How to Create Tooltip with Vue.js?
Last Updated :
22 Apr, 2024
Tooltips are the styling components used to provide informative or interactive hints when hovering over an element, enhancing user experience, and guiding interactions in web applications.
Below are the approaches to creating a tooltip with Vue.js:
Using Vue Directives
In this approach, we are using Vue directives to create a custom tooltip functionality. The v-tooltip directive is bound to a button, triggering the creation and removal of a tooltip element when the button is hovered over and the cursor moved away from it, respectively.
Example: The below example uses Vue Directives to create a tooltip with Vue.js.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 1</title>
<script src=
"https://cdn.jsdelivr.net/npm/vue@2">
</script>
<style>
.tooltip {
position: absolute;
background-color: black;
color: white;
padding: 5px;
border-radius: 3px;
z-index: 999;
}
</style>
</head>
<body>
<div id="app" style=
"text-align: center; margin-top: 50px;">
<h1>
Using Vue Directives
</h1>
<button v-tooltip="'Hello GFG'">
Hover Me
</button>
</div>
<script>
Vue.directive('tooltip', {
bind: function (el, binding) {
const tooltipText = binding.value;
el.addEventListener('mouseover',
function () {
const tooltip =
document.createElement('div');
tooltip.className = 'tooltip';
tooltip.textContent = tooltipText;
el.appendChild(tooltip);
});
el.addEventListener('mouseout',
function () {
const tooltip =
el.querySelector('.tooltip');
if (tooltip) {
el.removeChild(tooltip);
}
});
}
});
new Vue({
el: '#app'
});
</script>
</body>
</html>
Output:
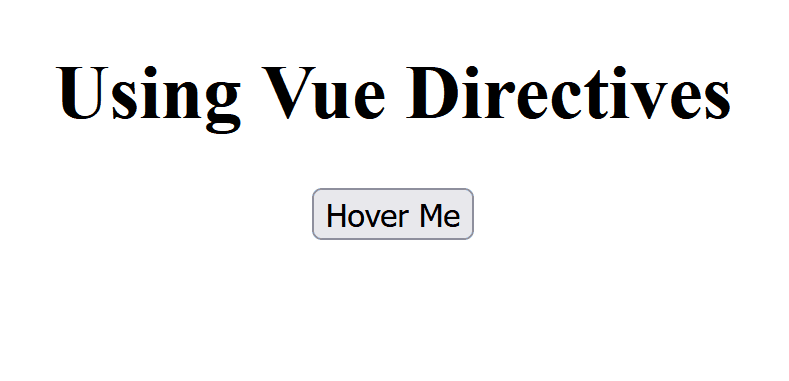
In this approach, we are using the Vue Tooltip Library (v-tooltip) to create a tooltip for a button. The v-tooltip directive is applied to the button, providing options such as content, delay, offset, and placement to customize the tooltip’s behavior and appearance.
Tooltip library CDN Link:
<script src="https://unpkg.com/v-tooltip@2"></script>
Example: The below example uses Vue Tooltip Library (v-tooltip) to create a tooltip with Vue.js.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Example 2</title>
<script src=
"https://cdn.jsdelivr.net/npm/vue@2">
</script>
<script src=
"https://unpkg.com/v-tooltip@2">
</script>
</head>
<body>
<div id="app" style=
"text-align: center;
margin-top: 50px;">
<h1>
Using Vue Tooltip Library
(v-tooltip)
</h1>
<button v-tooltip=
"{
content: 'Hello GFG',
delay: 200, offset: 10,
placement: 'bottom'
}">
Hover Me
</button>
</div>
<script>
Vue.use(VTooltip);
new Vue({
el: '#app'
});
</script>
</body>
</html>
Output:
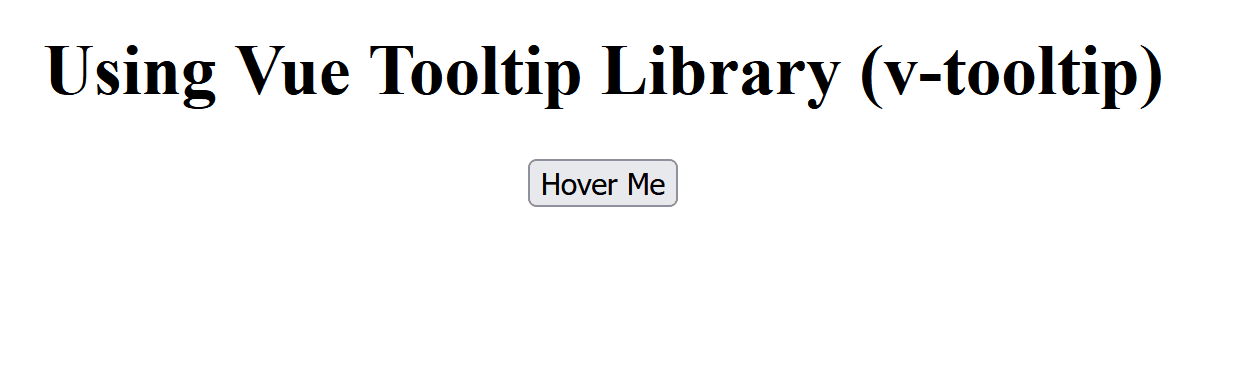
Share your thoughts in the comments
Please Login to comment...