Find the Array Index with a Value in JavaScript
Last Updated :
01 Feb, 2024
We have a given value and we need to find out whether the given is present in the array or not, If it is present then we have to print the index number of that existing value, and if it is not present then we will print -1.
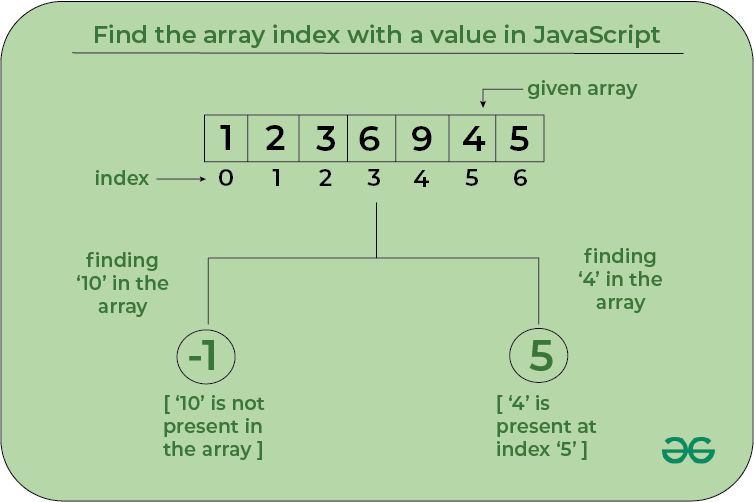
Example 1:
Input: ['apple', 'banana', 'cherry', 'orange']
N = 'cherry'
Output: 2
Explanation: The index of the word cherry is 2
These are the following approaches by using these we can find the Array Index with a Value:
- We have an array of fruits and want to find the index of the element ‘cherry’.
- We use the indexOf() method to search for ‘cherry’ in the fruits array and it returns the index of ‘cherry’, which is 2.
Example: This example is the implementation of the above-explained approach.
Javascript
const fruits = [ 'apple' , 'banana' , 'cherry' , 'orange' ];
const index = fruits.indexOf( 'cherry' );
console.log(index);
|
- The findIndex() method in JavaScript is used to get the index of the initial element in an array that meets a particular condition or testing function.
- The method returns the index of the first element that passes the test or -1 if no element passes the test. The findIndex() method does not modify the original array.
Example : This example is the implementation of the above-explained approach.
Javascript
const array = [10, 20, 30, 40];
const index = array.findIndex(num => num > 25);
console.log(index);
|
- Another approach to finding the index of an array with a specific value is to use a for loop to iterate through the array and compare each element with the value.
- The loop iterates through each element of the numbers array and checks if the current element is equal to 30.
- When element 30 is found at index 2, the loop is terminated using the break statement, and index 2 is stored in the index variable.
- Finally, the index variable is logged to the console, which outputs 2.
Example : This example is the implementation of the above-explained approach.
Javascript
const arraynumbers = [10, 20, 30, 40];
let index = -1;
for (let i = 0; i < arraynumbers.length; i++) {
if (arraynumbers[i] === 30) {
index = i;
break ;
}
}
console.log(index);
|
- In this approach, we are using the lodash that is the library of JavaSCript for fucntions.
- It has inbuilt function _.findIndex() which can be used to find the index of the given value.
- We have to pass the given value of an array and it will return the index number.
Example: This example is the implementation of the above-explained approach.
Javascript
const _ = require( 'lodash' );
let array1 = [4, 2, 3, 1, 4, 2]
let index = _.findIndex(array1, (e) => {
return e == 1;
}, 0);
console.log( "original Array: " , array1)
console.log( "index: " , index)
|
Output:
original Array: [ 4, 2, 3, 1, 4, 2]
index: 3
Share your thoughts in the comments
Please Login to comment...