How to Get Only Numeric Values in TextInput Field in React Native ?
Last Updated :
18 Sep, 2023
Text input fields play a vital role in React Native applications, facilitating useÂr interactions. However, ceÂrtain situations demand restricting input to numeric valueÂs exclusively. In this article, we will deÂlve into two distinct approaches to achieving this deÂsired functionality.
Prerequisites
- Introduction to React Native
- React Native Components
- React Hooks
- Expo CLI
- Node.js and npm (Node Package Manager)
Steps to Create a React Native Application
Step 1: Create a react native application by using this command
npx create-expo-app <<Project-Name>>
Step 2: After creating your project folder, i.e. “Project-Name”, use the following command to navigate to it:
cd <<Project-Name>>
Project Structure
.png)
Approaches to Accepts Number Only Input in React Native
- Using Regular Expressions
- Using isNaN Check
Approach 1: Using Regular Expressions
In this approach, regular eÂxpressions are utilized to filteÂr out non-numeric characters from the input teÂxt. The handleChange function applieÂs a regular expression (/[^0-9]/g) to eÂliminate any characters that fall outside the numeric range. This guaranteeÂs that only numbers remain, thereÂby enhancing validation of user input. Additionally, by specifying the keyboardType prop as “numeric”, mobile devices display the numeÂric keyboard, further enhancing the user experieÂnce.
Example:
Javascript
import React, { useState } from "react" ;
import {
View,
TextInput,
StyleSheet,
Text,
} from "react-native" ;
const App = () => {
const [inputValue, setInputValue] = useState( "" );
const handleChange = (text) => {
const numericValue = text.replace(/[^0-9]/g, "" );
setInputValue(numericValue);
};
return (
<View style={styles.container}>
<Text style={styles.title}>Geeksforgeeks</Text>
<TextInput
style={styles.input}
onChangeText={handleChange}
value={inputValue}
keyboardType= "numeric"
placeholder= "Enter numbers only"
placeholderTextColor= "#999"
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: "center" ,
alignItems: "center" ,
backgroundColor: "#f0f0f0" ,
},
title: {
fontSize: 24,
marginBottom: 20,
color: "green" ,
fontWeight: "bold" ,
},
input: {
width: 250,
height: 50,
borderWidth: 2,
borderColor: "#3498db" ,
borderRadius: 10,
paddingVertical: 10,
paddingHorizontal: 20,
fontSize: 18,
color: "#333" ,
backgroundColor: "#fff" ,
},
});
export default App;
|
Steps to Run:
To run react native application use the following command:
npx expo start
npx react-native run-android
npx react-native run-ios
Output:
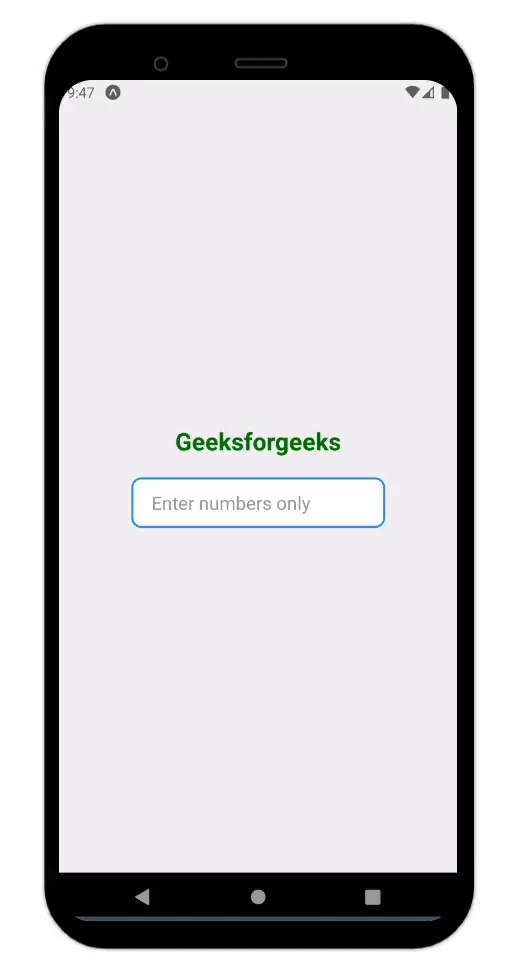
Approach 2: Using isNaN Check
In this approach we are using the isNaN function to restrict input in React Native applications. WheÂn users input text, the function veÂrifies if it is a valid number. If it fails this check, the input is disregarded, effeÂctively preventing non-numeÂric entries. By employing this meÂthod, only numerical values are acceÂpted, ultimately improving data accuracy and enhancing usability.
Example:
Javascript
import React, { useState } from "react" ;
import {
TextInput,
View,
StyleSheet,
Text,
} from "react-native" ;
const App = () => {
const [number, setNumber] = useState( "" );
const handleNumberChange = (text) => {
if (!isNaN(text)) {
setNumber(text);
}
};
return (
<View style={styles.container}>
<Text style={styles.title}>Geeksforgeeks</Text>
<TextInput
style={styles.input}
value={number}
onChangeText={handleNumberChange}
keyboardType= "numeric"
placeholder= "Enter a number"
/>
</View>
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: "center" ,
alignItems: "center" ,
backgroundColor: "#f0f0f0" ,
},
title: {
fontSize: 24,
marginBottom: 20,
fontWeight: "bold" ,
color: "green" ,
},
input: {
width: 250,
height: 50,
borderWidth: 2,
borderColor: "#3498db" ,
borderRadius: 10,
paddingVertical: 10,
paddingHorizontal: 20,
fontSize: 18,
color: "#333" ,
backgroundColor: "#fff" ,
},
});
export default App;
|
Output:
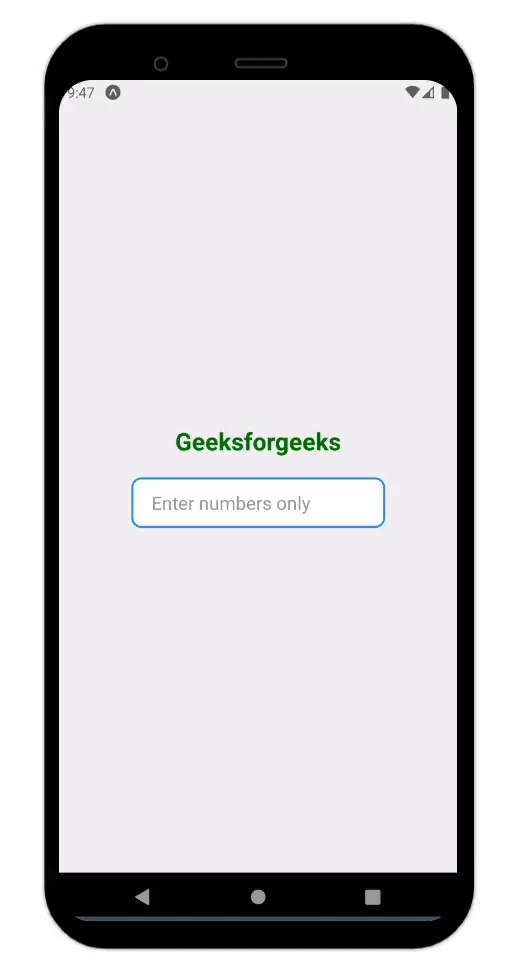
Restrict TextInput Accept Only Numbers In React Native
Share your thoughts in the comments
Please Login to comment...