How to use CDN Links in React JS?
Last Updated :
28 Nov, 2023
React is integrated in the projects using package managers like npm or yarn. However, there are some cases where using Content Delivery Network (CDN) links can be a advantage, especially for quick prototypes, or when integrating React into existing projects.
The two libraries imported CDN Links are discussed below
Prerequisites
In this article, we are going to learn how we can use CDN links in React JS. CDN links stand for Content Delivery Network Links. These links are used to connect CSS frameworks or scripts; basically, already-written code can be connected through these links.
Steps to Create Project
Step 1: Create a React App via following command.
npx create-react-app name
Step 2: Move to root directory.
cd name
Project Structure
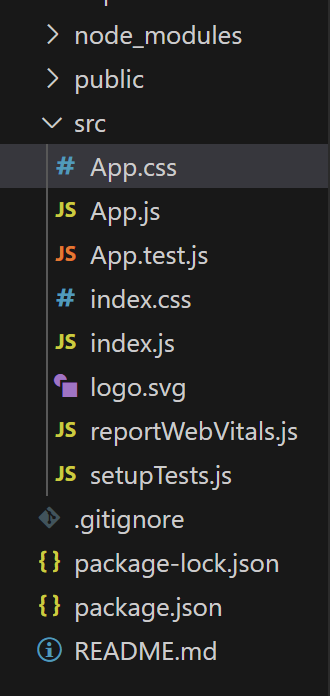
Approach 1: Importing Bootstrap using CDN
Bootstrap is a CSS-based framework that is used to connect Bootstrap CSS using CDN (Content Delivery Network). We can connect it through CDN. The CDN link is always added to the public folder where the index.html file is present.
CDN Link:
<script src=”https://cdn.jsdelivr.net/npm/bootstrap@5.3.2/dist/js/bootstrap.bundle.min.js” integrity= “sha384-C6RzsynM9kWDrMNeT87bh95OGNyZPhcTNXj1NW7RuBCsyN/o0jlpcV8Qyq46cDfL” crossorigin=”anonymous”></script>
Example: Implementation of above approach.
Javascript
import './App.css' ;
function App() {
return (
<h1 className= "text-center bg-primary p-4" >GeeksforGeeks</h1>
)
}
export default App;
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" />
< link rel = "icon" href = "%PUBLIC_URL%/favicon.ico" />
< meta name = "viewport" content = "width=device-width, initial-scale=1" />
< meta name = "theme-color" content = "#000000" />
< meta name = "description" content = "Web site created using create-react-app" />
< link rel = "apple-touch-icon" href = "%PUBLIC_URL%/logo192.png" />
< link rel = "manifest"
href = "%PUBLIC_URL%/manifest.json" />
< link href =
rel = "stylesheet"
integrity =
"sha384-T3c6CoIi6uLrA9TneNEoa7RxnatzjcDSCmG1MXxSR1GAsXEV/Dwwykc2MPK8M2HN"
crossorigin = "anonymous" >
< script src =
integrity =
"sha384-C6RzsynM9kWDrMNeT87bh95OGNyZPhcTNXj1NW7RuBCsyN/o0jlpcV8Qyq46cDfL"
crossorigin = "anonymous" ></ script >
< title >React App</ title >
</ head >
< body >
< noscript >You need to enable JavaScript to run this app.</ noscript >
< div id = "root" ></ div >
</ body >
</ html >
|
Output:

Approach 2: Importing Tailwind using CDN
Tailwind CSS is also a framework of CSS that is used to implement CSS. Instead of writing CSS, we can directly apply class names to the React component. Tailwind provides a CDN (Content Delivery Network) link through which we can connect Tailwind CSS to our program.
CDN Link:
<script src=”https://cdn.tailwindcss.com”></script>
Example: Implementation of above Tailwind approach.
Javascript
import './App.css' ;
function App() {
return (
<h1 className= "text-center bg-primary p-4 text-white" >GeeksforGeeks</h1>
)
}
export default App;
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "utf-8" />
< link rel = "icon" href = "%PUBLIC_URL%/favicon.ico" />
< meta name = "viewport" content = "width=device-width, initial-scale=1" />
< meta name = "theme-color" content = "#000000" />
< meta name = "description" content = "Web site created using create-react-app" />
< link rel = "apple-touch-icon" href = "%PUBLIC_URL%/logo192.png" />
< link rel = "manifest" href = "%PUBLIC_URL%/manifest.json" />
< title >React App</ title >
</ head >
< body >
< noscript >You need to enable JavaScript to run this app.</ noscript >
< div id = "root" ></ div >
</ body >
</ html >
|
Output:

Conclusion
Using CDN links in React gives quick and convenient way to integrate React into your projects without the need for a package manager. It’s mostly useful for small projects, or when creating quick prototyping. However, for production-grade applications, consider using package managers for better dependency management and version control.
Share your thoughts in the comments
Please Login to comment...