How to Bind Attributes in VueJS ?
Last Updated :
27 Dec, 2023
In Vue.JS, the attribute binding allows us to properly manipulate the HTML elements that are based on the Vue Data properties and the expressions. We can bind these attributes using 2 different methods as listed below:
Approach 1: Using v-bind
In this approach, we use the v-bind directive to bind attributes in Vue.js. We will dynamically bind the src attribute of an image element to the imageUrl data property. By this, users can input the image URL, which will be rendered and displayed on the UI screen.
Syntax:
<element v-bind="{ attribute: expression }"></element>
Example: Below is the implementation of the v-bind approach to bind attributes in VueJS.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Example 1
</ title >
< style >
#app{
text-align: center;
}
#app img {
max-width: 100%;
height: auto;
}
h1 {
color: green;
}
</ style >
</ head >
< body >
< div id = "app" >
< h1 >
GeeksforGeeks
</ h1 >
< h3 >
Approach 1: Using v-bind
</ h3 >
< label for = "image-url" >
Enter Image URL:
</ label >
< input type = "text"
id = "image-url"
v-model = "imageUrl" >< br />
< img v-bind:src = "imageUrl"
alt = "Image" >
</ div >
< script src =
</ script >
< script >
new Vue({
el: '#app',
data: {
imageUrl:
}
});
</ script >
</ body >
</ html >
|
Output:
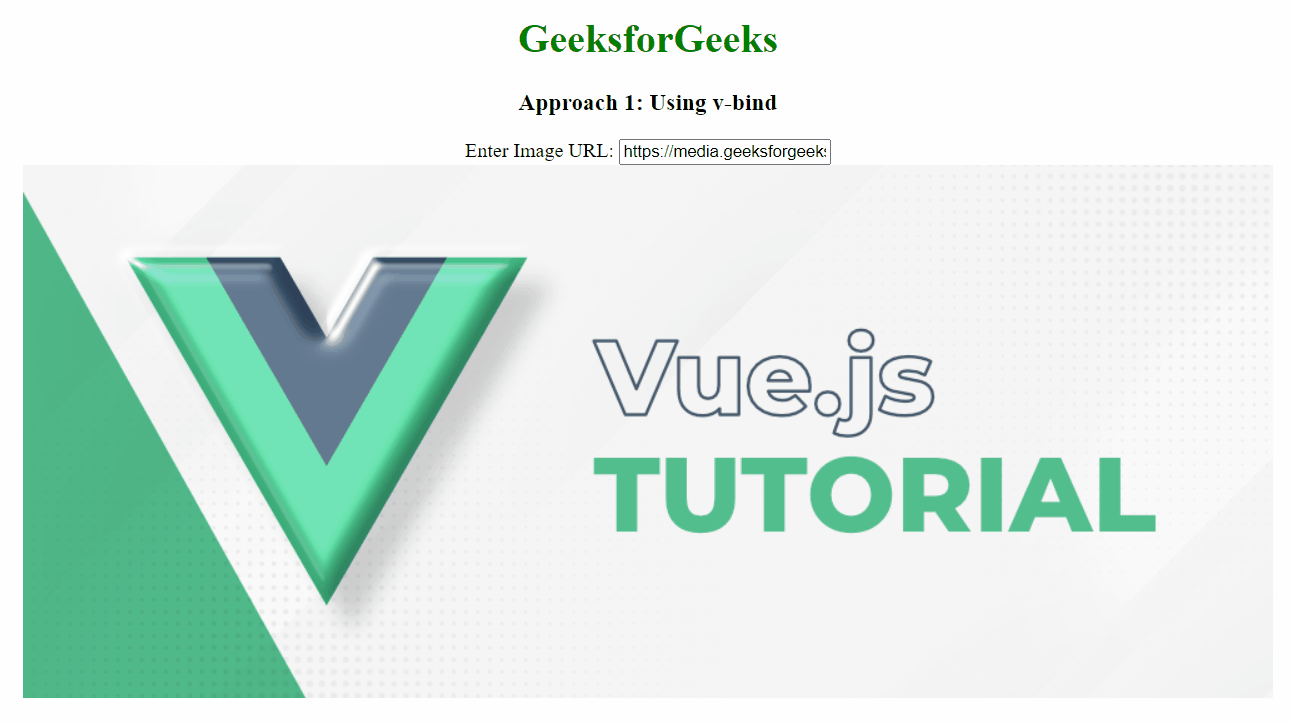
Approach 2: Using shortHand
In this apporach, we are performing binding of attributes using the shorthand as :Style to dyncmically change the background color of the button.
Syntax:
<element :attribute="expression"></element>
Example: The below code example implements the shorthand approach to bind attributes in VueJS.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
Vue.js Attribute Binding
</ title >
< style >
#app{
text-align: center;
}
h1 {
color: green;
}
</ style >
</ head >
< body >
< div id = "app" >
< h1 >
GeeksforGeeks
</ h1 >
< h3 >
Approach 2: Using Shorthand
</ h3 >
< button :style =
"{ backgroundColor: buttonColor }"
@ click = "changeBtnColor" >
Click me!
</ button >
</ div >
< script src =
</ script >
< script >
new Vue({
el: '#app',
data: {
buttonColor: 'blue',
},
methods: {
changeBtnColor() {
this.buttonColor =
colorFn();
}
}
});
function colorFn() {
const l = '0123456789ABCDEF';
let col = '#';
for (let i = 0; i < 6 ; i++) {
col +=
l[Math.floor(Math.random() * 16)];
}
return col;
}
</script>
</ body >
</ html >
|
Output:
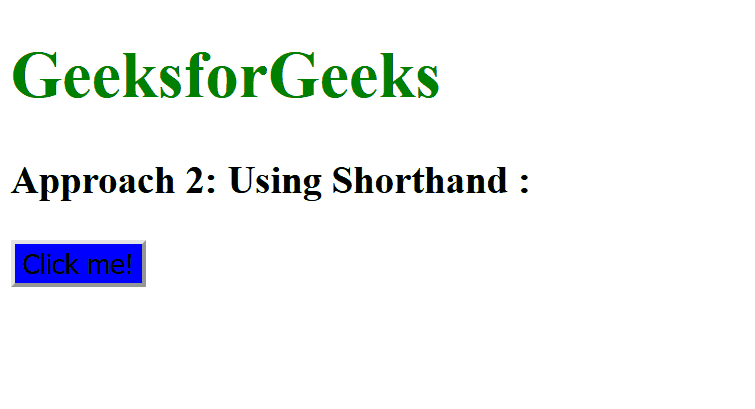
Share your thoughts in the comments
Please Login to comment...