How to Call a JavaScript Function from Chrome Console ?
Last Updated :
01 Apr, 2024
One can call a JavaScript Function from the Chrome Console. We will learn how can we call the function in the console that is written in JavaScript.
Steps to call a JavaScript Function from Chrome Console
Open the Chrome Console
You can open the Chrome Console by right-clicking on your webpage, selecting “Inspect” from the context menu, and then clicking on the “Console” tab. Alternatively, you can use the keyboard shortcut Ctrl+Shift+I in Windows or Cmd+Opt+I on Mac to open the Developer Tools and then click on the “Console” tab.
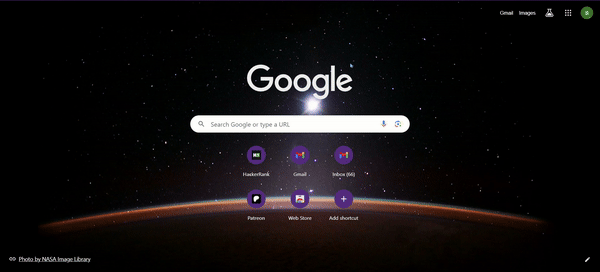
To Open the Console in the Chrome.
Call the Function
Once the console is open, you can call any JavaScript
function that is defined in the script(<script> </script>)tag in the HTML or in the JavaScript file by typing the function name followed by parentheses () and pressing Enter.
For example: If you have a function named myFunction, you would type myFunction() in the console and press Enter to execute it.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1></h1>
<script>
function myFunction(){
console.log(2+3);
}
</script>
</body>
</html>
Output:
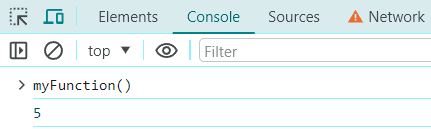
Output of the Code in the Console
Other Types of function calls
By Passing Arguments:
If your function requires arguments, you can include them within the parentheses.
Example: To demonstrate calling myFunction(arg1, arg2) would call myFunction with arg1 and arg2 as arguments in console.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1></h1>
<script>
function myFunction(x,y){
console.log(x+y);
}
</script>
</body>
</html>
Passing an x and y value as an arguments to the myFunction() in console as myFunction(6,7) gives an output of 13 in console;
Output:
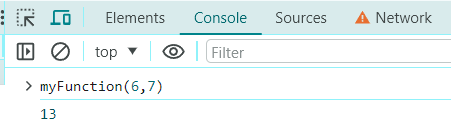
Output of argument myFunction().
By using Return Value
After executing the function, the console will display the return value of the function. If the function does not return a value, you might see undefined printed in the console. This is normal behavior for functions that do not explicitly return a value.
Example: In the above output 13 is the return value because in the code there is an return value like console.log(), if there is no return value is gives the output has Undefined.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1></h1>
<script>
function myFunction(x,y){
//No return value
}
</script>
</body>
</html>
Output:
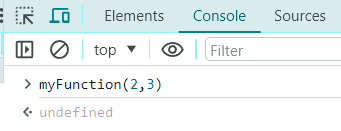
Output of the above code
Share your thoughts in the comments
Please Login to comment...