How to Store Data in Local Storage using AngularJS ?
Last Updated :
26 Oct, 2023
Angular JS is a typescript-based web application framework. It is supported by the Angular team of Google and open-source developers. It is a component-based framework allowing the developers to reuse the components created. It is well-suited for large and complex applications because of its well-defined project structure, templates, etc.
Local Storage
Local Storage in Angular JS allows the developers to store some data in the browser itself to access it almost instantly without any HTTP request. Local Storage in the Website allows the users to save the data in the form of key-value pairs. It does not have any expiration date or TTL (Time to Live) and it doesn’t depend on the session. If we close the browser and reopen later, the values will be saved. It is the property of the window object and hence is accessible globally without requiring any libraries or importing any package. Different browsers have different storage locations and types. For Google Chrome, it is stored in the user profile directory, where Chrome is installed in an SQLite d database. In Firefox, local storage is stored in the webappsstore.sqlite file in the profile folder. It is suitable for small amounts of storage like strings, dates, or similar. Storing files is not suitable since it would then block the process and hence slow down the activity of the browser.
Syntax
The key-value pair always needs to be a string, i.e.
$window.localStorage.setItem(key,value):
Both the key and value are strings, so we need to provide strings only. local storage can save strings only. The key needs to string as well. Since the data is saved with the help of a key, the key needs to be unique for different values, otherwise, the data will be overwritten.
Steps to Configure the Angular JS Project
Step 1: Create a new project
ng new local_storage_gfg
Step 2: Open the project on any IDE or Text Editor(VSCode, or Sublime Text).
Step 3: To run the project, follow the below command
ng serve --open
Step 4: Replace the pre-existing code in the src>app>app.component.html with the following code examples.
Project Structure
The following project structure will be generated after completing the above steps.
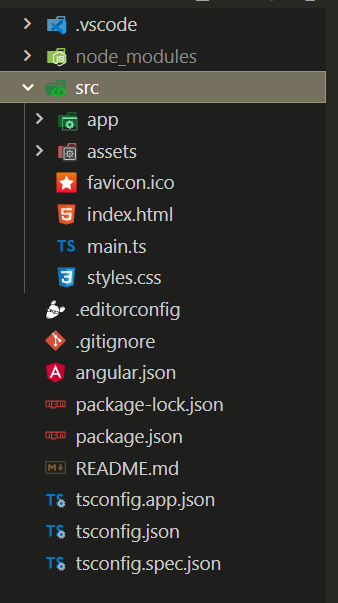
Approach
In this approach, we will use the $window.localStorage functionality of the browser to save data. The methods & their description are given below:
- $window.localStorage.setItem(key, value): To save data in local storage.
- $window.localStorage.getItem(key): To retrieve data based on the key from local storage.
- $window.localStorage.removeItem(key): To remove the key-value pair from local storage.
We will see how to store data in localStorage in AngularJS and also how to retrieve and later remove it from storage with the help of basic examples.
