How to Hide Audio Controls in HTML5 ?
Last Updated :
21 Feb, 2024
In HTML5, the <audio> element embeds audio content into web pages. By default, it comes with built-in controls like play, pause, and volume adjustment. However, there might be scenarios where you want to hide these controls for a cleaner or more customized user experience. This article explores various approaches to achieve this in HTML5.
Using the controls Attribute
The controls attribute in the <audio> element specifies that the browser should provide controls for playing the audio. By removing this attribute, we can hide the default audio controls.
Syntax:
<audio src="audio.mp3" controls>
Your browser does not support the audio element.
</audio>
Example: Illustration of hiding Audio controls in HTML5 Using the controls Attribute
HTML
<!DOCTYPE html>
< html >
< head >
< title >Hide Audio Controls using
'controls' attribute
</ title >
</ head >
< body >
< h1 >Audio Player</ h1 >
< p >Audio with Controls:</ p >
< audio controls src = "audiosample.mp3" >
Your browser does not support the audio element.
</ audio >
< p >Audio without Controls:</ p >
< audio src = "audiosample.mp3" >
Your browser does not support the audio element.
</ audio >
</ body >
</ html >
|
Output:
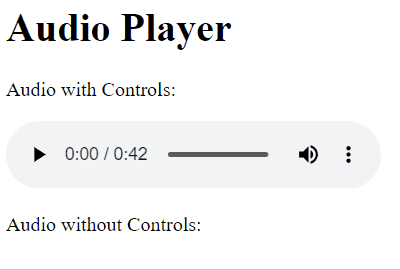
Using CSS to Hide Controls
We can utilize CSS to hide the default audio controls by setting the display property to none for the audio element.
Syntax:
<style>
audio::-webkit-media-controls {
display: none;
}
</style>
Example: Illustration of hiding Audio controls in HTML5 using CSS to Hide Controls
HTML
<!DOCTYPE html>
< html >
< head >
< title >Hide Audio Controls using CSS</ title >
< style >
audio::-webkit-media-controls {
display: none;
}
</ style >
</ head >
< body >
< h1 >Audio Player</ h1 >
< audio src = "audio.mp3" controls>
Your browser does not support the audio element.
</ audio >
</ body >
</ html >
|
Output:
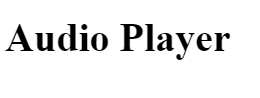
Using JavaScript to Dynamically Hide Controls
JavaScript can be used to dynamically remove the controls attribute from the <audio> element, effectively hiding the default controls. The JavaScript function toggles visibility of audio controls by adding or removing the “controls” attribute based on its presence. It targets the audio element with ID “myAudio”, enabling users to dynamically show or hide controls. The HTML includes a button for user interaction, invoking the function, and basic styling for visual appeal.
Syntax:
document.addEventListener('DOMContentLoaded', function() {
var audio = document.querySelector('audio');
audio.removeAttribute('controls');
});
Example: Illustration of hiding Audio controls in HTML5 using JavaScript to Dynamically Hide Controls
HTML
<!DOCTYPE html>
< html >
< head >
< title >Hide Audio Controls using 'controls' attribute</ title >
< script >
// Function to toggle audio controls
function toggleAudioControls() {
var audioElement = document.getElementById("myAudio");
if (audioElement.hasAttribute("controls")) {
// Remove controls
audioElement.removeAttribute("controls");
} else {
// Add controls
audioElement.setAttribute("controls", "controls");
}
}
</ script >
</ head >
< body >
< h1 >Audio Player</ h1 >
< audio id = "myAudio" controls src = "audiosample.mp3" >
Your browser does not support the audio element.
</ audio >
< br >
< button onclick = "toggleAudioControls()"
style="margin: 10px; border-radius: 10px;
padding: 10px;">
Toggle Audio Controls
</ button >
</ body >
</ html >
|
Output:
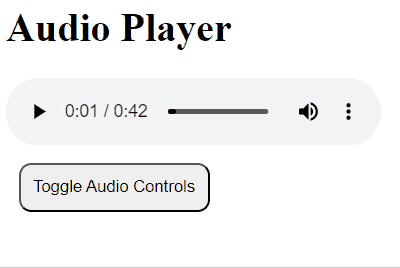
Share your thoughts in the comments
Please Login to comment...