How to Create Popup Box using HTML and CSS?
Last Updated :
26 Mar, 2024
Popup boxes are a valuable tool for improving user experience on websites. A Popup (or dialog box) is a modal window used to inform, warn, or receive input from the user. Pop-up windows should not be used as they prevent the user from accessing other aspects of the program until the pop-up window is closed.
In this create popup box in HTML and CSS article, we’ll cover the steps to build a simple popup using HTML and CSS. Whether you want to display additional information, collect user input, or showcase special offers.
Examples of Creating Popup Box using HTML and CSS
1. Using Display Property:
In this approach, we will show how to create a popup box or window using HTML and CSS. We use some basic HTML tags like heading, paragraph, button, and anchor tags. We create a button and when clicked, the popup box will open as you can see in the output. The anchor element is attached to the popup box with the “modal” class by using the href attribute that is used to specify a link to the “popup-box” address.
Example: Implementation of the popup box
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta
name="viewport"
content="width=device-width, initial-scale=1.0"
/>
<style>
.box {
background-color: black;
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
}
.box a {
padding: 15px;
background-color: #fff;
border-radius: 3px;
text-decoration: none;
}
.modal {
position: fixed;
inset: 0;
background: rgba(
254,
126,
126,
0.7
);
display: none;
align-items: center;
justify-content: center;
}
.content {
position: relative;
background: white;
padding: 1em 2em;
border-radius: 4px;
}
.modal:target {
display: flex;
}
</style>
</head>
<body>
<div class="box">
<a href="#popup-box"
>Click to Open Popup Box!</a
>
</div>
<div id="popup-box" class="modal">
<div class="content">
<h1 style="color: green">
Hello GeeksForGeeks!
</h1>
<p>Never Give Up!</p>
<a
href="#"
style="
position: absolute;
top: 10px;
right: 10px;
color: #fe0606;
font-size: 30px;
text-decoration: none;
"
>×</a
>
</div>
</div>
</body>
</html>
Output:
.gif)
Using Display Property
2. Using JavaScript EventListener:
In this example, we created an automatic popup window using HTML and CSS with the help of some markup tags like button, heading, anchor, and div element and then used a few lines of JavaScript code to create the dynamic popup window, we just add loading of the popup window in screen automatically. We use internal CSS for styling using the class names. The addEventListener() function in JavaScript takes the click event to listen for showing and closing the popup box with the id “myPopup”.
Example: In this example we are following above explained apporach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta
http-equiv="X-UA-Compatible"
content="IE=edge"
/>
<meta
name="viewport"
content="width=device-width,
initial-scale=1.0"
/>
<style>
.popup {
position: fixed;
z-index: 1;
left: 0;
top: 0;
width: 100%;
height: 100%;
overflow: auto;
background-color: rgba(
0,
0,
0,
0.4
);
display: none;
}
.popup-content {
background-color: white;
margin: 10% auto;
padding: 20px;
border: 1px solid #888888;
width: 30%;
font-weight: bolder;
}
.popup-content button {
display: block;
margin: 0 auto;
}
.show {
display: block;
}
</style>
</head>
<body>
<h3>
Create popup box using HTML and CSS
</h3>
<button id="myButton">Click me</button>
<div id="myPopup" class="popup">
<div class="popup-content">
<h1 style="color: green">
GeekforGeeks !
</h1>
<p>This is a popup box!</p>
<button id="closePopup">
Close
</button>
</div>
</div>
<script>
myButton.addEventListener(
"click",
function () {
myPopup.classList.add("show");
}
);
closePopup.addEventListener(
"click",
function () {
myPopup.classList.remove(
"show"
);
}
);
window.addEventListener(
"click",
function (event) {
if (event.target == myPopup) {
myPopup.classList.remove(
"show"
);
}
}
);
</script>
</body>
</html>
Output:
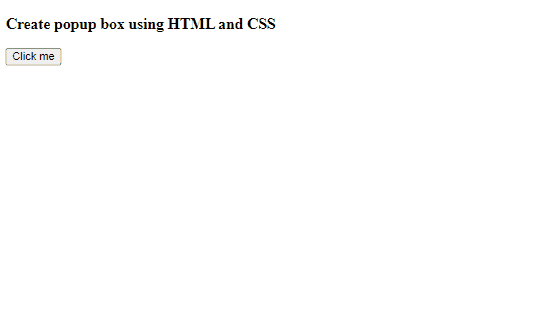
Using JavaScript EventListener Example Output
2. Using JavaScript SweetAlert Library:
Here we are creating popup using SweetAlert library. It is a JavaScript library that allows you to create beautiful and customizable popup boxes for displaying messages, alerts, confirmations, and prompts.
Example: In this example we are using SweetAlert library to create a popup.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta
name="viewport"
content="width=device-width, initial-scale=1.0"
/>
<title>Popup Box Example</title>
<scrit src=
"https://cdn.jsdelivr.net/npm/sweetalert2@11">
</scrit>
<style>
body {
text-align: center;
height: 100vh;
margin: 0;
}
</style>
</head>
<body>
<h3>Create popup box using SweetAlert</h3>
<button onclick="showPopup()">
Show Popup
</button>
<script>
function showPopup() {
Swal.fire({
title: "Welcome to GeeksForGeeks!",
text: "GeeksForGeeks is the best platform for Learning.",
showCloseButton: true,
});
}
</script>
</body>
</html>
Output:
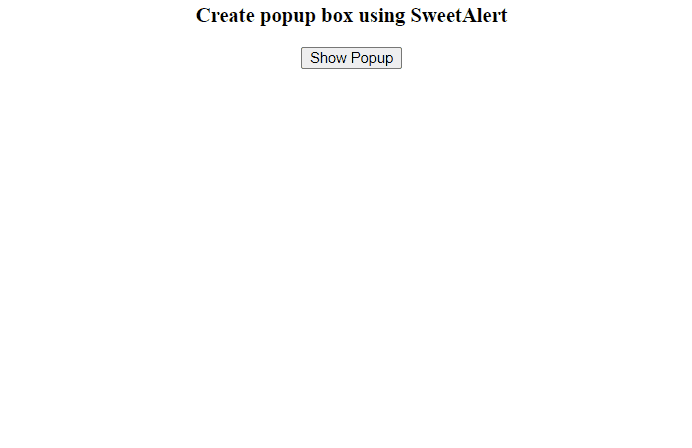
Share your thoughts in the comments
Please Login to comment...