How to take user input in JavaScript?
Last Updated :
22 Nov, 2023
Interacting with the user by taking inputs is essential to make an application engaging and user-friendly. Whether building a simple form validation script or a complex web application that relies on user interactions, mastering the art of taking user input in JavaScript is essential.
Approach: The “prompt” method
- The prompt method is part of the JavaScript window object. It displays a dialog box with a message to the user, an input field, and an OK and Cancel button. Users can enter text into the input field, and when they press OK, the input is returned as a string. If they press Cancel or close the dialog, null is returned.
- The prompt method is very helpful in situations where you require rapid and straightforward user input.
- The prompt is a blocking function, which means it halts JavaScript execution until the user interacts with the dialogue. As this can have an impact on the user experience in web applications, it’s frequently utilized for short input scenarios or fast interactions where blocking behavior is acceptable.
Syntax:
prompt("Message to the user")
Example: Dialog with the message “Please enter your name:”.
Javascript
const userInput = prompt( "Please enter your name:" );
|
Output:
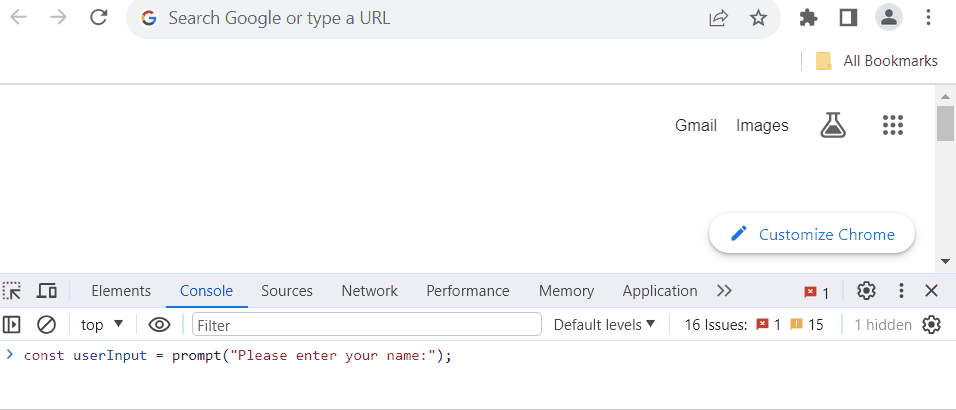
take user input with message “Please enter your name”
Example: Provide a default value in the prompt so that the input field will already contain the default value. Users can choose to modify the default value or enter their input.
Javascript
const userInput = prompt( "Please enter your name:" ,
"GeeksForGeeks User" );
|
Output:
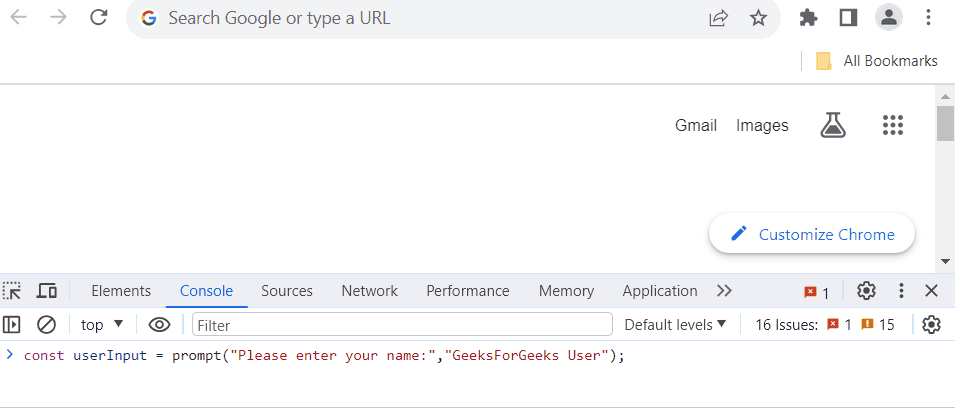
take user input with message “Please enter your name” and default value “GeeksforGeeks User”
Example: If you don’t provide any arguments to the prompt function (i.e., you call it without any message or default value), it will still open a dialog box, but the user won’t see any message or default text in the input field.
