How to create HTML list from JavaScript array ?
Last Updated :
07 Dec, 2023
In this article, we will be creating an HTML list from a JavaScript array. This is needed sometimes when we fetch JSON from any source and display the data in the front end and in many other cases.Â
Below are the ways to create the list from the Javascript array:
Method 1: Using the for loop
We can iterate in the list using a simple for loop and then append the list item using appendChild.
Example:
HTML
<!DOCTYPE html>
< html lang = "en" >
< body >
< center >
< h1 >GeeksforGeeks</ h1 >
</ center >
< ul id = "myList" ></ ul >
< script >
let data = ["Ram", "Shyam", "Sita", "Gita"];
let list = document.getElementById("myList");
for (i = 0; i < data.length ; ++i) {
let li = document .createElement('li');
li.innerText = data [i];
list.appendChild(li);
}
</script>
</ body >
</ html >
|
Output:
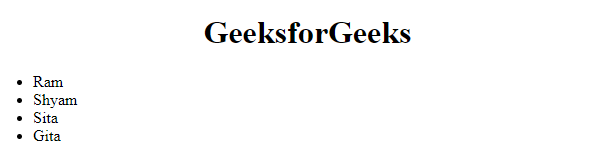
The arr.forEach() method calls the provided function once for each element of the array. The provided function may perform any kind of operation on the elements of the given array.Â
Syntax:
array.forEach(callback(element, index, arr), thisValue)
Example:
HTML
<!DOCTYPE html>
< html lang = "en" >
< body >
< center >
< h1 >GeeksforGeeks</ h1 >
</ center >
< ul id = "myList" ></ ul >
< script >
let data = ["Ram", "Shyam",
"Sita", "Gita"];
let list =
document.getElementById("myList");
data.forEach((item) => {
let li =
document.createElement("li");
li.innerText = item;
list.appendChild(li);
});
</ script >
</ body >
</ html >
|
Output:
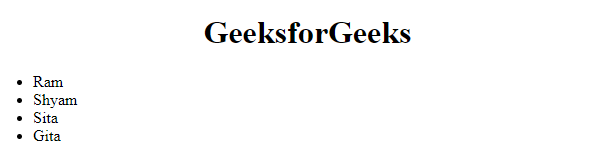
The JavaScript Array join() Method is used to join the elements of an array into a string. The elements of the string will be separated by a specified separator and its default value is a comma(, ).
Syntax:
array.join(separator)
Example:
Javascript
<!DOCTYPE html>
<html lang= "en" >
<body>
<center>
<h1>GeeksforGeeks</h1>
</center>
<ul id= "myList" ></ul>
<script>
let data = [ "Ram" , "Shyam" ,
"Sita" , "Gita" ];
let list =
document.getElementById( 'myList' );
let ul = `<ul>${data.map(data =>
`<li>${data}</li>`).join( '' )}
</ul>`;
list.innerHTML = ul;
</script>
</body>
</html>
|
Output:
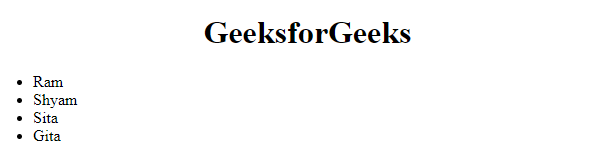
Share your thoughts in the comments
Please Login to comment...