How to fix a 413: request entity too large error ?
Last Updated :
22 Jan, 2024
In this article, we will learn about an Error request entity too large which typically occurs when the size of the data, being sent to a server, exceeds the maximum limit allowed by the server or the web application in web development when handling file uploads, form submissions, or any other data sent in the request body.
We will discuss the following approaches to resolve this error:
What is the request entity too large error ?
The “Error: request entity too large” typically occurs when the size of the data being sent in a request exceeds the server’s configured limit. This error is common in web applications that handle file uploads or large data payloads.
Steps to setup the Node Application:
Step 1: Initialize a directory as a new Node.js project
npm init -y
Step 2: Install Express and body-parser
npm install express body-parser
The updated dependencies in package.json file for backend will look like:
"dependencies": {
"body-parser": "^1.20.2",
"express": "^4.18.2",
}
Step 3: Create an Express server and add the following code:
Javascript
const express = require( 'express' );
const bodyParser = require( 'body-parser' );
const app = express();
app.use(bodyParser.json({ limit: '1kb' }));
app.post( '/api/data' , (req, res) => {
const data = req.body;
console.log( 'Received data:' , data);
res.status(200).json({ message: 'Data received successfully.' });
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server is running on http:
});
|
Step 4: Run the server
node app.js
Step 5: Test the endpoint with a large payload
You can use tools like Postman or curl to send a POST request with a large JSON payload to the `/api/data` endpoint. The server should now be able to handle larger JSON payloads with the “Request Entity Too Large” error.
Output: Your Express server is now running on http://localhost:3000.
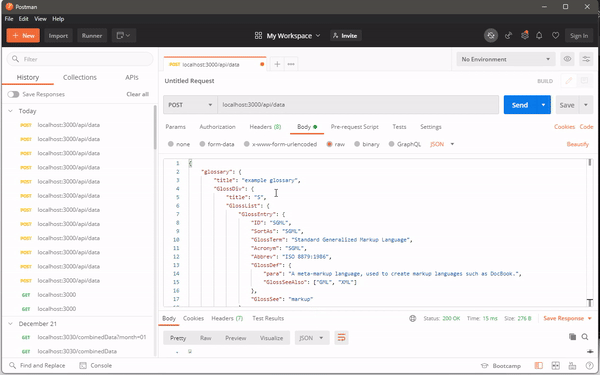
Middleware Approach:
Use the body-parser middleware to handle incoming JSON payloads and set the limit option to increase the allowed request size.
Example: Below is the code example of middleware
Javascript
const express = require( 'express' );
const bodyParser = require( 'body-parser' );
const app = express();
app.use(bodyParser.json({ limit: '20mb' }));
app.post( '/api/data' , (req, res) => {
const data = req.body;
console.log( 'Received data:' , data);
res.status(200)
.json(
{
message: 'Data received successfully.'
}
);
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(
`Server is running on http:
);
});
|
In this example, the body-parser middleware is configured to parse JSON with a limit of 20MB. Adjust the limit according to your specific requirements.
Output:
.gif)
File Upload Middleware Approach:
If you are dealing with file uploads, you may encounter the error due to file size limitations. You can use the multer middleware for handling file uploads in Express
Example: Below is the code example of file upload using middleware:
Javascript
const express = require( 'express' );
const multer = require( 'multer' );
const app = express();
const storage = multer.memoryStorage();
const upload = multer(
{
storage: storage,
limits: {
fileSize: 20 * 1024 * 1024
}
});
app.post( '/api/upload' ,
upload.single( 'image' ), (req, res) => {
const file = req.file;
console.log( 'Received file:' , file);
res.status(200)
.json(
{
message: 'File received successfully.'
}
);
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(
`Server is running on http:
);
});
|
In this example, multer is configured to accept file uploads with a maximum size of 20MB. Adjust the fileSize limit as needed.
Output:
.gif)
Raw Body Parsing Approach:
You can directly access and parse the raw request body. This gives you more control over the parsing process.
Example: Below is the code example of raw body parsing
Javascript
const express = require( 'express' );
const app = express();
app.use((req, res, next) => {
let data = '' ;
req.setEncoding( 'utf8' );
req.on( 'data' , (chunk) => {
data += chunk;
});
req.on( 'end' , () => {
req.body = data;
next();
});
});
app.post( '/api/rawdata' , (req, res) => {
console.log( 'Received raw data:' , req.body);
res.status(200)
.json(
{
message: 'Raw data received successfully.'
}
);
});
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(
`Server is running on http:
);
});
|
This approach manually reads and concatenates chunks of the request body to give you control over the entire request payload and handle large payloads efficiently.
Output:
.gif)
Share your thoughts in the comments
Please Login to comment...