How to Enable HTML 5 Mode in Angular 1.x ?
Last Updated :
25 Aug, 2023
HTML5 mode in Angular1.x is the configuration that replaces the default hash-based URLs and provides more user-friendly URLs using the HTML5 History API. This feature mainly enhances our one-page application and also provides a better experience to the users. We need to configure our server with proper routes and URL paths. Then, we can inject the “$locationProvider” and invoke the ‘html5Mode(true)’ function. We will also explore one more approach or enable the HTML5 mode in Angular1.x.
Steps to Enable HTML5 mode in Angular 1.x
The below steps will be followed for enabling HTML5 mode in Angular 1.x.
Step 1: Create a new folder for the project. As we are using the VSCode IDE, we can execute the command in the integrated terminal of VSCode.
mkdir angular1-html5-mode-example1
cd angular1-html5-mode-example1
Step 2: Now, initialize the npm for our example using the below command”
npm init
Step 3: Now, we need to install the Angular 1.x and HTTP-server dependencies by executing the below command in the terminal.
npm install angular@1 http-server --save
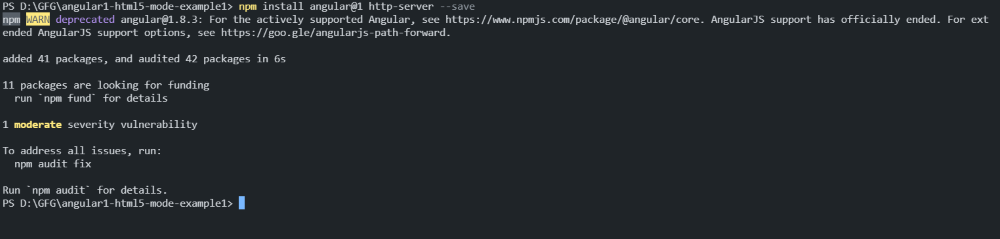
We will understand the above concept with the help of suitable examples & will understand its implementation through the illustration.
Approach 1: Using $locationprovider.html5mode(true) Method
In this approach, we will enhance the overall URL structure by removing the hash symbol from the route URLs. In spite of this, we will utilize the browser’s HTML5 History API to change and manipulate the URL without performing the complete page reload. Create the following files in the newly created folder, in these files we will have our all logic.
Project Structure:
Follow the below directory structure:
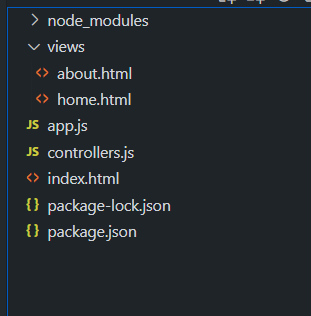
Add the below code in the respective files as shown in the above directory structure:
Example: Below is an example that uses the $locationprovider.html5mode(true) method.
Javascript
var app = angular.module( 'myApp' , [ 'ngRoute' ]);
app.config([ '$routeProvider' ,
'$locationProvider' ,
function ($routeProvider, $locationProvider) {
$routeProvider
.when( '/' , {
templateUrl: 'views/home.html' ,
controller: 'HomeController'
})
.when( '/about' , {
templateUrl: 'views/about.html' ,
controller: 'AboutController'
})
.otherwise({ redirectTo: '/' });
$locationProvider.html5Mode( true );
}]);
|
Javascript
app.controller( 'HomeController' , [ '$scope' , function ($scope) {
