How to create NPV Calculator Card using JavaScript & Tailwind CSS ?
Last Updated :
12 Mar, 2024
A Net Present Value (NPV) Calculator is a web-based tool that helps users calculate the net present value of an investment. It typically includes input fields for the initial investment amount, the discount rate, and the expected cash flows. The calculator then calculates the NPV based on these inputs. The calculator is styled using Tailwind CSS, a utility-first CSS framework, which ensures a responsive and visually appealing design.
Approach to Create NPV Calculator Card
- Start with the basic HTML structure, including the
<!DOCTYPE html>
declaration, <html>
, <head>
, and <body>
tags. Import Tailwind CSS for styling. - Create a container div with Tailwind CSS classes for styling. Inside the container, include elements for the app title, input fields for the initial investment, discount rate, and cash flows, buttons for calculating NPV and clearing inputs, and a div for displaying the result.
- Use JavaScript to handle the logic of calculating the NPV based on the initial investment, discount rate, and cash flows. Add event listeners to the calculate and clear buttons. When the calculate button is clicked, the script retrieves the values from the input fields, performs the calculation, and displays the result. When the clear button is clicked, the script clears the input fields and result.
- Use Tailwind CSS classes to style the app container, input fields, buttons, and result div. Customize the colors, fonts, and layout to create an appealing visual design for the NPV calculator.
- Ensure that the NPV calculator is responsive and works well on different screen sizes. Use Tailwind CSS classes for responsive design, such as
sm:
, md:
, and lg:
to adjust the layout and styling based on the screen size.
Example: Implementation to design NPV Calculator
HTML<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport"
content="width=device-width, initial-scale=1.0">
<title>NPV Calculator</title>
<script src="https://cdn.tailwindcss.com"></script>
</head>
<body class="bg-gray-100 flex justify-center
items-center h-screen">
<div class="border-4 border-green-400 bg-white
p-5 rounded-lg shadow-md w-full max-w-md">
<h1 class="text-3xl font-bold text-center text-green-600 mb-6">
NPV Calculator
</h1>
<div class="mb-4">
<label for="initialInvestment"
class="block text-lg font-semibold text-gray-700">
Initial Investment ($)
</label>
<input type="number" id="initialInvestment"
name="initialInvestment"
placeholder="Enter initial investment"
class="mt-1 block w-full px-4 py-2 border
border-gray-300 rounded-md focus:outline-none
focus:ring-2 focus:ring-blue-500
focus:border-transparent">
</div>
<div class="mb-4">
<label for="discountRate"
class="block text-lg font-semibold text-gray-700">
Discount Rate (%)
</label>
<input type="number" id="discountRate"
name="discountRate"
placeholder="Enter discount rate"
class="mt-1 block w-full px-4 py-2
border border-gray-300
rounded-md focus:outline-none focus:ring-2
focus:ring-blue-500 focus:border-transparent">
</div>
<div class="mb-4">
<label for="cashFlows"
class="block text-lg font-semibold text-gray-700">
Cash Flows
</label>
<textarea id="cashFlows" name="cashFlows" rows="3"
placeholder="Enter cash flows separated by commas"
class="mt-1 block w-full px-4 py-2 border
border-gray-300 rounded-md focus:outline-none
focus:ring-2 focus:ring-blue-500
focus:border-transparent">
</textarea>
</div>
<div class="mb-4 flex justify-center">
<button onclick="calculateNPV()"
class="px-6 py-3 bg-blue-500 text-white
rounded-md hover:bg-blue-600 focus:outline-none
focus:ring-2 focus:ring-blue-500
focus:ring-opacity-50">
Calculate
</button>
<button onclick="clearInputs()"
class="ml-4 px-6 py-3 bg-gray-300 text-gray-700
rounded-md hover:bg-gray-400 focus:outline-none
focus:ring-2 focus:ring-gray-300
focus:ring-opacity-50">
Clear
</button>
</div>
<div id="result"
class="text-xl font-semibold text-gray-800 text-center"></div>
</div>
<script>
function calculateNPV() {
const initialInvestment =
document.getElementById('initialInvestment').value.trim();
const discountRate =
document.getElementById('discountRate').value.trim();
const cashFlows =
document.getElementById('cashFlows').value.trim();
if (!initialInvestment || !discountRate || !cashFlows) {
alert('Please fill in all fields.');
return;
}
const initialInvestmentValue =
parseFloat(initialInvestment);
const discountRateValue =
parseFloat(discountRate);
const cashFlowsArray =
cashFlows.split(',').map(flow => parseFloat(flow.trim()));
let npv = -initialInvestmentValue;
for (let i = 0; i < cashFlowsArray.length; i++) {
npv += cashFlowsArray[i]/Math.pow(1 + discountRateValue / 100, i + 1);
}
document.getElementById('result').innerText =
`NPV: $${npv.toFixed(2)}`;
}
function clearInputs() {
document.getElementById('initialInvestment').value = '';
document.getElementById('discountRate').value = '';
document.getElementById('cashFlows').value = '';
document.getElementById('result').innerText = '';
}
</script>
</body>
</html>
Output:
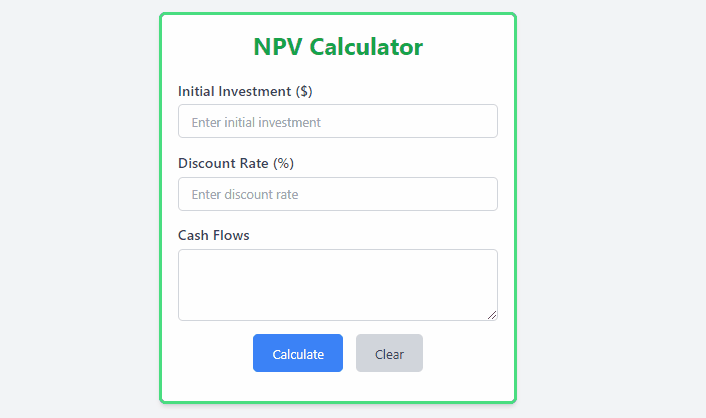
Share your thoughts in the comments
Please Login to comment...