How to Handle Route Parameters in Angular?
Last Updated :
03 May, 2024
In Angular, routing plays an important role in building single-page applications (SPAs). Often, you need to pass data between components through the URL using route parameters. Route parameters allow you to define dynamic segments in your routes, which can be extracted and used in your components. This article will explore how to handle route parameters in Angular and demonstrate their usage.
Prerequisites
What are Route Parameters?
Route parameters are dynamic values in the URL that can be used to identify specific data or resources within your application. They are defined in the route configuration of your Angular application. They are specified within the route path by enclosing them in colons (:
). Let us consider an example Route parameters are defined in the route configuration of your Angular application.
const routes: Routes = [
{ path: 'product/:id', component: ProductDetailComponent }
];
Steps to Handle Route Parameters
Step 1: Create angular application
We can use below angular cli commands to create a new application.
ng new route-parameter
cd route-parameter
This will create a new directory route-parameter and change the current directory to route-parameter.
Step 2: Creating product component
This component will have list of available products, you can think of it as the amazon homepage. Again, by using angular cli we can create product component.
ng g c product
Step 3: Create product detail component
Once we have product component, we will also create a product detail component where we can show a specific product in detail.
ng g c product/product-detail
Folder Structure
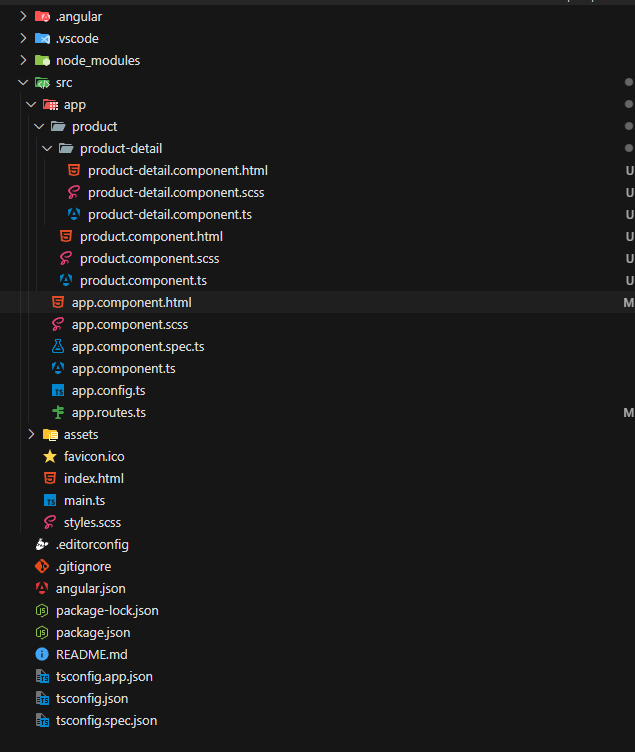
Dependencies
"dependencies": {
"@angular/animations": "^17.3.0",
"@angular/common": "^17.3.0",
"@angular/compiler": "^17.3.0",
"@angular/core": "^17.3.0",
"@angular/forms": "^17.3.0",
"@angular/platform-browser": "^17.3.0",
"@angular/platform-browser-dynamic": "^17.3.0",
"@angular/router": "^17.3.0",
"rxjs": "~7.8.0",
"tslib": "^2.3.0",
"zone.js": "~0.14.3"
},
Example
HTML
<!-- product.component.html -->
<div class="container">
@for (product of products; track $index) {
<div class="product" [routerLink]="['/product', product.id]">
<p class="name">
{{ product.name }}
</p>
<p class="description">
{{ product.description }}
</p>
</div>
}
</div>
HTML
<!-- product-detail.component.html -->
@if (productId) {
<p>
Showing product with id: {{ productId }}
</p>
<button routerLink="/product">
Go back
</button>
}
HTML
<!-- app.component.html -->
<router-outlet></router-outlet>
CSS
/* product.component.scss */
.container {
max-width: 800px;
margin: 64px auto;
padding: 16px;
display: flex;
flex-direction: row;
justify-content: space-between;
gap: 16px;
.product {
border: 1px solid #ccc;
padding: 16px;
&:hover {
border-color: #333;
cursor: pointer;
}
.name {
font-size: 24px;
font-weight: bold;
}
.description {
font-size: 16px;
margin-top: 8px;
}
}
}
JavaScript
// product.component.ts
import { Component } from '@angular/core';
import { RouterLink } from '@angular/router';
@Component({
selector: 'app-product',
standalone: true,
imports: [RouterLink],
templateUrl: './product.component.html',
styleUrl: './product.component.scss'
})
export class ProductComponent {
products = [
{
id: 1,
name: 'Product 1',
description: 'This is a product description.'
},
{
id: 2,
name: 'Product 2',
description: 'This is a product description.'
},
{
id: 3,
name: 'Product 3',
description: 'This is a product description.'
}
]
}
JavaScript
// product-detail.component.ts
import { Component } from '@angular/core';
import { ActivatedRoute, RouterLink } from '@angular/router';
@Component({
selector: 'app-product-detail',
standalone: true,
imports: [RouterLink],
templateUrl: './product-detail.component.html',
styleUrl: './product-detail.component.scss',
})
export class ProductDetailComponent {
productId: string | null = null;
constructor(private route: ActivatedRoute) {
this.route.params.subscribe((params) => {
this.productId = params['productId'];
});
}
}
JavaScript
// app.routes.ts
import { Routes } from '@angular/router';
export const routes: Routes = [
{
path: '',
redirectTo: 'product',
pathMatch: 'full',
},
{
path: 'product',
loadComponent: () =>
import('./product/product.component')
.then((m) => m.ProductComponent),
},
{
path: 'product/:productId',
loadComponent: () =>
import('./product/product-detail/product-detail.component').then(
(m) => m.ProductDetailComponent
),
},
];
JavaScript
// app.component.ts
import { Component } from '@angular/core';
import { RouterOutlet } from '@angular/router';
@Component({
selector: 'app-root',
standalone: true,
imports: [RouterOutlet],
templateUrl: './app.component.html',
styleUrl: './app.component.scss'
})
export class AppComponent {
title = 'route-parameter';
}
To start the application run the following command.
ng serve
Output
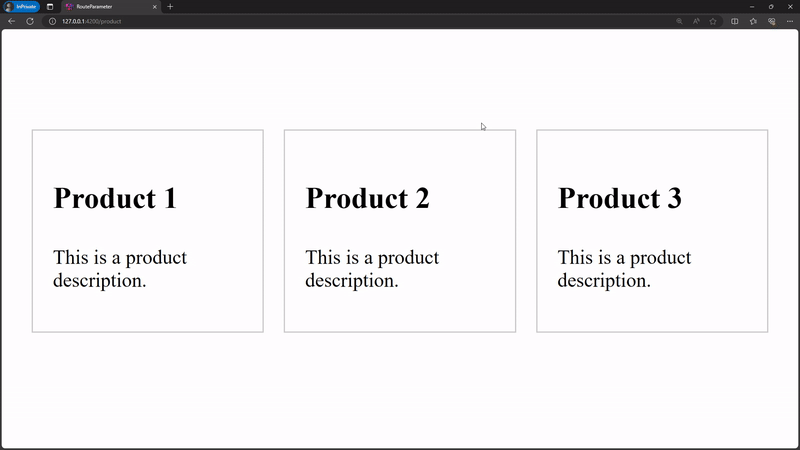
Share your thoughts in the comments
Please Login to comment...