Difference between sessions and cookies in Express
Last Updated :
27 Dec, 2023
Express.js is a popular framework for Node.js, that is used to create web applications. It provides tools to manage user sessions and cookies. The session and cookies are used to maintain the state and manage user authentication. In this article, we will learn about what sessions and cookies in Express and their differences.
Cookies in Express:
Cookies are small pieces of data that are stored on the client side (browser) in the form of a key-value pair. Cookies are used for session management, user preference,a and tracking of user behavior. when user loads the website a cookie is sent with the request that helps us to track the user’s actions.
To use cookies in Express, you have to install the cookie-parser package, It is a middleware that is used to parse cookies from the incoming request.
npm install cookie-parser
Example:
Javascript
const express = require( "express" );
const cookieParser = require( "cookie-parser" );
const app = express();
app.use(cookieParser());
app.get( "/setCookie" , (req, res) => {
res.cookie( "username" , "GeeksForGeeks" );
res.send( "Cookies set successfully!" );
});
app.get( "/getCookie" , (req, res) => {
const username = req.cookies.username;
res.send(`Username: ${username}`);
});
app.get( "/clearCookie" , (req, res) => {
res.clearCookie( "username" );
res.send( "Cookie deleted successfully!" );
});
app.listen(3000, () => {
console.log( "Server is running on port 3000" );
});
|
To Run the Application, Type the following command in terminal:
node index.js
Output
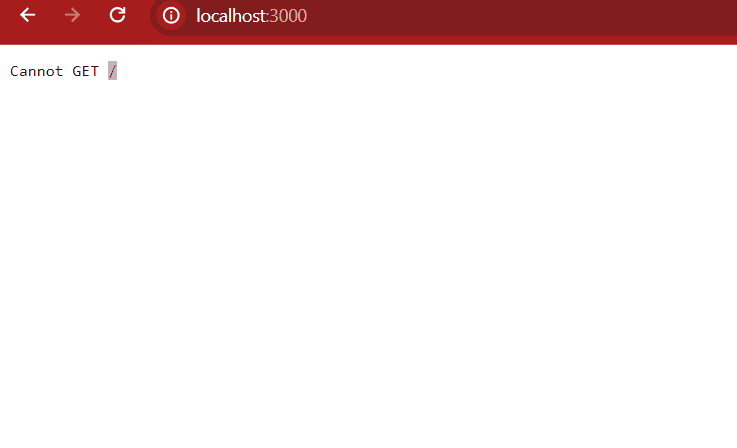
cookies example output
Session in Express:
A session is a feature in Express that let you maintaining state and user-specific data across multiple requests. sessions stores information at a server side with a unique session identifier. In a session you assign a unique session id to the client. After that client makes all request to the server with that unique id.
To use session in a Express, you have to install express-session package, It is a middleware that is used to provides a simple API for creating, reading, and updating session data.
npm install express-session
Example:
Javascript
const express = require( 'express' );
const session = require( 'express-session' );
const app = express();
app.use(session({
secret: 'secret_key' ,
resave: false ,
saveUninitialized: true ,
}));
app.get( '/setSession' , (req, res) => {
req.session.username = 'GeeksForGeeks' ;
res.send( 'Session set successfully!' );
});
app.get( '/getSession' , (req, res) => {
const username = req.session.username;
res.send(`Username from session: ${username}`);
});
app.get( '/destroySession' , (req, res) => {
req.session.destroy((err) => {
if (err) {
console.error(err);
} else {
res.send( 'Session destroyed successfully!' );
}
});
});
app.listen(3000, () => {
console.log( 'Server is running on port 3000' );
});
|
To Run the Application, Type the following command in terminal:
node index.js
Output
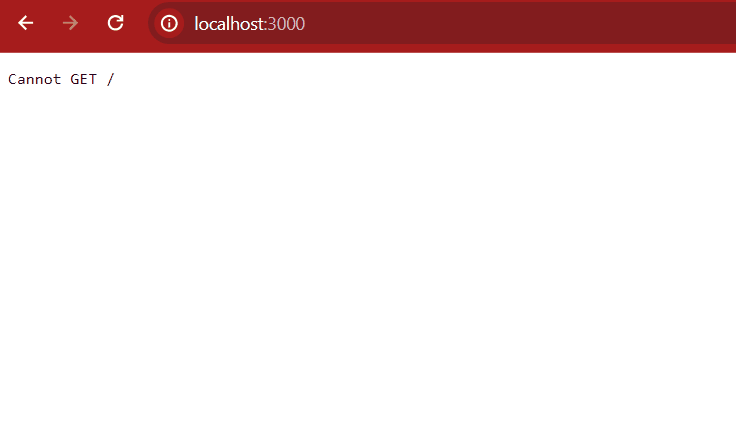
sessions example output
Difference between Session and Cookies in Express
A session is stored at server side
|
A cookie is stored at client side
|
It can store a data ranging between 5mb – 10mb
|
It can only store a data of 4kb
|
It is destroyed when user logout.
|
It is destroyed when user closes the page or it will remain until the defined time.
|
express-session middleware is required to create a session.
|
It doesn’t require any middleware to create a cookie. Express provide built in support.
|
Session id is used as a identifier.
|
Key-value pair data is used as a identifier.
|
The performance of session is slower due to server interaction.
|
The performance of cookie is faster, as data is stored locally.
|
It is more secure as data are stored at server side.
|
It is less secure as data are stored at client side.
|
It is used for storing user-specific data.
|
It is used to store user preference data.
|
Share your thoughts in the comments
Please Login to comment...