How to validate POST request body required params in Pre-request script?
Last Updated :
01 Dec, 2023
APIs are a set of rules and protocols that allow different software applications to communicate and exchange data with each other, so it is important to test these APIs for their reliability by doing so-called API testing. API testing or application programming interface testing is a type of software testing that verifies the functionality, reliability, performance, and security of the API’s. There are different API requests like(POST, DELETE, GET, PUT, Etc..) in some requests like POST we have to pass the data as JSON and it is common that we will miss some required parameters especially if the parameters are huge. So in order to validate it we can make use of Pre-request scripts let’s explore in this article how we can validate the POST request body required params in a Pre-request script.
What is a Pre-request Script?
The pre-request script is a piece of JavaScript code that will be executed even before the API request.
- It allows us to add dynamic behavior to our request.
- If we have to pass the current time every time we send a request or have to pass a unique number each time it is difficult to enter it manually, the Pre-request script solves this issue.
In Postman the pre-request script is executed even before the request after that, an API request returns a response on which the test is executed.
Approach
Follow the steps below to validate a post-request body required parameters in the Pre-request script:
- Take an array or an object to store all the required parameters in it so that we can compare them with the actual entered parameters in the body.
- We will then use pm API to get the whole request body parse it and store it in a variable.
- Then in the next step, we need to separate the keys from the object using Objects.key() method which will return only the keys which users have entered.
- In this step, we are going to filter out the unmatched elements by comparing the elements in both the user-entered parameters and the actual required parameters.
- After storing all the unmatched elements in a variable, we will check if the length of it is equal to zero, if not then there are some elements that users have skipped.
- In this step, we are going to use the Postman visualizer to display to the user that he has skipped some required parameters with a list of them.
- Or if the user has entered all the required parameters in the POST request body, then we are going to display a message in the visualizer that he has entered everything correctly.
Below is the Javascript code to implement the above approach:
Javascript
const Data = [ 'name' , 'Age' , 'Education' , 'Occupation' , 'MartialStatus' ];
data = pm.request.body;
body = data[data.mode];
JsonData = JSON.parse(body);
JsonData = Object.keys(JsonData);
const unMatchedData = Data.filter(keys => !JsonData.includes(keys));
var template = `
<html>
<head>
<style>
.container {
background-color: #3498db;
color: #fff;
padding: 20px;
width: 40%;
border-radius: 5px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2);
}
.message {
font-size: 18px;
font-weight: bold;
text-align: center;
margin: 0;
}
.cta-button {
display: block;
text-align: center;
margin-top: 20px;
padding: 10px 20px;
background-color: #e74c3c;
color: #fff;
text-decoration: none;
border-radius: 3px;
transition: background-color 0.3s ease;
}
.cta-button:hover {
background-color: #c0392b;
}
</style>
</head>
<body>
<div class= 'container' >
<p class= 'message' >OOps U missed some parameters ${unMatchedData}</p>
<a href= "#" class= "cta-button" >Learn More</a>
</div>
</body>
</html>
`;
var result = {
res : "This is the result."
};
var template1 = `
<html>
<head>
<style>
.container {
background-color: #3498db;
color: #fff;
padding: 20px;
width: 40%;
border-radius: 5px;
box-shadow: 0 4px 8px rgba(0, 0, 0, 0.2);
}
.message {
font-size: 18px;
font-weight: bold;
text-align: center;
margin: 0;
}
.cta-button {
display: block;
text-align: center;
margin-top: 20px;
padding: 10px 20px;
background-color: #e74c3c;
color: #fff;
text-decoration: none;
border-radius: 3px;
transition: background-color 0.3s ease;
}
.cta-button:hover {
background-color: #c0392b;
}
</style>
</head>
<body>
<div class= 'container' >
<p class= 'message' >You have entered everything correctly</p>
<a href= "#" class= "cta-button" >Learn More</a>
</div>
</body>
</html>
`;
unMatchedData.length == 0 ? pm.visualizer.set(template1, result) : pm.visualizer.set(template, result);
|
In the Postman under the API tab, we can see the Pre-request Script click on it and write the code along with that we will get suggestions on the right pane to go through the documentation.