$scope.message = 'Welcome to the Home Page!' ;
}]);
app.controller( 'AboutController' , [ '$scope' , function ($scope) {
$scope.message = 'This is the About Page.' ;
}]);
|
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< base href = "/" >
< title >AngularJS HTML5 Mode</ title >
< style >
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
nav {
background-color: #333;
color: #fff;
padding: 10px;
text-align: center;
}
nav a {
color: #fff;
text-decoration: none;
margin: 0 10px;
}
h1 {
color: #008000;
text-align: center;
}
h3 {
color: #333;
text-align: center;
}
.container {
padding: 20px;
}
.message-container {
background-color: #f4f4f4;
border: 1px solid #ddd;
padding: 20px;
margin-top: 20px;
text-align: center;
}
.message {
font-size: 18px;
color: #333;
line-height: 1.5;
}
</ style >
</ head >
< body >
< nav >
< a href = "/#!/" >Home</ a > | < a href = "/#!/about" >About</ a >
</ nav >
< div ng-view class = "container" ></ div >
< script src =
</ script >
< script src =
</ script >
< script src = "app.js" ></ script >
< script src = "controllers.js" ></ script >
</ body >
</ html >
|
HTML
< div >
< h1 >GeeksforGeeks</ h1 >
< h3 >Enable HTML5 Mode in Angular 1.x</ h3 >
< div class = "message-container" >
< p class = "message" >{{ message }}</ p >
</ div >
</ div >
|
HTML
< div >
< h1 >GeeksforGeeks</ h1 >
< h3 >Enable HTML5 Mode in Angular 1.x</ h3 >
< div class = "message-container" >
< p class = "message" >{{ message }}</ p >
</ div >
</ div >
|
- package.json: Below is the package.json file for the reference:
{
"name": "angular1-html5-mode-example1",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC",
"dependencies": {
"angular": "^1.8.3",
"express": "^4.18.2",
"http-server": "^14.1.1"
}
}
- Run the application using the below command:
cd public/
python -m http.server
Output:
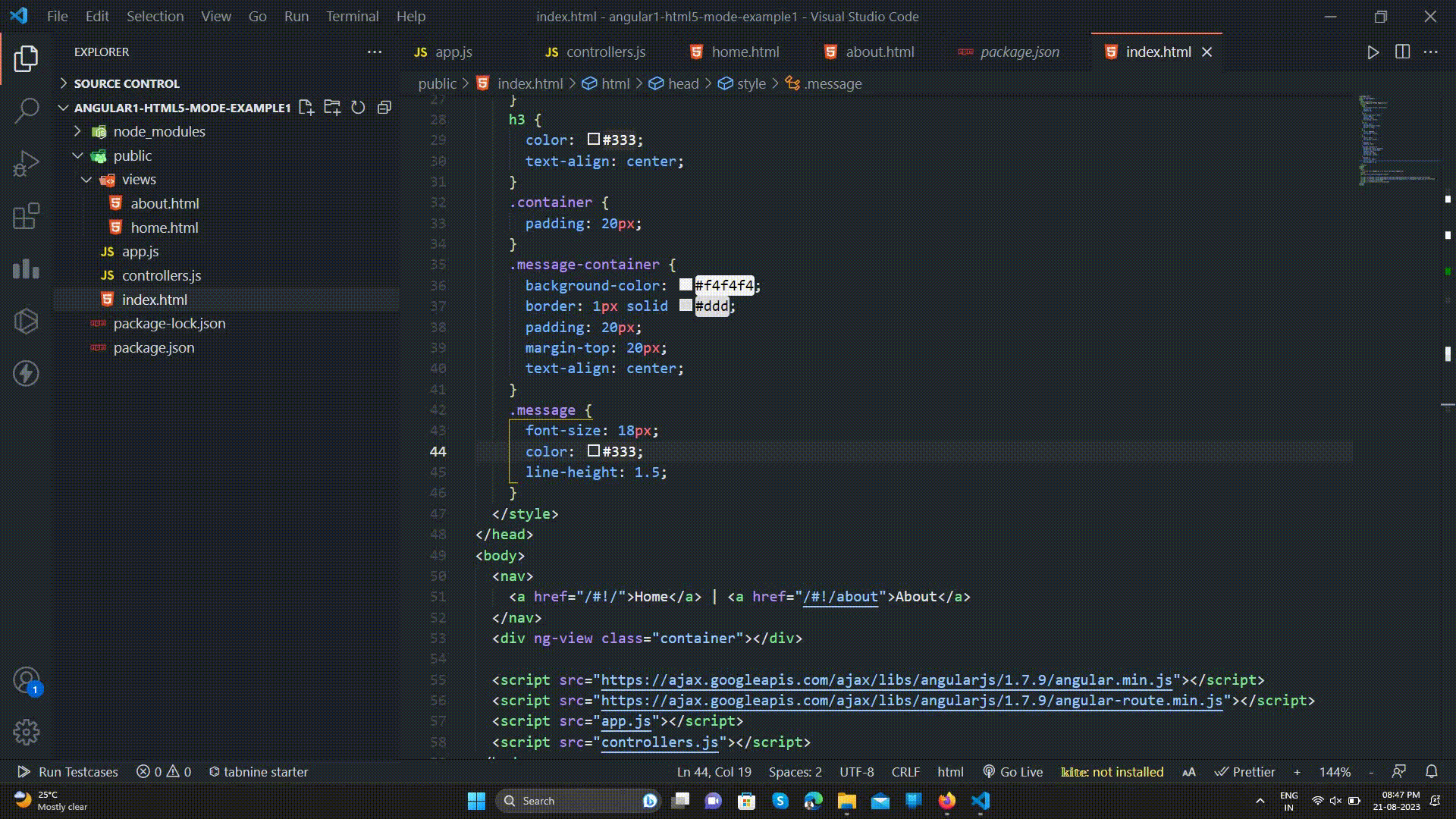
Approach 2: Using Hashbang Mode
In this approach, we will be using the Hashbang mode which is also known as the “hash-routing” technique. In this approach, the hash symbol which is used in the URL is used to manage the client-side routing of the application. This is the default approach in AngularJS which enables a way to navigate through various views rather than performing page reloads. Here, the hash portion of the URL to decide which view to display and it also listens for the changes to the hash to manipulate and change the content of the actual page.