Javascript
const userInput = prompt();
|
Output:
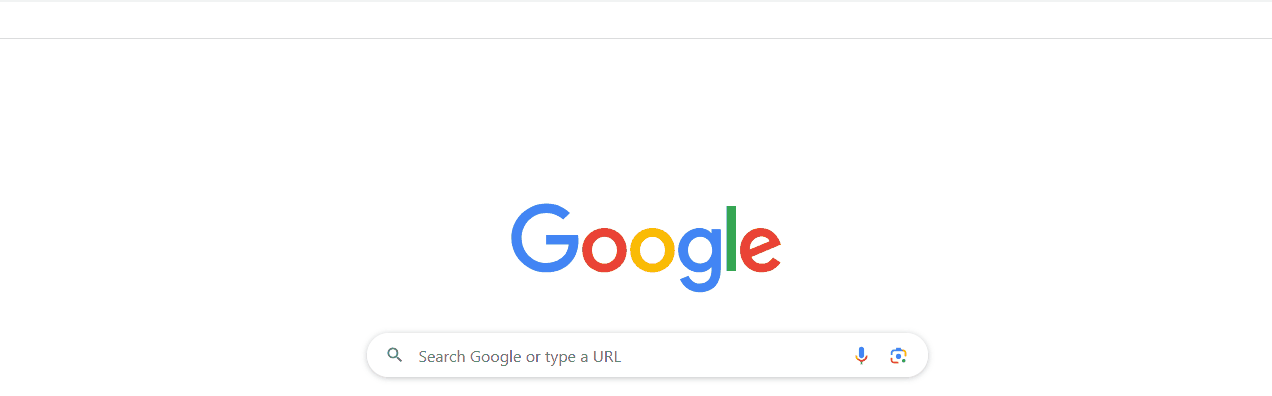
Example: we first check if the user provided input (didn’t press Cancel or close the dialog), and if so, we display a greeting. If the user canceled, we display a message indicating that they canceled the input.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content=" width = device -width,
initial-scale = 1 .0">
< title >Document</ title >
</ head >
< body >
< h1 >
How to take user input in JavaScript
</ h1 >
< script >
const userInput = prompt("Please enter your name:",
"GeeksForGeeks User");
// Handling user input
if (userInput !== null) {
alert("Hello, " + userInput + "!");
} else {
alert("You canceled the input.");
}
</ script >
</ body >
</ html >
|
Output:
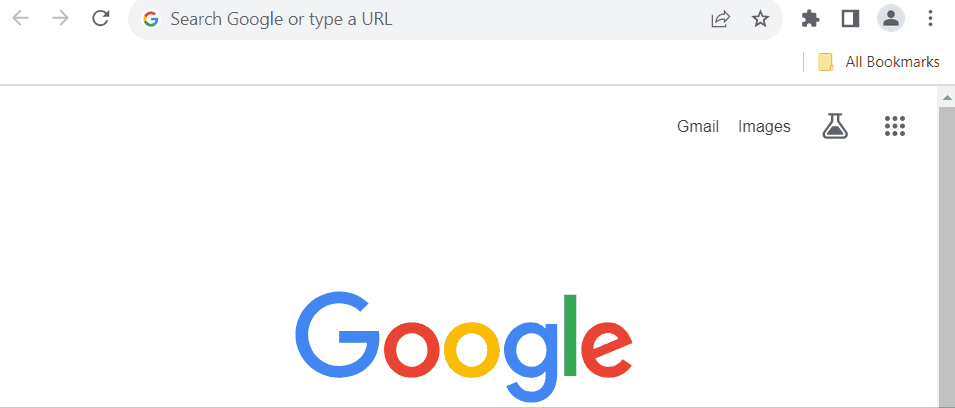
take user input and show the output
Share your thoughts in the comments
Please Login to comment...