How to Format Currencies in a Vue Component ?
Last Updated :
10 Jan, 2024
In this article, we will use a Vue.js component with a simple HTML template containing <div> and button elements. The goal is to toggle the formatting of currencies within the <div> element when the button is clicked. By understanding the concepts of data binding, conditional rendering, and event handling in Vue.js, you’ll be able to create more interactive and visually appealing user interfaces.
Steps to Setup the Project Environment
Step 1: Create a VueJS application using this command.
npm create vue@latest
Step 2: Navigate to the project that you have just created by the following command.
cd your-project-name
Step 3: Run this command to install all packages
npm install
Step 4: Navigate to src and within src, go to the components folder. Inside it, we will edit the default HelloWorld.vue component file which was created during the Vue.js application creation.
Step 5: Setup the HTML template in the file using the template tag to define the structure of the web page using the HTML tags like button, div, headings etc.
Step 6: Define the data property that returns the default random number that has to be converted into the currency format.
Step 7: Now, create the formatCurrency and the reset methods to format the currency into the clicked format and reset it to the initial number using the below code.
methods: {
formatCurrency(value, countryCode, currencyCode) {
// You can customize the options based on your requirements
this.price = value.toLocaleString(`en-${countryCode}`, {
style: 'currency',
currency: `${currencyCode}`,
});
},
reset() {
this.price = 123456789.54;
},
},
Project Structure:
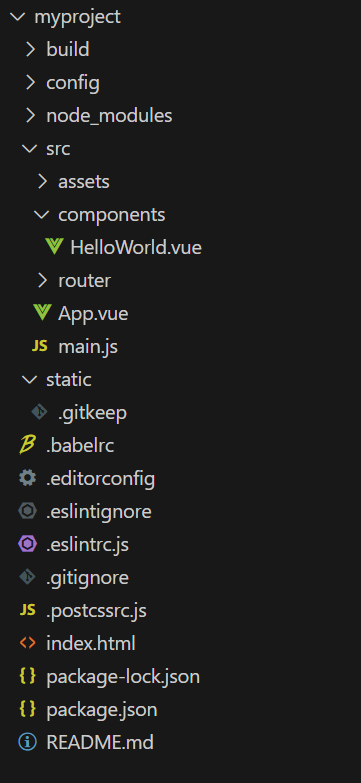
Example: The below code example converts the randomly choosen number into the US and the Indian currency based on the button click.
HTML
< template >
< div >
< p >
< span >Price: </ span >
{{ price }}
</ p >
< button @click=
"formatCurrency
(price, 'US', 'USD')">
Format to USD
</ button >
< button @click=
"formatCurrency
(price, 'IN', 'INR')">
Format to INR
</ button >
< button @ click = "reset()" >
Reset
</ button >
</ div >
</ template >
< script >
export default {
data() {
return {
price: 123456789.54,
};
},
methods: {
formatCurrency( value,
countryCode,
currencyCode) {
// You can customize the options
// based on your requirements
this.price = value.
toLocaleString(`en-${countryCode}`, {
style: 'currency',
currency: `${currencyCode}`,
});
},
reset() {
this.price = 123456789.54;
},
},
};
</ script >
< style >
span {
font-weight: bold;
}
button {
cursor: pointer;
}
button:not(last-child) {
margin-right: 0.5rem;
}
</ style >
|
HTML
< template >
< div id = "app" >
< HelloWorld />
</ div >
</ template >
< script >
import HelloWorld from
'./components/HelloWorld.vue'
export default {
name: 'App',
components: {
HelloWorld
}
}
</ script >
< style >
#app {
font-family:
Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</ style >
|
Output:
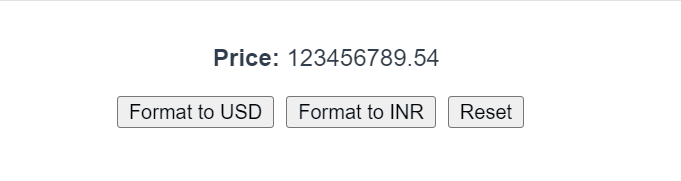
Share your thoughts in the comments
Please Login to comment...