Steps to Configure the Express:
Install the required dependency for this approach. Use the below command to install the dependency:
npm install express
Project Structure:
Create the following files in the newly created folder, in these files we will have our all logic. Follow the below directory structure:
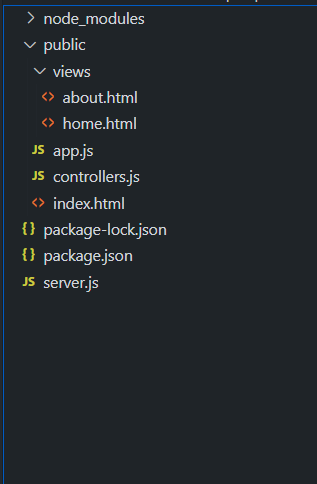
Example: This example illustrates enabling the HTML5 mode in Angular 1.x using Hashbang Mode.
Javascript
var app = angular.module( 'myApp' , [ 'ngRoute' ]);
app.config([ '$routeProvider' , function ($routeProvider) {
$routeProvider
.when( '/' , {
templateUrl: 'views/home.html' ,
controller: 'HomeController'
})
.when( '/about' , {
templateUrl: 'views/about.html' ,
controller: 'AboutController'
})
.otherwise({ redirectTo: '/' });
}]);
|
Javascript
app.controller( 'HomeController' , [ '$scope' , function ($scope) {
$scope.message = 'Welcome to the Home Page!' ;
}]);
app.controller( 'AboutController' , [ '$scope' , function ($scope) {
$scope.message = 'This is the About Page.' ;
}]);
|
Javascript
const express = require( 'express' );
const path = require( 'path' );
const app = express();
app.use(express.static(path.join(__dirname, 'public' )));
app.get( '*' , (req, res) => {
res.sendFile(path.join(__dirname, 'public' , 'index.html' ));
});
const PORT = process.env.PORT || 8000;
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
|
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< title >AngularJS Hashbang Mode</ title >
< style >
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
background-color: #f0f0f0;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
}
nav {
background-color: #333;
color: #fff;
padding: 10px;
text-align: center;
width: 100%;
position: fixed;
top: 0;
}
nav a {
color: #fff;
text-decoration: none;
margin: 0 10px;
}
.container {
width: 80%;
margin-top: 50px;
text-align: center;
padding: 20px;
