Create a Drag and Drop Sortable List using HTML CSS & JavaScript
Last Updated :
15 Nov, 2023
In this article, we will create a drag-and-drop sortable list using HTML, CSS, and JavaScript. This interactive feature will allow users to reorder items in a list by simply dragging and dropping them.
Preview of final output: Let us have a look at how the final output will look like.
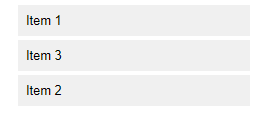
drag and drop sortable list
Prerequisites to create Drag & Drop
Approach
- HTML Structure: Start by defining the HTML structure for your sortable list. You will need an unordered list (<ul>) to hold the list items (<li>), and each list item should have the draggable attribute set to “true” to make them draggable.
- CSS Styling: Create a CSS file to style the list and list items. Style the items to make them visually appealing and clearly distinguishable.
- JavaScript for Drag and Drop: Implement JavaScript to add drag-and-drop functionality to the list items. This involves event listeners to handle the dragstart, dragend, and dragover events.
- Dragstart Event: When a list item is dragged, you need to store a reference to the dragged item and set its display to “none” temporarily to hide it.
- Dragend Event: After the drag operation is completed, you should reset the display property of the dragged item and clear the reference to the item.
- Dragover Event: This event is used to determine the position where the dragged item should be dropped. You’ll need to calculate the position relative to other list items and insert the dragged item accordingly.
- To make this process easier, you can create a function (getDragAfterElement) that finds the element where the dragged item should be placed.
Project Structure
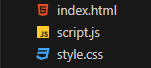
project structure
Example: Write the code in following files
- script.js: This file implements all the functionalities
- index.html: This file creates a skeleton structure of the document
- style.css: This file implements the styling of the application
Javascript
const sortableList =
document.getElementById( "sortable" );
let draggedItem = null ;
sortableList.addEventListener(
"dragstart" ,
(e) => {
draggedItem = e.target;
setTimeout(() => {
e.target.style.display =
"none" ;
}, 0);
});
sortableList.addEventListener(
"dragend" ,
(e) => {
setTimeout(() => {
e.target.style.display = "" ;
draggedItem = null ;
}, 0);
});
sortableList.addEventListener(
"dragover" ,
(e) => {
e.preventDefault();
const afterElement =
getDragAfterElement(
sortableList,
e.clientY);
const currentElement =
document.querySelector(
".dragging" );
if (afterElement == null ) {
sortableList.appendChild(
draggedItem
);}
else {
sortableList.insertBefore(
draggedItem,
afterElement
);}
});
const getDragAfterElement = (
container, y
) => {
const draggableElements = [
...container.querySelectorAll(
"li:not(.dragging)"
),];
return draggableElements.reduce(
(closest, child) => {
const box =
child.getBoundingClientRect();
const offset =
y - box.top - box.height / 2;
if (
offset < 0 &&
offset > closest.offset) {
return {
offset: offset,
element: child,
};}
else {
return closest;
}},
{
offset: Number.NEGATIVE_INFINITY,
}
).element;
};
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0" />
< link rel = "stylesheet" href = "style.css" />
< title >Drag and Drop Sortable List</ title >
</ head >
< body >
< div class = "sortable-list" >
< ul id = "sortable" >
< li draggable = "true" >Item 1</ li >
< li draggable = "true" >Item 2</ li >
< li draggable = "true" >Item 3</ li >
</ ul >
</ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
body {
font-family : Arial , sans-serif ;
}
.sortable-list {
max-width : 300px ;
margin : 0 auto ;
}
ul {
list-style-type : none ;
padding : 0 ;
}
li {
background-color : #f0f0f0 ;
padding : 10px ;
margin : 5px ;
cursor : grab;
}
|
Output
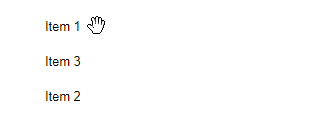
drag and drop
Share your thoughts in the comments
Please Login to comment...