How to Pass Parameters in Computed Properties in VueJS ?
Last Updated :
02 Jan, 2024
In this article, we are going to learn how can we pass parameters in computed properties. The difference between a computed property and a method is that computed properties are cached, Methods are functions that can be called normal JS functions, but computed properties will be “re-calculated” anytime their dependency changes in the component.
Approach
- We are going to use these for passing the parameters in computed properties:
- <template>: This is the HTML template for the Vue component. It contains a <p> tag that displays the computed value and a button that triggers the updateComputed method when clicked.
- <script>: This is the JavaScript part of the Vue component
- data(): This is a function that returns an object. The properties of this object are the reactive data for the component. In this case, baseValue is a reactive data
- computed: This is an object that contains computed properties. A computed property is a property that is computed based on other reactive data properties. In this case, computedValue is a computed property that depends on baseValue. It uses the calculateValue method to calculate its value and return
- methods: This is an object that contains methods. Methods are functions that can be called from the template. In this case, calculateValue is a method that takes a value and returns the value multiplied by 2. updateComputed is a method that updates baseValue and the computedValue computed property automatically triggers as the base value changes.
- The computedValue computed property is dependent on the baseValue data property. When baseValue changes, computedValue is automatically re-calculated.
- When you run the application (npm run dev), you’ll see the computed value displayed. Clicking the “Update Base Value” button triggers the recalculation of the computed property.
Example: This example shows the implementation of the above-explained appraoch.
HTML
< template >
< div >
< p >Computed Property: {{ computedValue }}</ p >
< button @ click = "updateComputed(10)" >
Update Value
</ button >
</ div >
</ template >
< script >
export default {
data() {
return {
baseValue: 5,
};
},
computed: {
computedValue() {
// You can't pass parameters
// directly to computed properties,
// but you can use a method that takes
// parameters to calculate the value.
return this.calculateValue(this.baseValue);
},
},
methods: {
calculateValue(value) {
// Perform computation based
// on the passed parameter
return value * 2;
},
updateComputed(newValue) {
// Update the base value triggering a
// re-calculation of computed property
this.baseValue = newValue;
},
},
};
</ script >
|
Step to run the application:
npm install
npm run dev
Output:
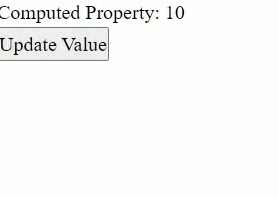
Share your thoughts in the comments
Please Login to comment...