How to Flatten Dynamically Nested Objects in Order in TypeScript ?
Last Updated :
12 Feb, 2024
We are required to flatten a Typescript nested object that contains arrays and objects as children such that there should be no nested children left and everything should be at the same height.
Example:
Input: obj = {
subject: "Computer Networks",
students: {
Jake: "USA"
}
};
Output: obj = {
"subject": "Computer Networks",
"students.Jake": "USA"
}
Using Recursion
Recursion is a programming technique that is used to solve different problems. In this technique, a function calls itself again and again until the terminating condition met. We can use this to flatten a nested object in TypeScript.
Approach:
- Create a function as flattenObject that takes an object as a parameter and returns it as a flattened object. Inside the function, we loop through each property of the input object to check its type.
- If it is an object, we recursively call the flattenObject function on that object. Otherwise, we simply store the value in the resultant object.
- After the given loop ends, we return the object as a result.
- Finally, we call the created function by passing the given response object inside.
Example:Â The below example illustrates the above approach to flatten the nested objects in TypeScript.
Javascript
let obj = {
company: "GeeksforGeeks" ,
members: {
John: "USA" ,
},
technology: {
language: "HTML, CSS" ,
library: {
name: "Node JS" ,
},
},
};
const flattenObject = (obj: any): any => {
let resultObj: any = {};
for (const i in obj) {
if ( typeof obj[i] === 'object' &&
!Array.isArray(obj[i])) {
const tempObj = flattenObject(obj[i]);
for (const j in tempObj) {
resultObj[i + '.' + j] = tempObj[j];
}
} else {
resultObj[i] = obj[i];
}
}
return resultObj;
};
const flattenedObject = flattenObject(obj);
console.log(flattenedObject);
console.log( "Accessing flattened properties: " )
console.log(flattenedObject[ 'technology.language' ]);
console.log(flattenedObject[ 'technology.library.name' ]);
|
Output:
company: "GeeksforGeeks"
members.John: "USA"
technology.language: "HTML, CSS"
technology.library.name: "Node JS"
Accessing flattened properties:
HTML, CSS
Node JS
Using the Lodash library
In this approach, we will use lodash library provided by NPM to flatten the given object. It provides several functions such as flatten, flattenDeep, and flatMap which allow us to recursively flatten nested objects and arrays.
Syntax:
const result: any = lodash.flatten(object);
Steps to use lodash with TypeScript:
- Step 1: Create a directory with the project name in your local system. Open the terminal and run the following commands to start with the project.
npm install lodash
npm install ts-node --save-dev
- Step 2: After installation, create a file app.ts in the root directory of the project and define a function flattenObject that utilizes lodash’s forEach() and flatten() method to flatten the object.
- Step 3: It constructs new keys by concatenating parent keys with child keys, separating them with dots.
- Step 4: Finally, it returns the resulting flattened object.
- Step 5: Run the application using the below command.
npx ts-node app.ts
Project Structure:
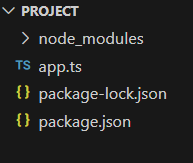
Example: The below code implements the lodash library to flatten the nested object in TypeScript.
Javascript
const lodash = require( 'lodash' );
let obj = {
company: "GeeksforGeeks" ,
members: {
John: "USA" ,
},
technology: {
language: "HTML, CSS" ,
library: {
name: "Node JS" ,
},
},
};
function flattenObject(obj: Record<string, any>):
Record<string, any> {
const resultObj: Record<string, any> = {};
function flatten(obj: Record<string, any>, prefix = '' ) {
lodash.forEach(obj, (value: any, key: string) => {
const newKey = prefix ? `${prefix}.${key}` : key;
if (lodash.isObject(value)) {
flatten(value, newKey);
} else {
resultObj[newKey] = value;
}
});
}
flatten(obj);
return resultObj;
}
const flattenedObject = flattenObject(obj);
console.log(flattenedObject);
console.log( "Accessing flattened properties: " )
console.log(flattenedObject[ 'technology.language' ]);
console.log(flattenedObject[ 'technology.library.name' ]);
|
Output:
company: "GeeksforGeeks"
members.John: "USA"
technology.language: "HTML, CSS"
technology.library.name: "Node JS"
Accessing flattened properties:
HTML, CSS
Node JS
Share your thoughts in the comments
Please Login to comment...