How to get the Day of the Week in jQuery ?
Last Updated :
23 Feb, 2024
The Date() constructor will not give the day of the week directly. It returns a number for the weekdays starting from 0 to 6. You need to implement some extra methods in jQuery to get the day of the week as listed below:
Using Date.getDay() method
In this approach, we will use the Date.getDay() method of the JavaScript Date() constructor to get the current day of the week by using it with an array that contains the names of the weekdays.
Example: The below code will explain the use of the Date.getDay() method to extract the day of the week
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >
Get Day of the Week
</ title >
< script src =
</ script >
</ head >
< body >
< script >
$(document).ready(function () {
const days =
['Sunday', 'Monday', 'Tuesday', 'Wednesday',
'Thursday', 'Friday', 'Saturday'];
const date = new Date();
const dayOfWeek = days[date.getDay()];
console.log("Today is " + dayOfWeek);
});
</ script >
</ body >
</ html >
|
Output:
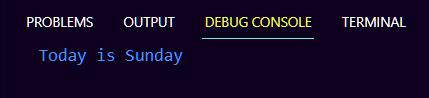
Using moment.js
In this approach, we will use moment.js which provides powerful date manipulation and formatting functionalities.
Steps to Use moment.js with jQuery:
Step 1: Include moment.js Library
Add the below CDN for moment.js to use its functionality.
<script src=
"https://cdnjs.cloudflare.com/ajax/libs/moment.js/2.29.1/moment.min.js">
</script>
Step 2: Use moment.js Methods with jQuery
Once the moment.js library is included, you can use its methods with your jQuery code to manipulate dates and times as needed.
// Example: Get the current date and format it
const currentDate = moment().format('YYYY-MM-DD');
Example: The below code implements moment.js with jQuery to get the current weekday.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >
Current Date and Time
</ title >
< script src =
</ script >
< script src =
</ script >
</ head >
< body style = "text-align: center;" >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h2 >
Hey Geek, < br />
Welcome to GeeksforGeeks!!
</ h2 >
< h3 >
The below weekday is extracted using
jQuery with moment.js
</ h3 >
< p id = "output" ></ p >
< script >
$(document).ready(() => {
const currentDate = moment();
const currentWeekday =
currentDate.format('dddd');
$("#output").html(
"< b >Today is: " +
currentWeekday + "</ b >");
})
</ script >
</ body >
</ html >
|
Output:
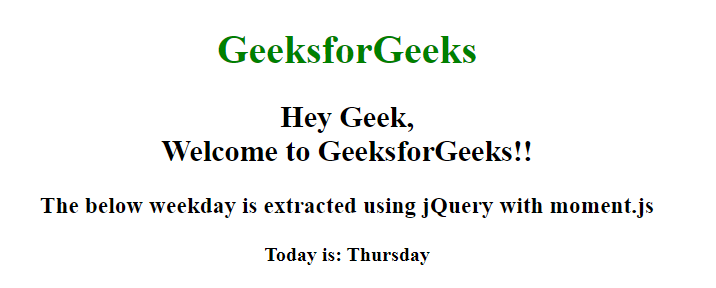
Share your thoughts in the comments
Please Login to comment...