In parallel testing, multiple tests can be run simultaneously in different execution modes, reducing execution time. This approach is particularly useful when running tests across multiple browsers or operating systems because it simplifies cross-browser testing. TestNG is often used with Selenium to provide seamless integration for execution.
Using parallel behavior in the TestNG.xml configuration file, TestNG can execute independent tests in parallel, simplifying the testing process and increasing efficiency.
Parallel Testing using Selenium and TestNG
Parallel execution is a very important concept in the field of automated testing. All the work we do at the same time always saves time. Similarly, in end-to-end testing of an application, running tests in parallel instead of sequentially saves us completion time; This will save us more time in the remaining stages of the software testing lifecycle and application delivery.
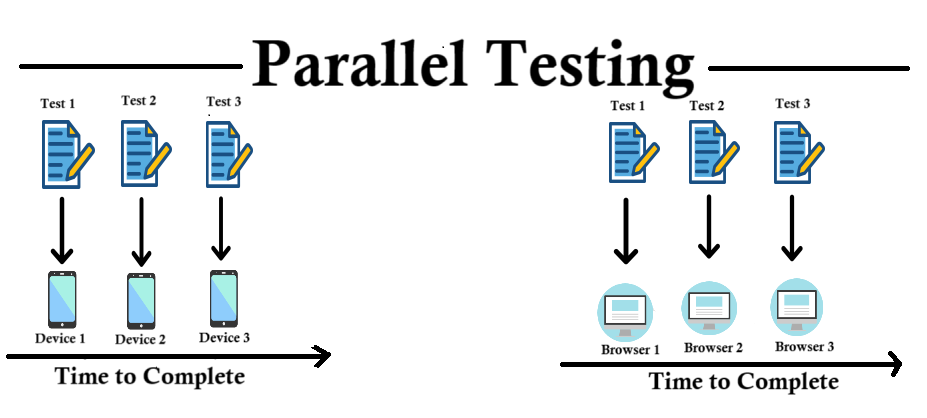
Running Parallel Test Cases in Selenium
Run parallel testing to improve our Selenium testing by running the most tests in the shortest amount of time. It helps large organizations run large-scale experiments. However, automation testers need to remember the following points before testing the same; for example, avoiding dependence of one test on another and adjusting parameters so that the test cannot be repeated successfully over several successful experiments.
Parallel tests using TestNG and Selenium are a powerful way to run multiple tests simultaneously, thereby reducing overall execution time. Here are the steps to run parallel tests using TestNG and Selenium:
Step 1: Create a Maven Project
If you are using Maven, you can create a new Maven project using the IDE or the Maven command line. Open the command line interface and navigate to the directory where you want to create the project. Then run the following command:
mvn archetype:generate -DgroupId=com.example -DartifactId=my-selenium-project -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This command will set the Maven project template.
Step 2: Add Dependencies
Open the pom.xml file in the project and add dependencies for TestNG and Selenium WebDriver. An example of how to add dependencies.
XML
<dependencies>
<!-- TestNG Dependency -->
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.4.0</version>
<scope>test</scope>
</dependency>
<!-- Selenium WebDriver Dependencies -->
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.1.0</version>
</dependency>
</dependencies>
Note: Make sure to replace the versions with the latest ones available.
Step 3: Set up a Java Project
If you are not using Maven or Gradle, you can set up a Java project by creating a new Java project and adding TestNG and Selenium WebDriver libraries to your IDE (Eclipse, IntelliJ, etc.). Structure of the workbook. You can download the JAR file from a web link or use a build engine like Apache Ivy.
Step 4: Create a Test Group
Now you can start creating a test group. You can organize your tests as ‘@Test’, ‘@BeforeTest’, ‘@AfterTest’ etc. Publish with TestNG annotations. and write Selenium WebDriver code in this process to run your tests.
Step 5: Write TestNG XML Configuration
Create a TestNG XML configuration file (‘testng.xml’) where you can define your test suites, test classes, and any parameters. Here’s a basic example.
