How to remove CSS “top” and “left” attribute with jQuery ?
Last Updated :
13 Dec, 2019
Method 1: Using the css() method: The css() method is used to get or set CSS properties of a selected element. It takes two arguments, the first argument is the property that has to be set and the second argument is the value that it has to be set to.
The ‘top’ value can be effectively disabled by using the ‘initial’ value which would set the property to its default value. This is similarly done with the ‘left’ value. This will remove the effect of the ‘top’ and ‘left’ values in the element.
Syntax:
function removeTopLeft() {
$( 'selectedElement' ).css( 'top' , 'initial' );
$( 'selectedElement' ).css( 'left' , 'initial' );
}
|
Example:
<!DOCTYPE html>
< html >
< head >
< title >
How to Remove CSS "top" and
"left" attribute with jQuery?
</ title >
< style >
.box {
position: absolute;
top: 250px;
left: 200px;
height: 100px;
width: 100px;
background-color: green;
}
</ style >
< script
</ script >
</ head >
< body >
< h1 style = "color: green" >
GeeksForGeeks
</ h1 >
< b >
How to Remove CSS “top” and
“left” attribute with jQuery?
</ b >
< p >
Click on the button to remove
the top and left attribute.
</ p >
< button onclick = "removeTopLeft()" >
Remove top and left
</ button >
< div class = "box" ></ div >
< script type = "text/javascript" >
function removeTopLeft() {
$('.box').css('top', 'initial');
$('.box').css('left', 'initial');
}
</ script >
</ body >
</ html >
|
Output:
- Before clicking the button:
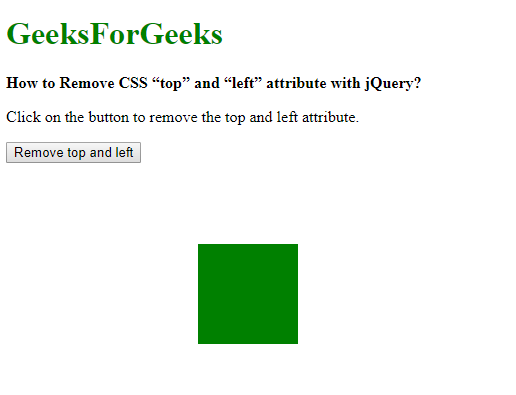
- After clicking the button:
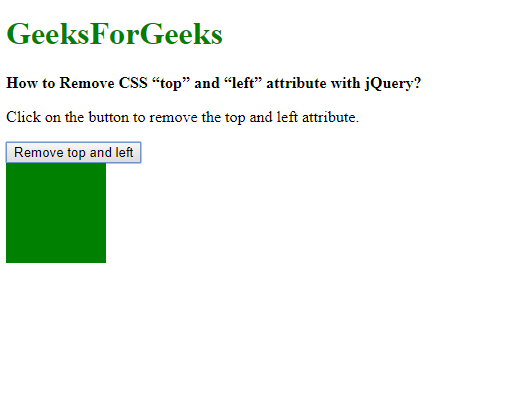
Method 2: Using the top and left properties: The top and left value can be changed by accessing these properties with the help of the style property of the element. The required element is first selected using a jQuery selector. The style property and its ‘top’ and ‘left’ values are then accessed using the dot notation on the selected object.
The ‘top’ value can be effectively disabled by using the ‘initial’ value which would set the property to its default value. It is similarly done with the ‘left’ value. This will remove the effect of the ‘top’ and ‘left’ values in the element.
Syntax:
function removeTopLeft() {
$( 'selectedElement' )[0].style.top = "initial" ;
$( 'selectedElement' )[0].style.left = "initial" ;
}
|
Example:
<!DOCTYPE html>
< html >
< head >
< title >
How to Remove CSS "top" and
"left" attribute with jQuery?
</ title >
< style >
.box {
position: absolute;
top: 250px;
left: 200px;
height: 100px;
width: 100px;
background-color: green;
}
</ style >
< script
</ script >
</ head >
< body >
< h1 style = "color: green" >
GeeksForGeeks
</ h1 >
< b >
How to Remove CSS “top” and
“left” attribute with jQuery?
</ b >
< p >
Click on the button to remove
the top and left attribute.
</ p >
< button onclick = "removeTopLeft()" >
Remove top and left
</ button >
< div class = "box" ></ div >
< script type = "text/javascript" >
function removeTopLeft() {
$('.box')[0].style.top = "initial";
$('.box')[0].style.left = "initial";
}
</ script >
</ body >
</ html >
|
Output:
- Before clicking the button:
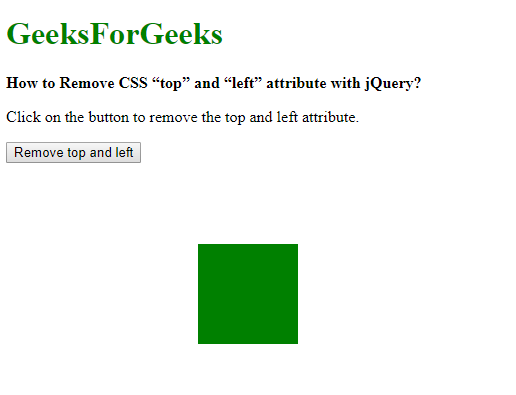
- After clicking the button:
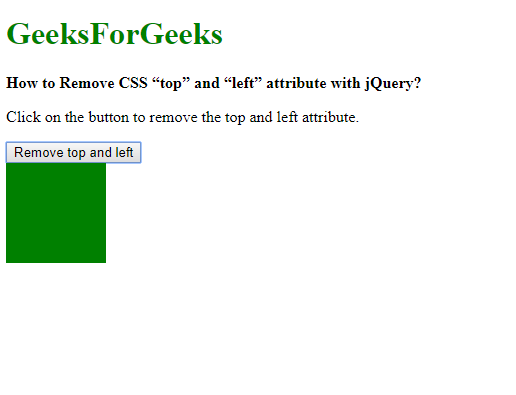
Share your thoughts in the comments
Please Login to comment...