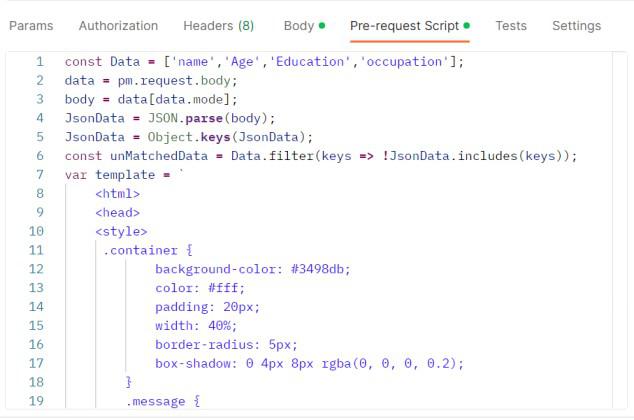
Steps to Run
To execute the script please follow the below mentioned steps:
- Click on the plus icon to create a new API request enter the URL of the API in the box and select the post request.
- Click on body then select raw and change the text to Json.
- Enter the data in the body as Json and also go to Pre-request script and write your script then just hit on send.
- Go to postman console and search for visualize tab open it you can see the output in it.
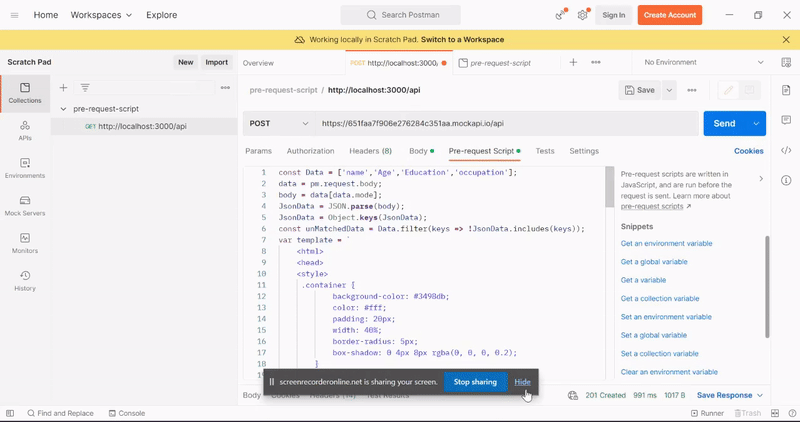
Output:
The two pictures below depict different outputs one when the user has missed the parameters entered, and the other when the user has entered all the parameters.
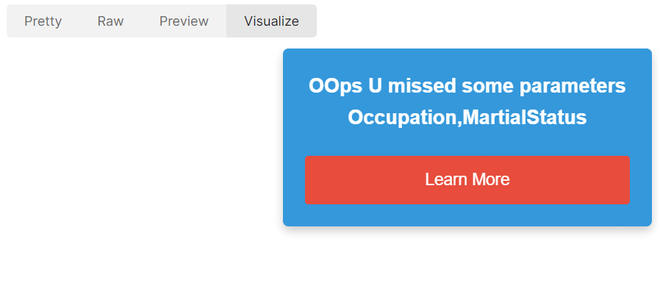
Missed required parameters
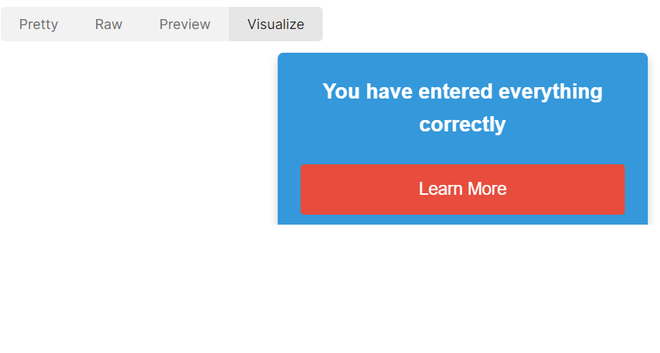
Entered all parameters
Conclusion
API testing is a crucial part of software development and integration, ensuring that different software applications can communicate effectively and securely. One essential aspect of API testing is validating the request body, especially when dealing with POST requests that require specific parameters. Pre-request script in tools like postman provide a powerful way to automate and streamline this validation process.
Share your thoughts in the comments
Please Login to comment...