background-color: #fff;
border-radius: 8px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.1);
}
.title {
color: #008000;
font-size: 36px;
margin-bottom: 10px;
}
.subtitle {
font-size: 24px;
color: #333;
margin-bottom: 20px;
}
.message-container {
background-color: #f5f5f5;
border: 1px solid #ddd;
padding: 20px;
border-radius: 4px;
box-shadow: 0 2px 4px rgba(0, 0, 0, 0.1);
}
.message {
font-size: 20px;
color: #444;
line-height: 1.6;
}
.message-container .message {
opacity: 0;
animation: fadeIn 1s ease-in-out forwards;
}
@keyframes fadeIn {
from {
opacity: 0;
transform: translateY(10px);
}
to {
opacity: 1;
transform: translateY(0);
}
}
</ style >
</ head >
< body >
< nav >
< a href = "#!/" >Home</ a > | < a href = "#!/about" >About</ a >
</ nav >
< div ng-view class = "container" ></ div >
< script src =
</ script >
< script src =
</ script >
< script src = "app.js" ></ script >
< script src = "controllers.js" ></ script >
</ body >
</ html >
|
HTML
< div >
< h1 class = "title" >GeeksforGeeks</ h1 >
< h3 class = "subtitle" >
Enabling HTML5 mode in Angular 1.x using Hashbang Mode
</ h3 >
< div class = "message-container" >
< p class = "message" >{{ message }}</ p >
</ div >
</ div >
|
HTML
< div >
< h1 class = "title" >GeeksforGeeks</ h1 >
< h3 class = "subtitle" >
Enabling HTML5 mode in Angular 1.x using Hashbang Mode
</ h3 >
< div class = "message-container" >
< p class = "message" >{{ message }}</ p >
</ div >
</ div >
|
- package.json: Below is the package.json file content for reference:
{
"name": "angular1-html5-mode-example1",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC",
"dependencies": {
"angular": "^1.8.3",
"express": "^4.18.2",
"http-server": "^14.1.1"
}
}
- Run the application using the below command:
node server.js
Output:
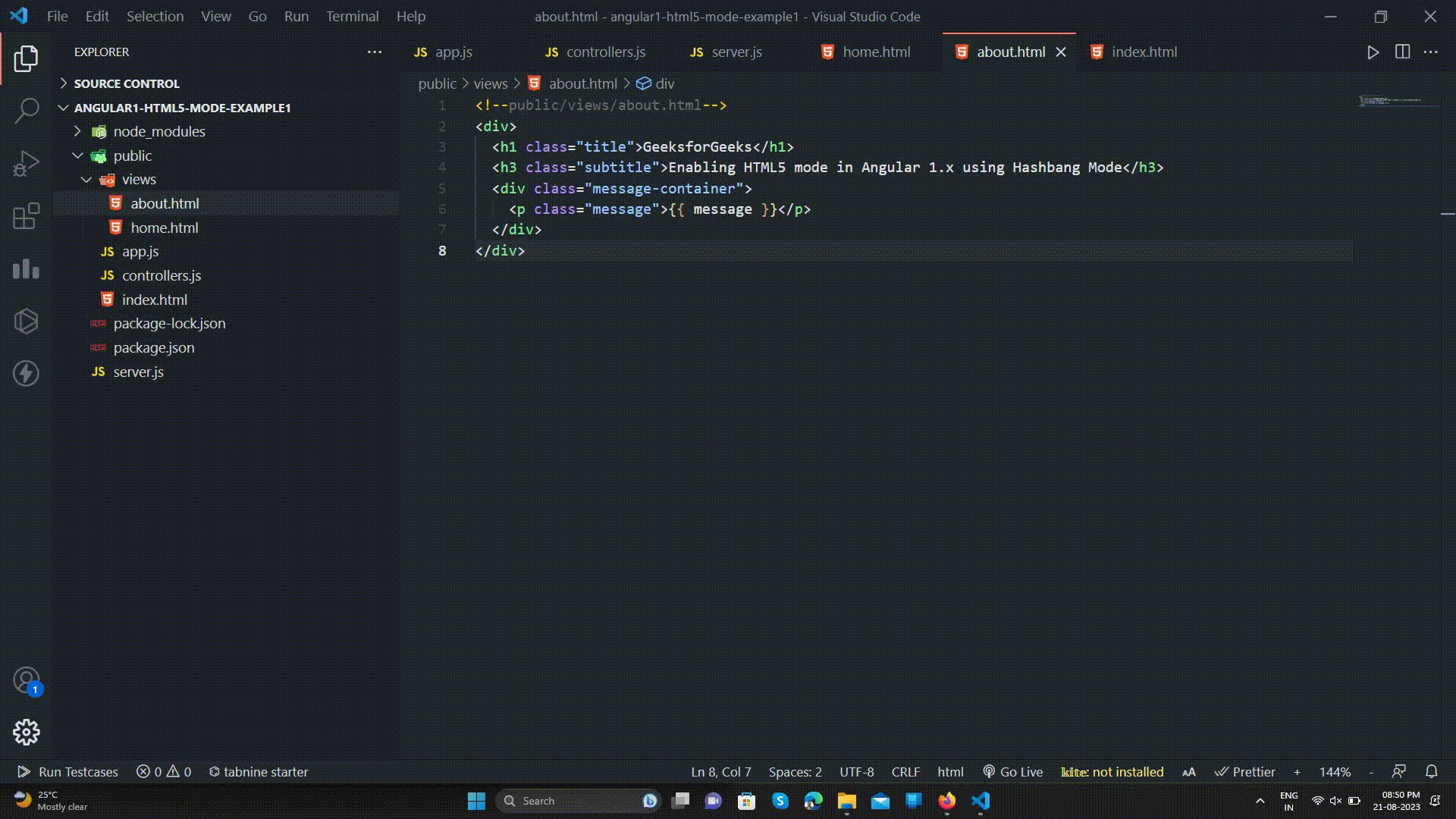
Share your thoughts in the comments
Please Login to comment...