JavaScript Program to Print an Integer Entered by user
Last Updated :
22 Feb, 2024
Creating a JavaScript program to accept an integer from the user and then print it is a fundamental exercise that helps in understanding user input and output operations. In this article, we’ll explore different approaches to achieve this task in a JavaScript environment.
Approach 1: Using Prompt and Alert in Browser
One of the simplest ways to get user input and display output in a web browser is by using the prompt()
function to capture input and the alert()
function to display the output.
HTML
<!DOCTYPE html>
< html >
< body >
< script >
let userInteger = parseInt(prompt("Enter an integer:"));
alert(`You entered: ${userInteger}`);
</ script >
</ body >
</ html >
|
Output
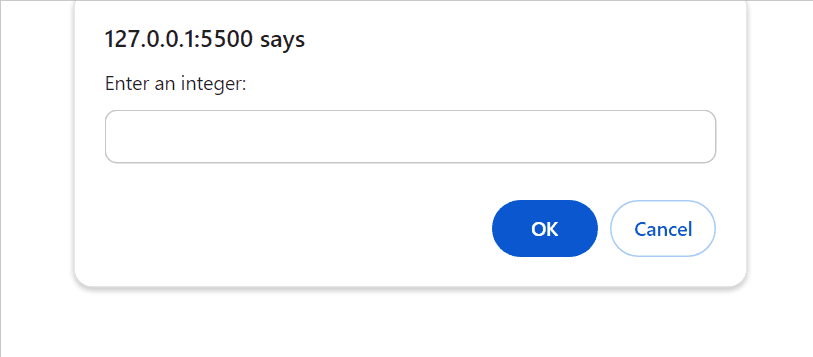
In this example, prompt()
displays a dialog box to the user to enter an integer, and parseInt()
is used to convert the input string to an integer. The alert()
function then displays the entered integer.
Approach 2: Using HTML Form and JavaScript
You can also create an HTML form to accept user input and use JavaScript to display the entered integer.
HTML
<!DOCTYPE html>
< html >
< body >
Enter an integer: < input type = "text" id = "integerInput" >
< button onclick = "printInteger()" >Submit</ button >
< script >
function printInteger() {
let userInteger = parseInt(
document.getElementById("integerInput").value);
alert(`You entered: ${userInteger}`);
}
</ script >
</ body >
</ html >
|
Output
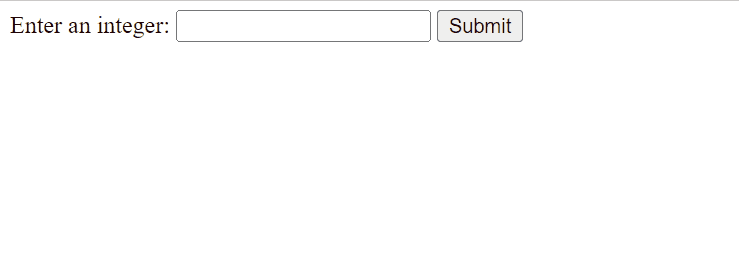
In this approach, the user’s input is captured from an HTML input element, and the printInteger()
function is called when the user clicks the “Submit” button. The function retrieves the value from the input field, converts it to an integer, and displays it using an alert box.
Share your thoughts in the comments
Please Login to comment...