How to navigate on path by button click in react router ?
Last Updated :
24 Nov, 2023
Navigation in React JS is done by implementing the routing between components using react-router-dom.
Prerequisites:
Approach:
In React, to navigate on the path by clicking a button we will be using the useHistory hook from react-router-dom^5 (useNavigate in v6). We will set the routes for specific components and navigate between them using a button.
Steps to Create React Application and Install Modules:
Step 1: Make a project directory, head over to the terminal, and create a react app named “ cs portal ” using the following command.
npx create-react-app cs-portal
Step 2: Move to the project directory.
cd cs-portal
Project Structure:
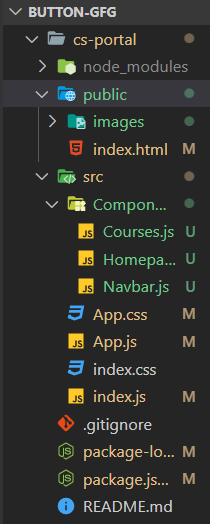
Final Project structure
The updated dependencies in package.json file.
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"bootstrap": "^4.6.2",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-router-dom": "^5.3.4",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
Example: This example demonstrates the Navigation between components in React Router when the button is clicked.
Javascript
import React from "react" ;
import {
BrowserRouter as Router,
Switch,
Route,
Link,
} from "react-router-dom" ;
import Homepage from "./Components/Homepage" ;
import Courses from "./Components/Courses" ;
import "bootstrap/dist/css/bootstrap.min.css" ;
import "./App.css" ;
import Navbar from "./Components/Navbar" ;
function App() {
return (
<>
<Navbar />
<Router>
<Switch>
<Route
exact
path= "/"
component={Homepage}
/>
<Route
exact
path= "/courses"
component={Courses}
/>
</Switch>
</Router>
</>
);
}
export default App;
|
Javascript
import React from "react" ;
function Navbar() {
return (
<div>
<nav className= "navbar navbar-light bg-light" >
<img
src=
width= "300"
height= "75"
className= "d-inline-block align-top logo"
alt= "GfG.png"
/>
GeeksforGeeks
</nav>
</div>
);
}
export default Navbar;
|
Javascript
import React from 'react'
import { useHistory } from "react-router-dom" ;
import "../App.css" ;
function Homepage() {
const history = useHistory();
const coursesPage = () => {
history.push( "/courses" )
}
return (
<>
<div className= "jumbotron text-center" >
<h1 className= "display-4" >Hello,Geeks</h1>
<p className= "lead" >
Geeks for Geeks is a Computer Science portal.
It contains well written, well thought and well
explained computer science and programming articles
</p>
<hr className= "my-4" />
<p>
Real-time Live and self paced courses carefully
curated for you !
</p>
<p className= "lead" >
<button className= "btn btn-success"
onClick={coursesPage}>Explore Courses
</button>
</p>
</div>
</>
)
}
export default Homepage
|
Javascript
import React from "react" ;
import "../App.css" ;
import { useHistory } from "react-router-dom" ;
function Courses() {
const history = useHistory();
const home = () => {
history.push( "/" );
};
return (
<>
<div className= "card " >
<h1>Courses </h1>
<ul className= "list-group list-group-flush" >
<li className= "list-group-item" >
Data Structures & Algorithms
</li>
<li className= "list-group-item" >
Competitive Programming
</li>
<li className= "list-group-item" >
Full Stack Development
</li>
<li className= "list-group-item" >
Java Backend
</li>
</ul>
</div>
<button
className= "btn btn-success"
onClick={home}
>
Back to Home
</button>
</>
);
}
export default Courses;
|
CSS
* {
text-align : center ;
}
.logo {
margin : auto ;
}
.jumbotron {
margin : 100px auto ;
max-width : 50% ;
text-align : center ;
}
.card {
width : 18 rem;
margin : 100px auto ;
}
|
Step to run the application: Now let us run our application by using the following command.
npm start
Output: By default, the React project will run on port 3000. You can access it at localhost:3000 on your browser.
