How to target Nested Objects from a JSON data file in React ?
Last Updated :
05 Apr, 2024
Accessing nested JSON objects is similar to accessing nested arrays. Suppose we have a JSON object called person
with a nested object ‘address
'
, and we want to access the ‘city'
property from the address
object. We can do this using dot notation like person.address.city
. This notation allows us to directly access properties of nested objects within the JSON structure.
Example:
const person = {
"name": "Geeks",
"address": {
"street": "Sector 136 Noida",
"city": "Noida",
"zipcode": "201310"
},
"email": "geeksforgeeks.org"
};
const city = person.address.city;
console.log("City:", city);
// Outputs: City: Noida
There are two main ways to target nested objects from a JSON data file:
Targeting Nested Objects with Dot Notation
Targeting nested objects from a JSON data file using dot notation involves specifying the path to the desired property by chaining object keys with dots. For example, accessing a property like ‘person.address.city’ targets the ‘city’ property within the nested ‘address’ object of the ‘person’ object. This approach simplifies navigation through nested structures and facilitates direct access to specific values within the JSON data.
Example: This example shows the targeting objects using dot notation.
Javascript
import React from 'react'
const NestedObjectExample = () => {
// Sample JSON data
const jsonData = {
person: {
name: 'Geeks',
address: {
street: 'Sector 136 Noida',
city: 'Noida',
zipcode: '201310'
},
email: 'geeksforgeeks.org'
}
}
// Access nested object using dot notation
const cityName = jsonData.person.address.city
return (
<div>
<h1>City Name:</h1>
<p>{cityName}</p>
</div>
)
}
export default NestedObjectExample
Output:
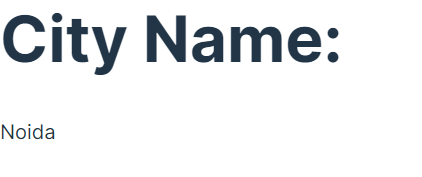
Bracket Notation for Dynamic Keys and Arrays
Bracket notation in JavaScript allows for dynamic access to object properties and array elements using variables or expressions within square brackets ( [ ] ). This is particularly useful when dealing with dynamic keys or indices in JSON data structures, enabling flexible and dynamic data manipulation in your code.
Example : This is another example to showcase for dynamic keys and Arryas.
Javascript
import React from 'react'
const DynamicKeysAndArraysExample = () => {
// Sample JSON data
const jsonData = {
person: {
name: 'geeks',
address: {
street: 'Sector 136 Noida',
city: 'Noida',
zipcode: '201310'
},
email: 'geeksforgeeks.org'
},
fruits: ['apple', 'banana', 'orange']
}
// Define dynamic keys and array index
const key = 'person'
const index = 1
// Access nested object with
// dynamic key and array element
const dynamicObject = jsonData[key]
const dynamicProperty = dynamicObject.address.city
const arrayElement = jsonData.fruits[index]
return (
<div>
<h2>Dynamic Property: {dynamicProperty}</h2>
<h2>Array Element: {arrayElement}</h2>
</div>
)
}
export default DynamicKeysAndArraysExample
Output:
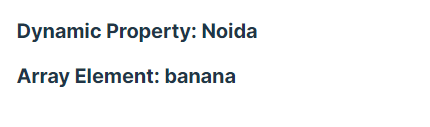
Example 2: This example shows fetching json data from an API and then accessing data.
Javascript
import React, { useState, useEffect } from 'react'
const NestedObjectExample = () => {
const [city, setCity] = useState('')
useEffect(() => {
// Fetch JSON data from the file
fetch('https://jsonplaceholder.typicode.com/users')
.then((response) => response.json())
.then((data) => {
// Access nested object using dot notation
const cityName = data[0].address.city
setCity(cityName)
})
.catch((error) =>
console.error('Error fetching data:', error))
}, [])
return (
<div>
<h1>City Name:</h1>
<p>{city}</p>
</div>
)
}
export default NestedObjectExample
Output:
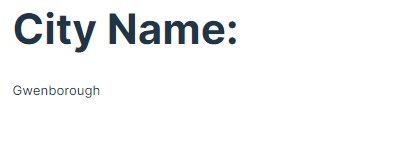
Share your thoughts in the comments
Please Login to comment...