Example 1: This example illustrates the Simple Store and Retrieve the values from localstorage. Here, we have placed a simple input text, and when we press the save button, it is saved to the localstorage by calling a method and passing the contents.
HTML
< div >
< h1 style="color: green;
text-align: center;">
Welcome to GeeksforGeeks
</ h1 >
< h3 style = "text-align: center;" >
How to store data in local
storage using AngularJS?
</ h3 >
< input type = "text"
[(ngModel)]="name"
# ctrl = "ngModel"
placeholder = "Enter your name" />
< br >
< button (click)="saveName()">Save</ button >
< button (click)="getName()">Get</ button >
< br >
< strong >Name: {{storeValue}}</ strong >
</ div >
|
Javascript
import { Component } from "@angular/core" ;
@Component({
selector: "app-root" ,
templateUrl: "./app.component.html" ,
styleUrls: [ "./app.component.css" ],
})
export class AppComponent {
title = "local_storage_gfg" ;
name: String = "" ;
CopyText: String = "" ;
storeValue: String = "" ;
saveName() {
let name = String( this .name);
window.localStorage.setItem( "name" , name);
}
getName() {
this .storeValue =
window.localStorage.getItem( "name" )
?? "No Value Stored" ;
}
}
|
Javascript
import { NgModule } from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { FormsModule }
from '@angular/forms' ;
import { AppRoutingModule }
from './app-routing.module' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent,
],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output
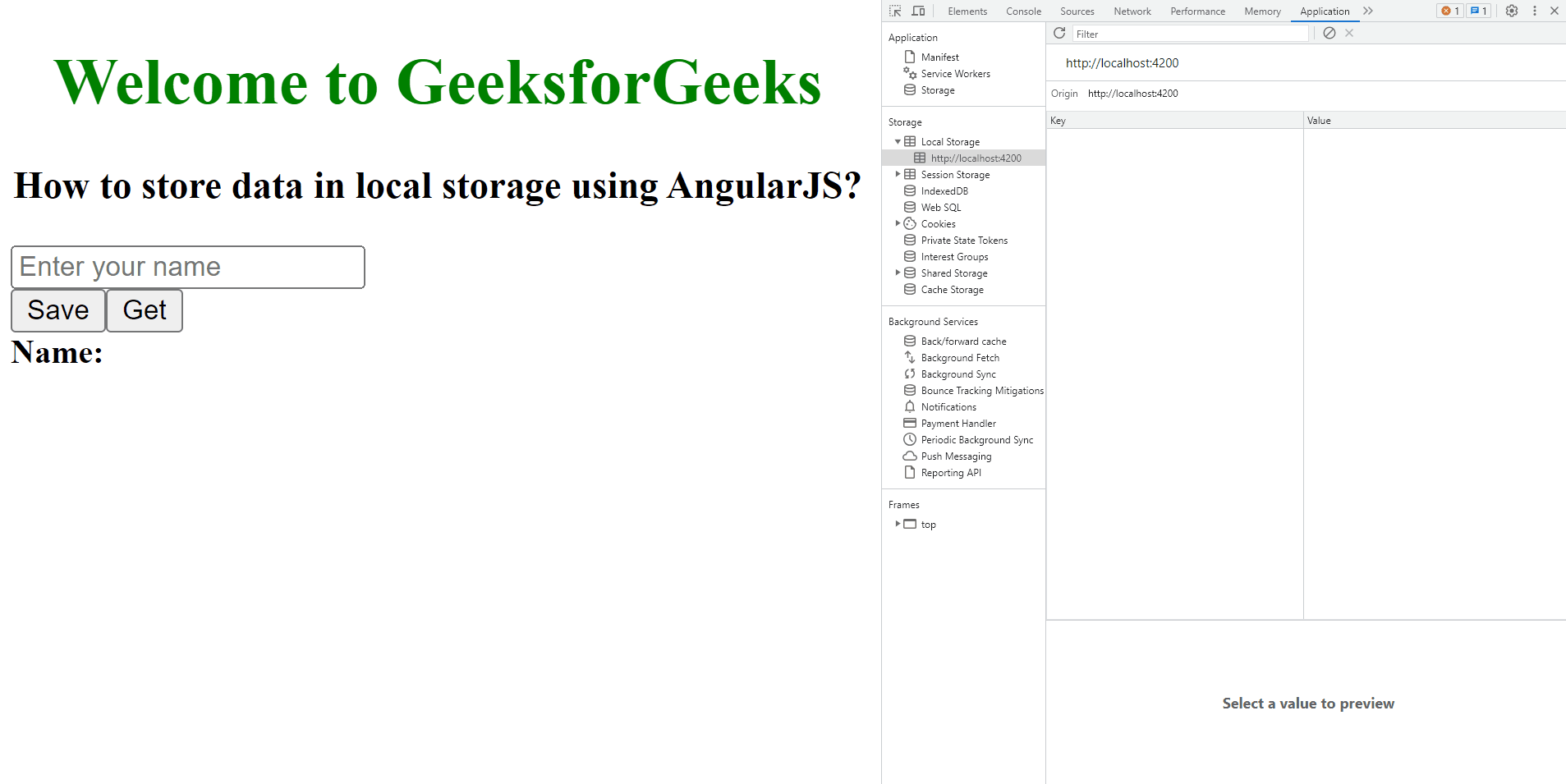
Example 2: This example illustrates the Store, Retrieve, and Remove the key-value pairs from Local Storage, i.e. we will store some key-value pairs and later remove them from storage. We will use all the three functionality of localStorage.
HTML
< div >
< h1 style="color: green;
text-align: center">
Welcome to GeeksforGeeks</ h1 >
< h3 style = "text-align: center" >
How to store data in local
storage using AngularJS?
</ h3 >
< strong >Store Value</ strong >
< br />
< input type = "text"
placeholder = "Enter key"
[(ngModel)]="key" />
< input type = "text"
placeholder = "Enter value"
[(ngModel)]="val" />
< button (click)="store()">
Store
</ button >
< br />
< br />
< strong >Get Value: {{getValue}}</ strong >
< br />
< input type = "text"
placeholder = "Enter key"
[(ngModel)]="retrievekey" />
< button (click)="retrieve()">
Get
</ button >
< br />
< br />
< strong >Remove Value</ strong >
< br />
< input type = "text"
placeholder = "Enter key to remove"
[(ngModel)]="removeKey" />
< button (click)="remove()">
Remove
</ button >
</ div >
|
Javascript
import { Component } from "@angular/core" ;
@Component({
selector: "app-root" ,
templateUrl: "./app.component.html" ,
styleUrls: [ "./app.component.css" ],
})
export class AppComponent {
title = "local_storage_gfg" ;
key: string = "" ;
val: string = "" ;
getValue: string = "" ;
removeKey: string = "" ;
retrievekey: string = "" ;
store() {
window.localStorage.setItem( this .key, this .val);
this .key = "" ;
}
retrieve() {
this .getValue =
window.localStorage.getItem( this .retrievekey) ??
