Maximize window with selenium in C#
Last Updated :
16 May, 2024
Selenium is a popular automation tool used for controlling and automating web browsers. It provides a way for developers and testers to automate interactions with web applications. Mainly it is used to mimic how a user would interact with the application. Selenium comes up with one of the major components known as WebDriver. It is the main tool for browser automation.
It allows us to write scripts in various programming languages (such as C#, Java, Python, etc.) to automate interactions with a web browser.
Prerequisites of Maximize window with selenium in C#
- Download Chrome Driver on your Machine. You can download the driver from Click here.
- For this article, we are using the C# Console Application. So create one if not created yet.
- You also have to download some NuGet packages from NuGet Package Manager or CLI. If you are using Visual Studio 2022 then go to Tools > NuGet Package Manager > Manage NuGet Packages for Solution > Search Selenium. WebDriver > And Install.
And If you are using VS Code then open CLI and past the following command,
dotnet add package Selenium.WebDriver –version 4.12.4
Selenium capabilities
- Automate Browser Actions
- Parallel Testing
- Testing Mobile Applications
- Capture Screenshots for later Debugging
- Manage Cookies and Sessions
- Implicit and Explicit Waits
- Integrate with Testing Frameworks like NUnit and JUnit.
C#
using System;
using OpenQA.Selenium;
using OpenQA.Selenium.Chrome;
public class GFG {
static public void Main()
{
IWebDriver driver = new ChromeDriver();
// Navigate to a website
driver.Navigate().GoToUrl(
"https://www.geeksforgeeks.org");
// Maximize the browser window
driver.Manage().Window.Maximize();
// Here you can perform any action to Test the site
Console.ReadLine();
// Close the browser
driver.Quit();
}
}
Initially, we have created an instance of the WebDriver and afterward, we will apply all the actions to this instance. Here we have first navigated to the website, but it is not necessary. Then we have maximized the browser window using the Maximize() function.
If in case you want to size the window according to some width and height, then you can also do this by using the Size property.
C#
using System;
using OpenQA.Selenium;
using OpenQA.Selenium.Chrome;
public class GFG {
static public void Main()
{
IWebDriver driver = new ChromeDriver();
// Navigate to a website
driver.Navigate().GoToUrl("https://example.com");
// Maximize the browser window
driver.Manage().Window.Maximize();
// ... Perform other actions ...
Console.ReadLine();
// Close the browser
driver.Quit();
}
This will resize the window with width and height to user requirements. According to this, you can resize the browser window according to different dimensions to test the responsiveness of your site or to view the positions of alerts.
Output
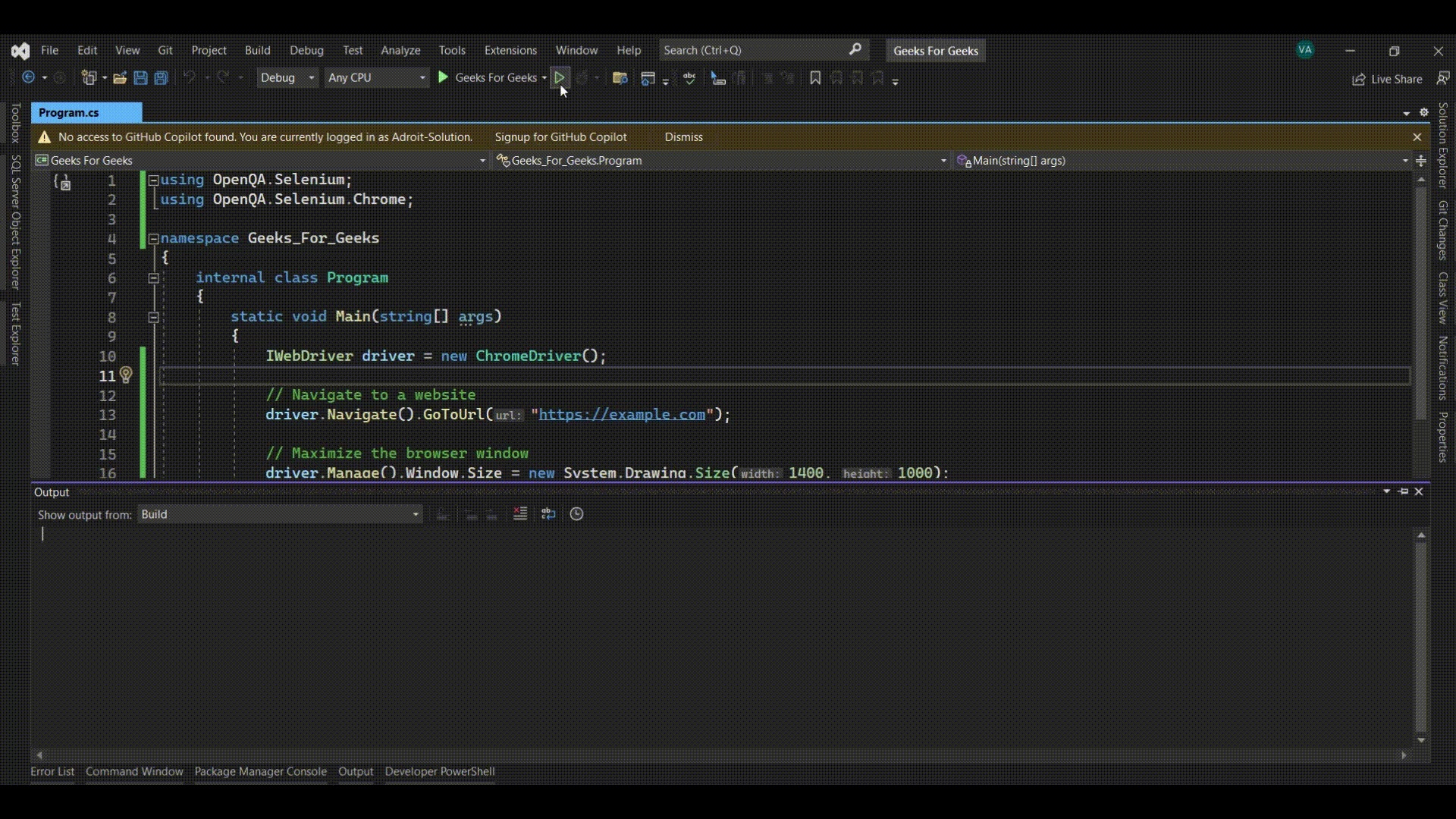
Output of maximizing the window Size
Conclusion
Thus, Selenium is very useful any many places from filling out the forms to pressing the buttons, from scrolling on a page to redirecting to different pages. You can use selenium to do anything that a user will do. You can perform operations by selecting the class name or ids. And by testing your site you can boost your UI performance. For more examples, you can view the Selenium GitHub repo.
Frequently Asked Questions on Maximize window with selenium in C#
How to maximize the window size in Selenium?
Answer:
We can maximize the browser in Selenium with the help of method which called selenium maximize().
How to make window full screen in Selenium?
Answer:
For maximize the browser in Selenium, which need to call the maximize() Selenium command to maximize window interface for the driver class.Â
How do I maximize my window size?
Answer:
press the Windows key + up arrow
Share your thoughts in the comments
Please Login to comment...