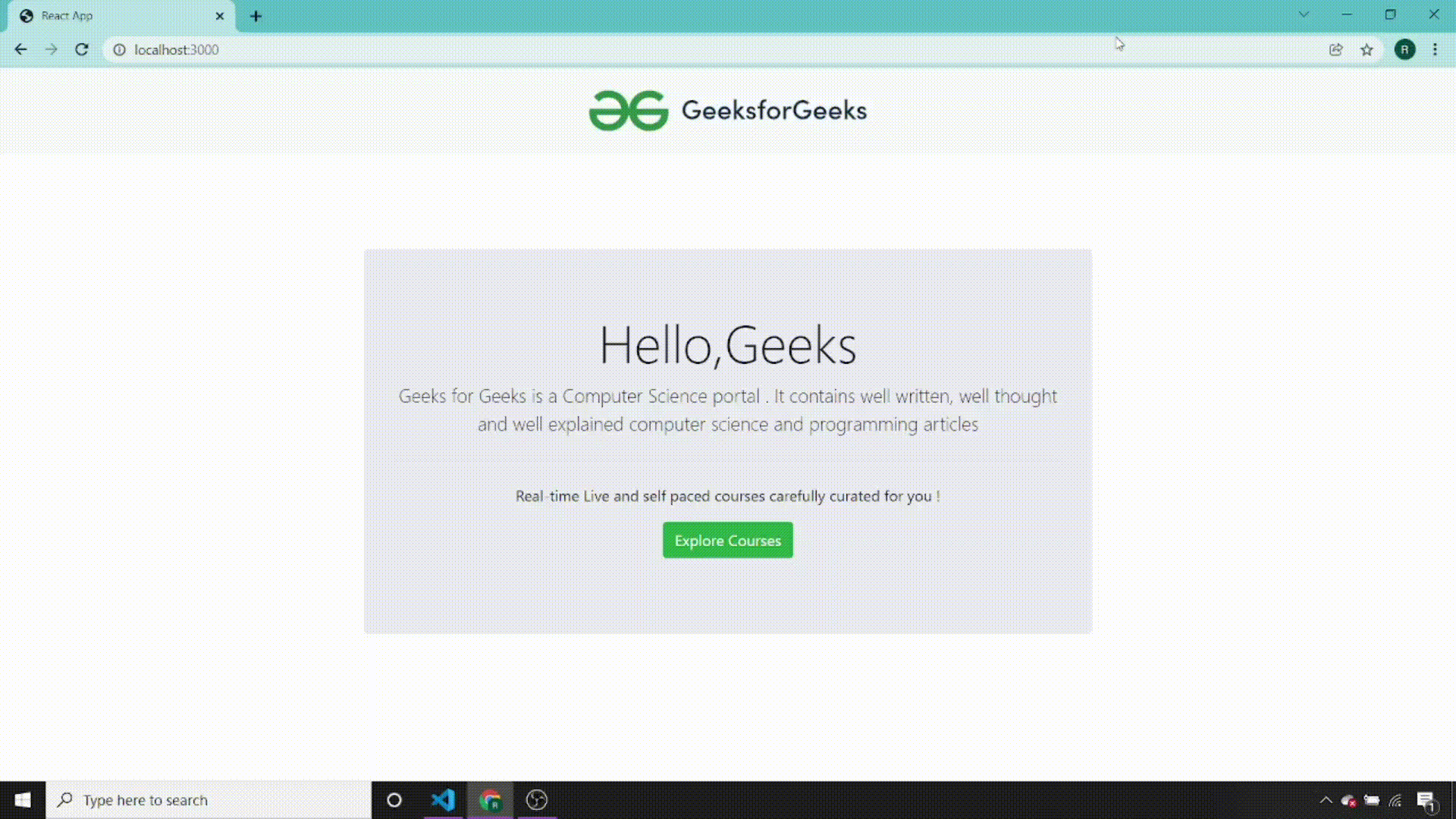
Working code output
You can watch the GeeksforGeeks video to learn more about React JS routing.
Share your thoughts in the comments
Please Login to comment...