How to create Calculator Card using Tailwind CSS & JavaScript ?
Last Updated :
12 Mar, 2024
In this article, we’ll create a Basic Calculator using the Tailwind CSS and JavaScript. The application allows users to input any numerical data and give the calculated result. We’ll utilize Tailwind CSS for styling to create an attractive user interface and JavaScript for the logic.
Approach to create Calculator Card in Tailwind & JavaScript
- Create an HTML file named index.html and link the Tailwind CSS stylesheet for styling.
- Create the structure of a Basic Calculator by using buttons for defining Numbers (0 – 9), Arithmetic operator (+, -, *, /, %), “.” for using decimal numbers and “=” operator for calculating the result.
- Create a file named script.js.
- Implement basic arithmetic operations like addition, subtraction, multiplication, division etc.
- Display the result in calculator’s display area.
Example: This example describes the implementation of a basic Calculator using Tailwind CSS and JavaScript.
HTML<!DOCTYPE html>
<html lang="en">
<head>
<title>Basic Calculator</title>
<link href=
"https://cdn.jsdelivr.net/npm/tailwindcss@2.2.19/dist/tailwind.min.css"
rel="stylesheet">
</head>
<body class="bg-gray-100 flex justify-center
items-center h-screen">
<div class="bg-white p-8 rounded-lg shadow-lg
border-2 border-green-500">
<h1 class="text-3xl font-bold
text-center mb-4">
Basic Calculator
</h1>
<!-- Display Section -->
<input type="text" id="result"
class="w-full bg-gray-200 text-right
p-4 mb-4 border border-gray-300
rounded-md focus:outline-none"
placeholder="0" readonly />
<!-- Input Section -->
<div class="grid grid-cols-4 gap-2">
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="clearResult()">
C
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('(')">
(
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter(')')">
)
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('/')">
/
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('7')">
7
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('8')">
8
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('9')">
9
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('*')">
*
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('4')">
4
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('5')">
5
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('6')">
6
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('-')">
-
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('1')">
1
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('2')">
2
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('3')">
3
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('+')">
+
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('0')">
0
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('.')">
.
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="calculateResult()">
=
</button>
<button class="bg-gray-300 hover:bg-gray-400
text-gray-800 font-bold py-2
px-4 rounded"
onclick="appendCharacter('%')">
%
</button>
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Javascript// file - script.js
// Clearing the display section
function clearResult() {
document.getElementById("result").
value = "";
}
// The user input
function appendCharacter(char) {
document.getElementById("result").
value += char;
}
// Performing the calculations
function calculateResult() {
let result =
document.getElementById("result").value;
try {
result = eval(result);
document.getElementById("result").
value = result;
} catch (error) {
document.getElementById("result").
value = "Error";
}
}
Output:
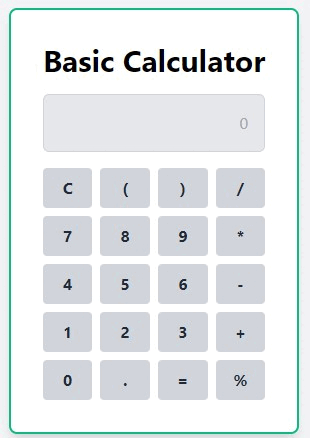
Share your thoughts in the comments
Please Login to comment...