How to Pass Variable to Inline Background Image in VueJS ?
Last Updated :
10 Jan, 2024
In this article, we will learn how we can pass variables to inline background images in VueJS. We will explore two different approaches with practical implementation in terms of examples and outputs.
Using :style Binding
In this approach, we are using the :style binding to dynamically pass the imageUrl variable to the inline background image. This makes sure that the background image is dynamically updated based on the value of the imageUrl variable.
Syntax:
<div :style="{ property1: value1, property2: value2, ... }"></div>
Example: Below is the implementation of the above-discussed approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Example 1</ title >
< script src =
</ script >
< style >
.image-container {
width: 300px;
height: 200px;
background-size: cover;
background-position: center;
margin-top: 10px;
}
.feedback-message {
color: green;
margin-top: 5px;
}
</ style >
</ head >
< body >
< div id = "app" >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
Approach 1: Using
:style Binding
</ h3 >
< label for = "imageUrl" >
Enter Image URL:
</ label >
< input v-model = "imageUrl"
type = "text" id = "imageUrl"
placeholder = "Enter Image URL" >
< button @ click = "resetImage" >
Reset to Default
</ button >
< div :style =
"{ backgroundImage: 'url(' + imageUrl + ')' }"
class = "image-container" >
</ div >
< p v-if =
"imageUrl !== defaultImageUrl"
class = "feedback-message" >
Background Image Changed!
</ p >
</ div >
< script >
new Vue({
el: '#app',
data: {
imageUrl:
defaultImageUrl:
},
methods: {
resetImage() {
this.imageUrl = this.defaultImageUrl;
},
},
});
</ script >
</ body >
</ html >
|
Output:
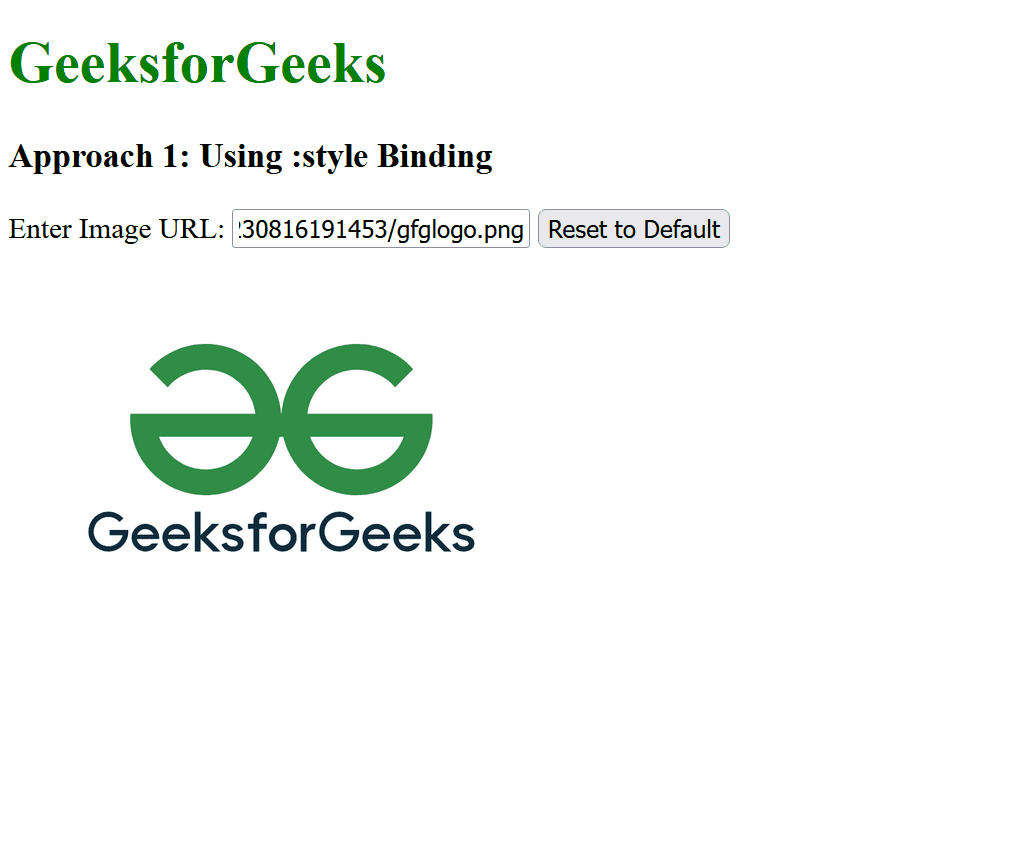
Using :class Binding
In this approach, we are using the :class binding. The background image is dynamically changed based on the user’s click. The variable selectImg is updated through the button clicks and determines which predefined background image class to apply.
Syntax:
<div :class="{ 'class-name': condition, 'another-class': anotherCondition }"></div>
Example: Below is the implementation of the above-discussed approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Example 2
</ title >
< script src =
</ script >
< style >
.background-image {
width: 300px;
height: 200px;
background-size: cover;
background-position: center;
}
#app{
display: flex;
align-items: center;
justify-content: center;
flex-direction: column;
}
.image-1 {
background-image:
}
.image-2 {
background-image:
}
.image-3 {
background-image:
}
</ style >
</ head >
< body >
< div id = "app" >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
Approach 2: Using
:class Binding
</ h3 >
< div :class="{
'background-image': true,
'image-1': selectImg === 'image-1',
'image-2': selectImg === 'image-2',
'image-3': selectImg === 'image-3'
}">
</ div >
< div >
< button @ click = "selectImage('image-1')" >
Image 1
</ button >
< button @ click = "selectImage('image-2')" >
Image 2
</ button >
< button @ click = "selectImage('image-3')" >
Image 3
</ button >
</ div >
</ div >
< script >
new Vue({
el: '#app',
data: {
selectImg: 'image-1'
},
methods: {
selectImage(image) {
this.selectImg = image;
}
}
});
</ script >
</ body >
</ html >
|
Output:
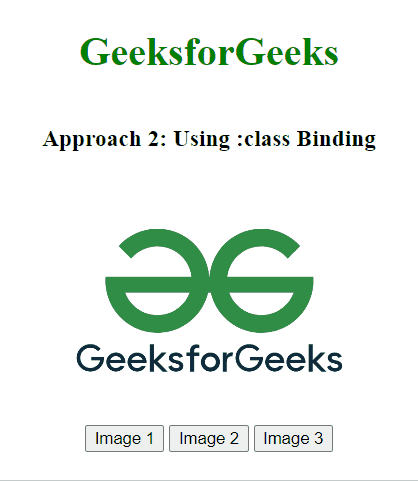
Share your thoughts in the comments
Please Login to comment...