"No value found" ;
this .key = "" ;
}
remove() {
window.localStorage.removeItem( this .removeKey);
this .removeKey = "" ;
}
}
|
Javascript
import { NgModule } from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { FormsModule }
from '@angular/forms' ;
import { AppRoutingModule }
from './app-routing.module' ;
import { AppComponent }
from './app.component' ;
@NgModule({
declarations: [
AppComponent,
],
imports: [
BrowserModule,
AppRoutingModule,
FormsModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
|
Output
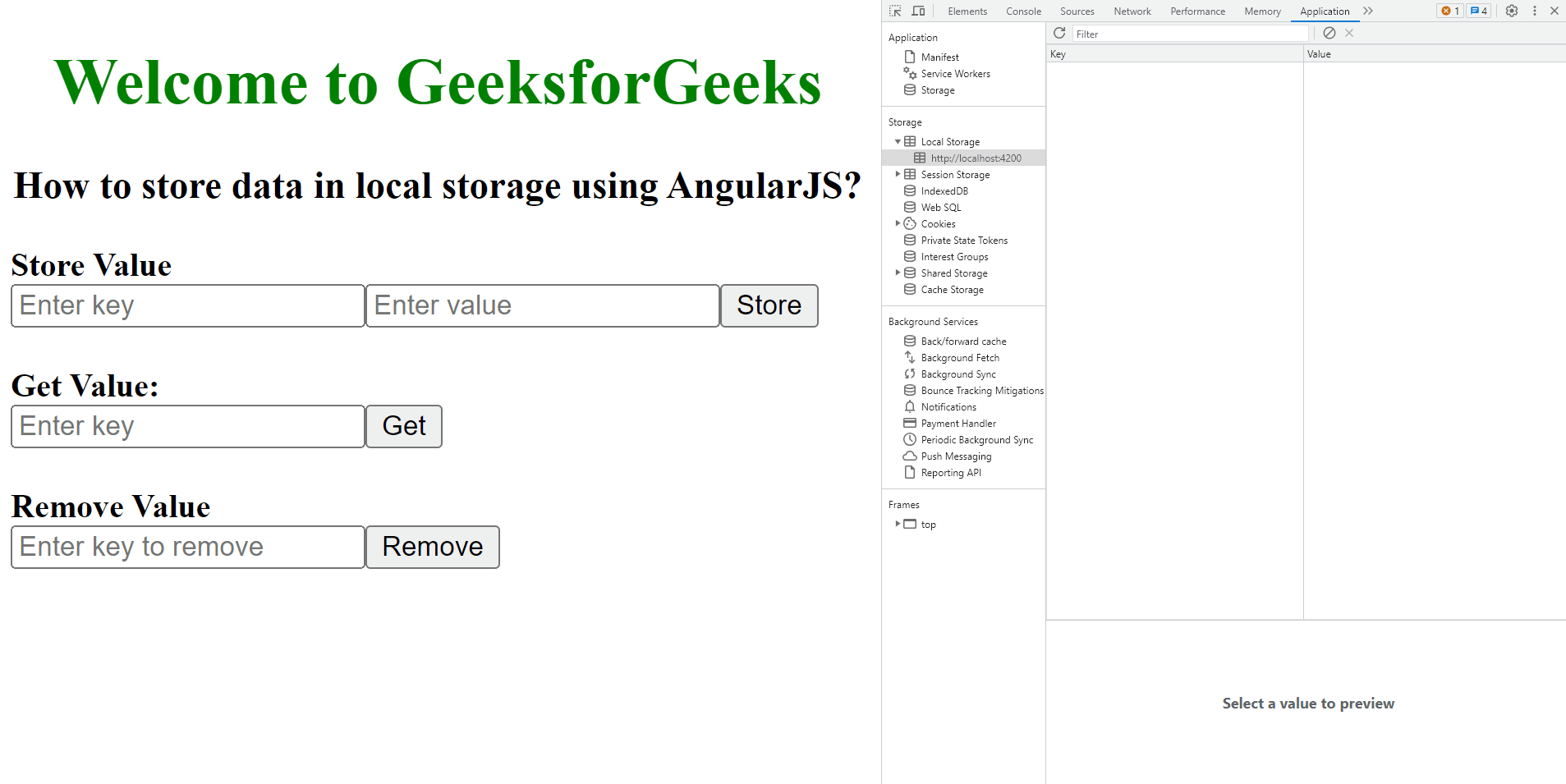
Share your thoughts in the comments
Please Login to comment...