As TestNG Test, the tests will be successful only if we follow the above rules. To run them in parallel, go to testng.xml and write the following code:
XML
<!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd" >
<suite name="Parallel Testing Suite">
<test name="Parallel Tests" parallel="methods">
<classes>
<class name="ParallelTest" />
</classes>
</test>
</suite>
Since our goal is to parallelize the process, parallel objects are assigned the “method” value.
Run the XML file as TestNG Suite and note that both drives must be opened together to prove that we are on the right track. Using the benchmarking in TestNG we can save a lot of time and solve more questions.
Step 6: Run Tests
You can now run tests from the IDE or using the TestNG command line or Maven commands. If you are using Maven, you can use the following command to run the test:
mvn test
Step7: View Results
After you complete the test, you can view the test results in the console output or in the HTML report generated by TestNG.
We will use TestNG to perform the testing procedure parallel. So when we run the test suite all the methods in the @Test annotation will run in parallel. The code below will launch the driver for two different browsers (Chrome and Firefox) simultaneously.
Java
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.chrome.*;
import org.testng.annotations.Test;
public class ParallelTest {
public WebDriver driver;
@Test
public void FirefoxTest() {
//Initializing the firefox driver (Gecko)
driver = new FirefoxDriver();
driver.get("https://www.demoqa.com");
driver.findElement(By.xpath("//*[@id=\"app\"]/div/div/div[2]/div/div[1]/div/div[1]")).click();
driver.quit();
}
@Test
public void ChromeTest()
{
//Initialize the chrome driver
driver = new ChromeDriver();
driver.get("https://www.demoqa.com");
driver.findElement(By.xpath("//*[@id=\"app\"]/div/div/div[2]/div/div[1]/div/div[1]")).click();
driver.quit();
}
}
By following these steps you will set up your project with TestNG and Selenium WebDriver dependencies, allowing you to write and execute automated tests efficiently.
Difference between Parallelization vs Serialization
Parallelization aims to improve test performance by executing tests simultaneously, while serialization ensures that tests are executed one after another. The choice of this method depends on factors such as the needs of the test, available resources, and execution speed.
Parallelization
| Serialization
|
---|
In parallelization, multiple tests run simultaneously across different environments or configurations, reducing overall test execution time.
| Serialization involves executing tests one after the other without overlapping with test execution.
|
This approach uses a testing framework such as TestNG to distribute test data across multiple threads, processes, or machines.
| Tests are executed sequentially, with each test starting only after the previous one has finished, preventing overlap between test executions.
|
Parallelization can reduce execution times, especially for high-pressure tests or cross-browser tests.
| Serialization is easier but potentially slower, especially for many tests or long tests.
|
The use and control of parallelism will include monitoring parallel work and ensuring parallelism of tests.
| Serialization is easier to implement and manage because tests run without having to deal with compatibility issues.
|
Parallelization works well in situations where efficiency is required, such as managing multiple tests or running cross-browser tests.
| For simpler tests or where specifying the application is important, serialization may be the preferred method. This option is important for the ease and speed of management, it speeds up the process for better management.
|
Serialization refers to the process of executing operations one by one in a thread of execution. In short, tasks are performed sequentially and each task starts only after the completion of the previous task.
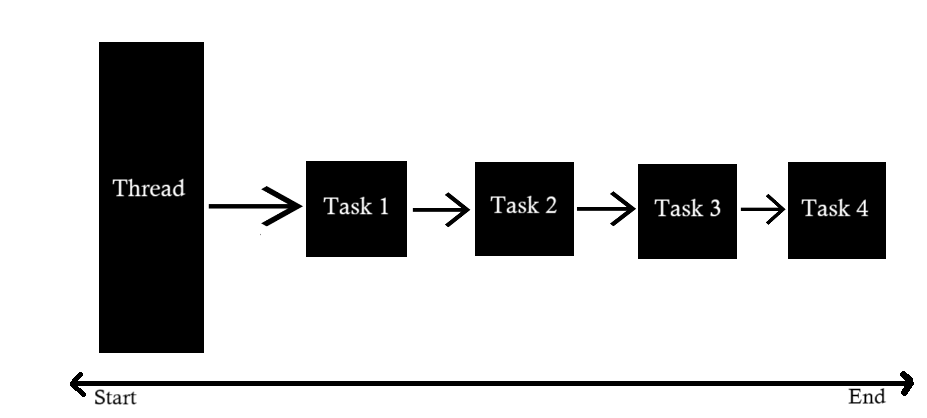
Serialization
Parallelization means executing a series of tasks on multiple threads, where one task is assigned to one thread. So these tasks are done together. This approach makes better use of multiple CPU cores and can improve application performance and performance.
