How to make Vue v-on:click work on Component in VueJS ?
Last Updated :
23 Apr, 2024
In Vue.js, the v-on directive is used to attach event listeners to the DOM elements and components. However, when working with the components, especially custom components may encounter situations where the v-on:click directive does not work as expected.
The below approaches can be used to make it work:
Using Native Events
In this approach, we utilize native DOM events to handle click events on Vue components. We can directly attach event listeners to the component’s root element using standard JavaScript.
Syntax:
<div @click="handleClick">
<!-- Component content -->
</div>
Example: The below code utilizes the native click event on the element in the DOM.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
The Vue v-on:click Example
</title>
<script src=
"https://cdn.jsdelivr.net/npm/vue@2">
</script>
</head>
<body style="text-align: center;">
<div id="app">
<h2>
Click the below button to
<br />open the alert box.
</h2>
<button @click="handleClick"
style="cursor: pointer;">
Click me!
</button>
</div>
<script>
new Vue({
el: '#app',
methods: {
handleClick() {
alert('Clicked!');
}
}
});
</script>
</body>
</html>
Output:
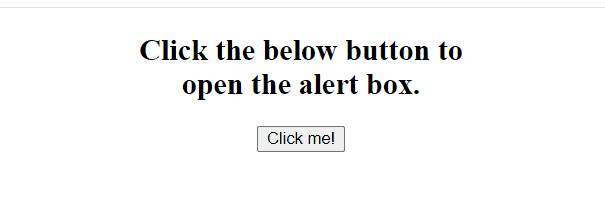
Using Child Components
In this approach, we emit custom events from the child component and handle them in the parent component or Vue instance. This method provides more flexibility and encapsulation.
Syntax:
<template>
<div @click="$emit('custom-click')">
<!-- Component content -->
</div>
</template>
Example: The below code implements the child component with emit() method to make click event work.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible"
content="IE=edge">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
Vue v-on:click Example
</title>
<script src=
"https://cdn.jsdelivr.net/npm/vue@2.6.14/dist/vue.js">
</script>
</head>
<body style="text-align: center;">
<div id="app">
<h2>
Click the below button to
<br />open the alert box.
</h2>
<custom-button @button-click="handleClick" />
</div>
<script>
Vue.component('custom-button', {
template: `
<button @click="childClick"
style="cursor: pointer;">
Click me!
</button>
`,
methods: {
childClick() {
this.$emit('button-click')
}
}
});
new Vue({
el: '#app',
methods: {
handleClick() {
alert('Clicked!');
}
}
});
</script>
</body>
</html>
Output:
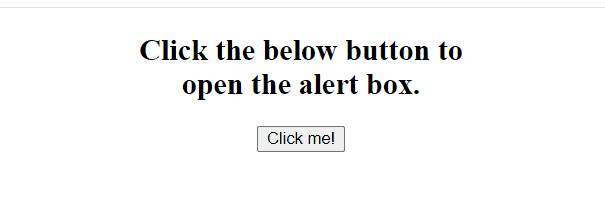
Share your thoughts in the comments
Please Login to comment...