leading/trailing whitespace, and displayed as “Your Name is: [input]”. Finally, the script exits the process.
Syntax:
process.stdin.on();
Example: The below example uses process.stdin to take input in JavaScript in VS Code Terminal.
Javascript
process.stdout.write( 'Enter Your Name: ' );
process.stdin.on( 'data' , (data) => {
const input = data.toString().trim();
console.log(`Your Name is : ${input}`);
process.exit();
});
|
Output:
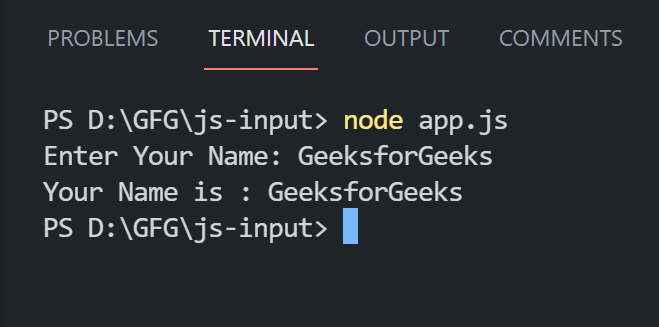
Share your thoughts in the comments
Please Login to comment...