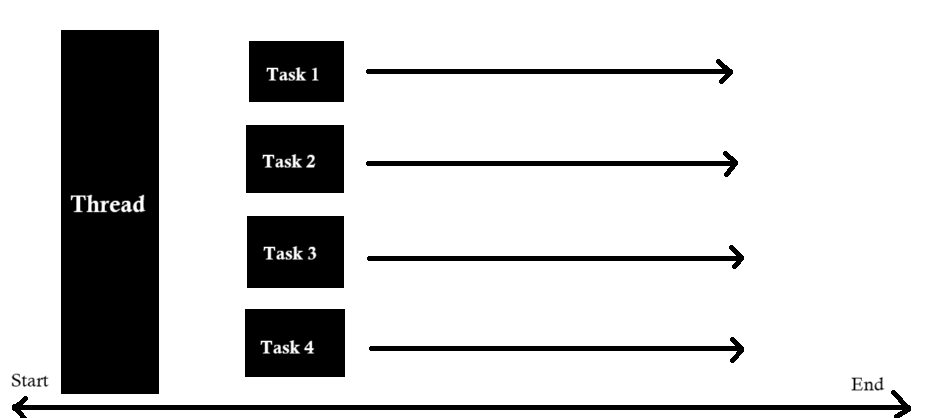
Parallelization
Time Taken Report of Parallelization vs Serialization
Consider the case where we have code with two test methods. This process will run simultaneously in two different browsers: one test will run in Chrome and the other test will run in Firefox. This setup illustrates how parallelization enhances testing speed and provide a better return on investment (ROI).
In the example, we will do this step by step, one after the other. This approach contrasts with other examples where two methods work in parallel, creating two processes that can be done simultaneously. The image below shows the times required for the two methods, respectively:
1. Time Taken Report of Parallelization
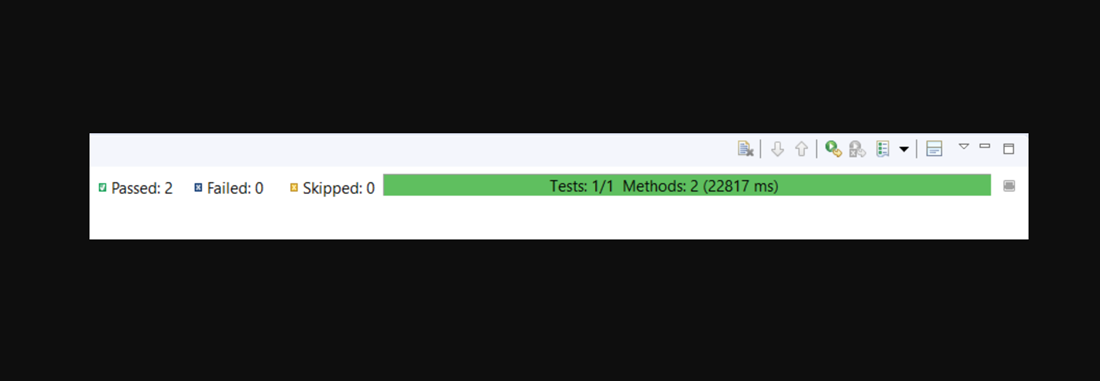
Time Taken report of Parallelization
2. Time Taken Report of Serialization
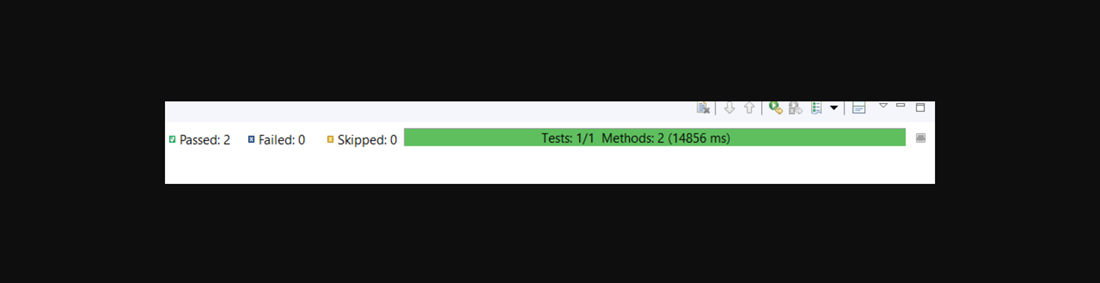
Time Taken report of Serialization
Both image indicates the time taken to execute the methods in parallel.
Advantages of Parallel Testing
- Reduced Execution Time: Parallel testing reduces total test execution time by running multiple tests simultaneously, making it especially useful for large test runs or multi-type setups.
- Allow Multi-Threaded Tests: TestNG’s parallel execution enables simultaneous running of multiple threads on test cases, fostering independence in executing different software components.
- Detect Issue: Parallel testing makes it easier to find the problem faster by providing rapid feedback on usage behavior in various situations, enabling timely solutions and reducing project time and associated costs.
Disadvantages of Parallel Testing
- Dependent module failed: Parallel testing allows independent modules to be run simultaneously, but often results in failure when modules are interdependent. This problem often arises during experiments that must be completed or sacrificed for independence, both of which require more time and effort.
- Debugging Complexity: Debugging parallel tests can be challenging due to concurrent execution, requiring more effort and time to pinpoint the root cause of failures or unexpected behavior.
