How to get the Length of the Path in SVG ?
Last Updated :
12 Apr, 2024
The Scalable Vector Graphics (SVG) is a widely used the XML-based format for describing two-dimensional vector graphics. When working with the SVG paths in JavaScript we may need to determine the length of path for the various purposes such as the animation, drawing or interactive effects.
These are the following methods:
Using getTotalLength() Method
This approach involves directly using getTotalLength() method provided by SVGPathElement interface to the retrieve the total length of SVG path. The approach involves the selecting the SVG path element using the document.querySelector() or similar method and then calling the getTotalLength() method on selected path element. This method directly computes the length of path based on its definition and returns the result.
Syntax:
let path = document.querySelector('path');
let length = path.getTotalLength();
Parameters:
- document.querySelector(‘path’): This syntax selects the first <path> element in document using the querySelector method.
- path.getTotalLength(): This syntax calls the getTotalLength() method on selected <path> element in which returns the total length of path.
Example: This approach involves using getTotalLength() method provided by SVGPathElement interface to the retrieve the total length of SVG path.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>The SVG Path Length</title>
</head>
<body>
<svg width="100" height="100">
<path id="path"
d="M10 10 L0 90"
stroke="black" />
</svg>
<div id="output"></div>
<script>
document.addEventListener('DOMContentLoaded', function () {
let path = document.querySelector('#path');
let length = path.getTotalLength();
let output = document.querySelector('#output');
output.innerHTML = 'Length of the path: ' + length;
});
</script>
</body>
</html>
Output:
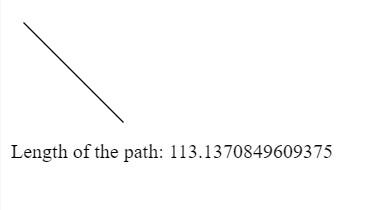
Using getBoundingClientRect() Method
This approach involves getting the bounding box of the SVG path using getBoundingClientRect() method and then calculating the diagonal length of bounding box. The approach using getBoundingClientRect() method involves the obtaining the bounding box of SVG path element in which represents its position and size relative to viewport.
Syntax:
let path = document.querySelector('path');
let box = path.getBoundingClientRect();
Parameters:
- document.querySelector(‘path’): Selects the first <path> element in document.
- path.getBoundingClientRect(): Retrieves the bounding rectangle of selected <path> element in which represents its position and size relative to viewport.
Example: This approach involves the getting the bounding box of the SVG path using getBoundingClientRect() method and then calculating the diagonal length of bounding box.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width,
initial-scale=1.0">
<title>The SVG Path Length</title>
</head>
<body>
<svg width="100" height="100">
<path d="M10 10 L90 90" stroke="black" />
</svg>
<div id="output"></div>
<script>
document.addEventListener('DOMContentLoaded', function () {
let path = document.querySelector('path');
let bbox = path.getBoundingClientRect();
let length = Math.sqrt(Math.pow(bbox.width,
2) + Math.pow(bbox.height, 2));
document.getElementById('output')
.innerText = 'Length of path: ' + length;
});
</script>
</body>
</html>
Output:
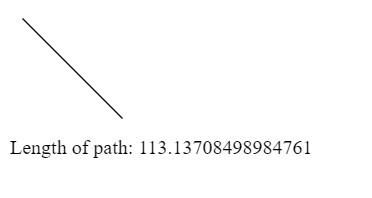
Share your thoughts in the comments
Please Login to comment...