How to Increase Button Size in HTML Without CSS ?
Last Updated :
08 Mar, 2024
To increase the button size in HTML without CSS, one can use JQuery and JavaScript language. In this article, we will explore both of these approaches with complete implementation in terms of examples and outputs.
Using jQuery
In this approach, we are using jQuery to dynamically adjust the width and height of the button based on user input from the number field, allowing the button size to change in real time without using any CSS or style properties.
Syntax:
$(function(){
// jQuery Method
});
Example: To demonstrate increasing button size in html without CSS using jQuery.
HTML
<!DOCTYPE html>
< head >
< title >Example 2</ title >
< script src =
< style >
body {
text-align: center;
margin: 50px;
}
</ style >
</ head >
< body >
< h1 style = "color: green;" >GeeksforGeeks</ h1 >
< h3 >Using jQuery</ h3 >
< div >
< label for = "sizeInput" >Enter size:</ label >
< input type = "number"
id = "sizeInput" min = "10"
max = "200" step = "5" value = "50" >
</ div >
< div >
< br />
< button id = "changeSizeButton" >Change Size</ button >
</ div >
< script >
$(document).ready(function() {
$('#sizeInput').on('input', function() {
var newSize = $(this).val();
$('#changeSizeButton').width(newSize).height(newSize);
});
});
</ script >
</ body >
</ html >
|
Output:
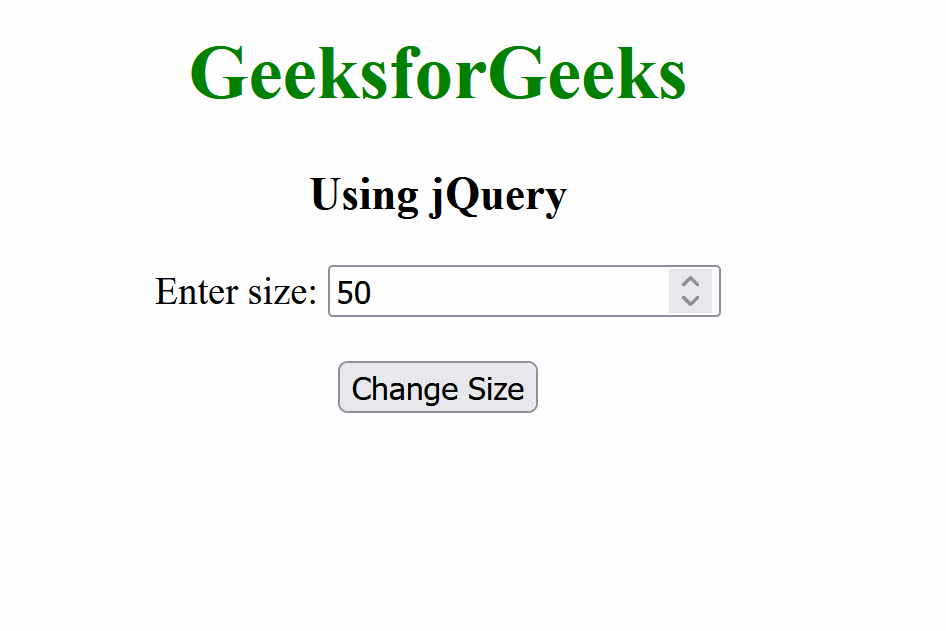
Using JavaScript
In this approach, we are using JavaScript to dynamically increase the button size without using CSS. The increaseFn() function, is triggered by the button click, retrieves the current width and height of the button and increments them by 20 pixels and 10 pixels.
Syntax:
<script>
<!-- javascript body -->
</script>
Example: The below example uses JavaScript to increase button size in html without CSS.
HTML
<!DOCTYPE html>
< head >
< title >Example 2</ title >
</ head >
< body >
< h1 style = "color: green;" >GeeksforGeeks</ h1 >
< h3 >Using JavaScript</ h3 >
< button type = "button" id = "myButton"
onclick = "increaseFn()" >Click to Increase Size</ button >
< script >
function increaseFn() {
var btn = document.getElementById("myButton");
var currwidth = btn.clientWidth;
var currheight = btn.clientHeight;
btn.style.width = (currwidth + 20) + "px";
btn.style.height = (currheight + 10) + "px";
}
</ script >
</ body >
</ html >
|
Output:
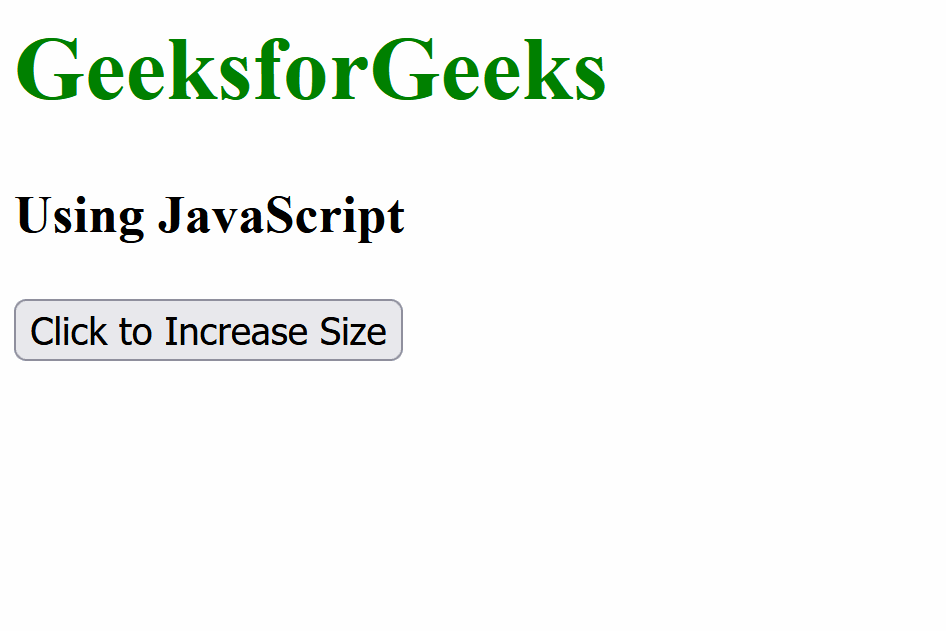
Share your thoughts in the comments
Please Login to comment...