- Program flow information: To create a balanced testing model, testers need to have a deep understanding of the flow. Even the slightest interdependence can destroy the entire successful experiment. Testers need to know which modules must run simultaneously and which modules must follow a pattern to ensure proper operation.
Conclusion
We have probably mentioned all the scenarios that a software tester must handle during the testing phase of parallel testing. Integrated testing is possible when cross-browser testing is required; This requires installing Selenium Grid or accessing a cloud-based platform that provides you with Selenium Grid to run automated tests in parallel or in conjunction with different configurations. Integrated testing not only saves testers’ time, but also speeds up the delivery process following good results of verification and validation during testing. Try to improve your automated tests.
FAQs
Q.1 What is Parallel Testing in Selenium?
Ans: Parallel testing speeds up the testing process by testing multiple applications or devices simultaneously. In parallel testing, different parts of the test look at different parts or features of the application. This product works on different computers simultaneously.
Q.2 What is parallel execution in Selenium?
Ans: Parallel testing allows methods to be run on different execution threads. It helps control scalability by running all methods in the same thread with the same code, and for classes it ensures that all methods in the class run in a single thread. Also, using its instance, one can run all methods on the same instance and thread.
Q.3 Can we do parallel testing in Selenium?
Ans: Yes, you can run parallel tests in Selenium. Selenium itself does not support parallel testing, but you can implement parallel testing by integrating Selenium with testing frameworks such as TestNG or JUnit that provide full execution capabilities.
Q.4 How can I test Selenium WebDriver in parallel across multiple browsers?
Ans: Selenium WebDriver and the TestNG framework can be combined to run test files in multiple browsers simultaneously on the same computer. Since there are two test tabs in this example (“ChromeTest” and “FirefoxTest”), the test file will be run twice, once for each browser.
Share your thoughts in the comments
Please Login to comment...