How to hide “Image not found” icon when source image is not found?
Last Updated :
17 Aug, 2021
JavaScript and jQuery can be used to hide the “Image Not Found”icon when the image is not found. The basic idea is to set the display property of the image object to ‘hidden’ when an error arises. Let us consider the below HTML code which displays the GeeksforGeeks logo for demonstration purpose.
Example: In this example, we will not hide the icon of image not found.
html
<!DOCTYPE html>
< html >
< head >
</ head >
< body >
< h2 >
Example to hide the 'Image Not Found' icon i.e.
< img src = "error.png" width = "20px" />
</ h2 >
< img id = "HideImg" src = "geeksforgeeks-6.png" />
</ body >
</ html >
|
Output:
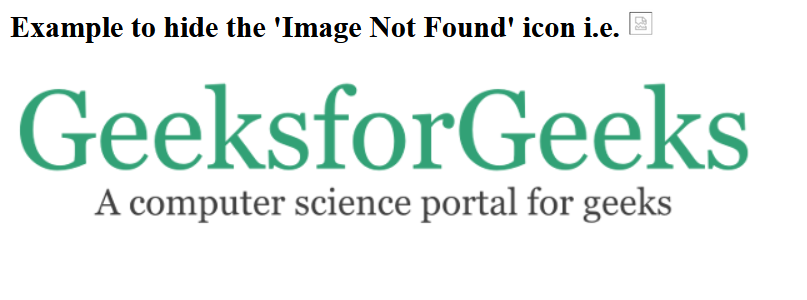
- When the image is not found:
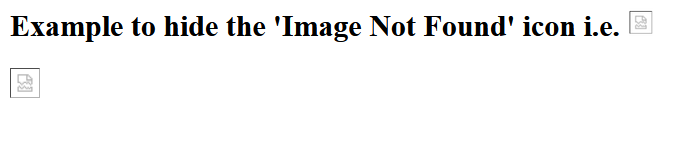
Methods to hide the image error icon:
- Using onerror() event: The onerror event is automatically called when the corresponding item raises an error. When the image is not found, the onerror event while call the hideImg() function which will set the visibility of the image to hidden.
Program:
html
<!DOCTYPE html>
< html >
< head >
</ head >
< body >
< h2 >
Example to hide the 'Image Not Found' icon i.e.
< img src = "error.png" width = "20px" />
</ h2 >
< img id = "HideImg" src = "geeksforgeeks-6.png"
onerror = "hideImg()" />
< script >
function hideImg() {
document.getElementById("HideImg")
.style.display = "none";
}
</ script >
</ body >
</ html >
|
-
- Using short-hand notation: You can apply this short-hand notation for HTML events. This will set the visibility of the reference object to hidden.
Program:
html
< html >
< head >
</ head >
< body >
< h2 >
Example to hide the 'Image Not Found' icon i.e.
< img src = "error.png" width = "20px" />
</ h2 >
< img id = "HideImg" src = "geeksforgeeks-6.png"
onerror = 'this.style.display = "none"' />
</ body >
</ html >
|
- Using jQuery: Using the JQuery error handler function error(), we can catch the error and hide the object using hide() function.
Note: The error() function has been removed from jquery version 3.0. and later.
Program:
html
<!DOCTYPE html>
< html >
< head >
< script src =
</ script >
</ head >
< body >
< h2 >
Example to hide the 'Image Not Found' icon i.e.
< img src = "error.png" width = "20px" />
</ h2 >
< img id = "HideImg" src = "geeksforgeeks-6.png" />
</ body >
</ html >
< script >
$(document).ready(function () {
$("#HideImg").error(function () {
$(this).hide();
});
});
</ script >
|
Output: All the above codes give the same output.
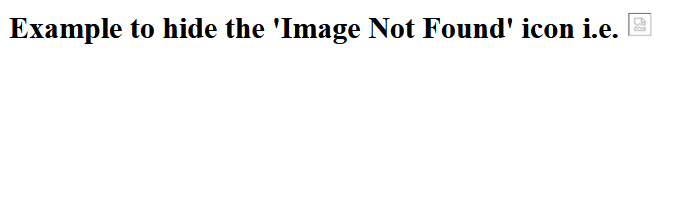
Share your thoughts in the comments
Please Login to comment...