How to Generate Random Number in React JS?
Last Updated :
17 Dec, 2023
This article explores generating random numbers in React.js, accomplished through the utilization of the Math.random() method.
- The math.random() method in JavaScript returns a random number between 0 (inclusive) and 1 (exclusive).
- The Math.floor() function in JavaScript is utilized to round off a given input number to the nearest smaller integer in a downward direction. In other words, it truncates the decimal part of the number.
Prerequisites:
Syntax:
Math.random();
Steps to Create React Application And Installing Module:
Step 1: Create a react application by using this command
npx create-react-app react-project
Step 2: After creating your project folder, i.e. react-project, use the following command to navigate to it:
cd react-project
Project Structure:
.png)
Project Structure
The updated dependencies in package.json file will look like:
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example: The “App” function utilizes useState to create a “num” state with an initial value of 0, includes a “randomNumberInRange” helper, and updates “num” on button click to display a random number between 1 to 20 in an h2 element inside a styled wrapper div.
Javascript
import React, { useState } from "react" ;
import "./App.css" ;
const App = () => {
const [num, setNum] = useState(0);
const randomNumberInRange = (min, max) => {
return Math.floor(Math.random()
* (max - min + 1)) + min;
};
const handleClick = () => {
setNum(randomNumberInRange(1, 20));
};
return (
<div className= "wrapper" >
<h2>Number is: {num}</h2>
<button onClick={handleClick}>
Click Me Generate
</button>
</div>
);
};
export default App;
|
CSS
.wrapper {
padding : 20px ;
text-align : center ;
}
h 2 {
font-size : 24px ;
margin-bottom : 10px ;
}
button {
background-color : green ;
color : white ;
padding : 10px 20px ;
border-radius: 5px ;
border : none ;
font-size : 16px ;
cursor : pointer ;
}
button:hover {
background-color : #3e8e41 ;
box-shadow: 1px 1px 10px 1px rgb ( 185 , 185 , 185 );
transform: translate( 0px , -2px );
}
|
Step To run the application: Open the Terminal and enter the command listed below.
npm start
Output:
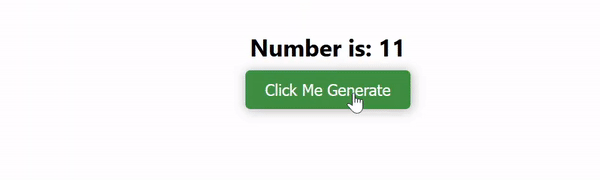
Output
Share your thoughts in the comments
Please Login to comment...